ECE2323Spring2024_Lab5
pdf
keyboard_arrow_up
School
Northeastern University *
*We aren’t endorsed by this school
Course
2323
Subject
Electrical Engineering
Date
Apr 3, 2024
Type
Pages
5
Uploaded by AdmiralDoveMaster973
EECE2323
Digital Logic Design Lab
Lab 5
Adding Instruction Decoding to the Datapath
1
Objective and Overview
In this lab you will control the datapath using instructions from the lab computer. This lab mainly
includes two new components. First, you should write an
instruction decoder
that translates inputs
given to control and data signals for the datapath. Next, you will be given a debounce circuit to
integrate it into your design that connects the button
BTN1
on the TUL PYNQ board to the clock
so that you can single step your design.
You must complete Lab 4 before you start this experiment.
2
Decoding Instructions and Executing them on the Datapath
In this lab, you will add instruction decode logic to the datapath you completed in Lab 4. You should
implement an instruction decoder as described below. The machine code for your instructions will
be entered into your design using VIO. The inputs to the instruction decoder rs1 [2:0], rs2 [2:0],
rd[2:0], immediate [6:0], nzimm [5:0], offset [8:0] and opcode[3:0] will be provided by the VIO.
Instruction formats are described in
instruction_set.pdf
available on Canvas. Please note that
the instruction decoder is a combinational logic and a clock edge is only needed for writing to the
register file and writing to memory in the datapath. The second change is to add a debounce circuit
that allows you to single step your datapath. This circuit will connect the
BTN1
button on the
TUL PYNQ board to the clock inputs for memory and the register file. Figure 2 shows the top
level design for this lab experiment.
2.1
Prelab
2.1.1
Complete the instruction decoder table
Inputs
Outputs
operation
opcode
immediate
offset
nzimm
RegWrite
RegDst
instr
i
ALUSrc1
ALUSrc2
ALUOp
MemWrite
MemToReg
Regsrc
load word (lw)
4’b0000
value from vio
x
x
store word (sw)
4’b0001
value from vio
x
x
add
4’b0010
x
x
x
add immediate (addi)
4’b0011
x
x
value from vio
and
4’b0100
x
x
x
andi
4’b0101
value from vio
x
x
or
4’b0110
x
x
x
xor
4’b0111
x
x
x
sra immediate (srai)
4’b1000
x
x
value from vio
slli
4’b1001
x
x
value from vio
beqz
4’b1010
x
value from vio
x
bneqz
4’b1011
x
value from vio
x
Table 1: Prelab table
1
Figure 1: Instruction Decoder Interface
2.2
Entering Your Design
2.2.1
Instruction Decoder
Design an instruction decoder in SystemVerilog with the interface shown in Figure 1
.
Name your module
instruction_decoder
. Your circuit should be combinational and should take
in an instruction and generate the signals shown in the figure.
Note that there are two new signals
RegDst
and
Regsrc
that comes from the decoder. The
RegDst
signal chooses whether the register write address is the
rd
field or the
rs2
field.
The
Regsrc
chooses whether the register ReadAddr1 is the
rs1
field or the
rd
field. The top level module will
instantiate a mux based on this signal.
2.2.2
Simulate the instruction decoder
Before implementing the instruction decoder on FPGA, write a testbench to test your instruction
decode circuitry. The test bench should only test the instruction decoder circuit. Your
test vectors
should cover all possible types of instruction (by varying opcode, following the same order as shown
in Prelab Table 1).
Create a new testbench module called
inst
decoder
tb
.
Enter the test vectors that you have chosen into the testbench, save the testbench,
and run it in XSIM. Make sure that you set the
Simulation Run Time
property to an
appropriate value before running your simulation.
Save the waveform as part of the evidence that your lab works.
The TA will provide specific
instructions regarding what is expect to be handed in.
2
Figure 2: Datapath with Instruction Decoder Unit
2.2.3
Designing the top level
Get the top level SystemVerilog file,
pdatapath_top.v
from the course webpage. This Sys-
temVerilog code shows how all of your components are connected. You should have regfile, alu and
instruction decode components already designed. Get the debounce circuit,
debounce.v
from the
course webpage. You will need to generate data memory and VIO to add to this experiment. Note
that this VIO module has several new inputs and outputs. Refer to table
2
to see how the vio
probes are conneted to the inputs and outputs.
Add the constraint file for your design. The constraint file for this design is named:
pdatapath.xdc
2.3
Testing in Hardware
2.3.1
Implementing the Design with Vivado Synthesizer
Click on
Generate Bitstream
under the Program and Debug section in the Flow Nav-
igator pane to run the synthesis process.
Vivado IDE will run the Synthesis and
Implementation processes automatically.
3
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
VIO
Probes
Component
number of bits
probe
in0
regfile
WriteData
16
probe
in1
regfile
ReadData1
16
probe
in2
regfile
ReadData2
16
probe
in3
alu
input1
16
probe
in4
alu
input2
16
probe
in5
alu
take
branch
1
probe
in6
alu
ovf
flag
1
probe
in7
alu
output
16
probe
in8
DataMemOut
16
probe
in9
alu
input2
instr
src
16
probe
out0
rs1
3
probe
out1
rs2
3
probe
out2
rd
3
probe
out3
immediate
7
probe
out4
nzimm
6
probe
out5
offset
9
probe
out6
opcode
4
Table 2: VIO map
If the synthesis process runs correctly, continue by programming the FPGA board. Follow the same
steps you have for previous labs.
2.3.2
Programming the FPGA with Hardware Manager
Program your hardware the way you have in previous labs. Open the hardware man-
ager, open the target device, and program the PYNQ.
Now you should see the
hw_vio_1
window open. Click on the + sign and add all of the probes by
selecting and adding them. Now you are ready to test your design in hardware.
2.3.3
Testing Your Design
For this design, we are using one reset to reset the memory elements (memory and register file).
On the board, it is connected to the right push button (
BTN0
).
We have added the single step
button the push button (
BTN1
). You need to press
BTN1
whenever you want to store data in either
memory or the register file.
Use VIO to test your design. Please execute the operations in table 3. You will need
to complete this table and provide it in your report. Keep screen shots of the vio to
show that you have done this.
Follow instructions from your TA for what you need to submit or demo to show that
your lab works.
4
Inputs
Outputs
operation
opcode
immediate
offset
nzimm
rd
rs1
rs2
WriteData
ReadData1
ReadData2
input1
input2
alu
out
alu
ovf
take
branch
addi value of 5 with register 2
x
x
5
Let 1 clk cycle pass using BTN1
x
x
5
addi value of 3 with register 1
x
x
3
Let 1 clk cycle pass using BTN1
x
x
3
store value at register 1 to memory address 6
6
x
x
Let 1 clk cycle pass using BTN1
6
x
x
load value at memory address 6 to register 4
6
x
x
Let 1 clk cycle pass using BTN1
6
x
x
srai value at register 4 with immediate value of 1
x
x
1
Let 1 clk cycle pass using BTN1
x
x
1
xor value at register 2 with value at register 4
x
x
x
addi value of 5 with 2 register
x
x
5
beqz value at register address 3 with offset 0
x
0
x
bneqz value at register address 3 with offset 0
x
0
x
Table 3: completion table
5
Related Documents
Related Questions
Please help me to do this
arrow_forward
Here is the data flow diagram for a particular instruction. The various values shown indicate the state of the machine after the action of this
instruction. The question mark locates this instruction in memory.
GatePC
DR 100
GateMARMUX
RO
X2FFF
PC X
RI
x3006
MARMUX
TRAPVECTOR
R2
x3005
X0:0
PCMUX
R3
x3004
ZEXT
R4
x3003
OFPSETŐ OR
R5
x3002
PC OR BASER
R6
x3001
PCOFFSET
(7:0)
R7
x3000
ADDR2MUX
ADDRIMUX
SR2
SRI
(100
SEXT
IMMS
SEXT
SRZMUX
SEXT
FINITE
[50]
SEXT
STATE
[40]
MACHINE
Arith/Logic Operation
AL select
IRO01
A/L RESULT
LOGIC
GateALU
Gate MDR
16
16
MDR
MAR
T16
МЕМORY
x 30 0O
x 30 0 1
X 3:0:0 2
x3003
x3004
x 30 03
x3001
x: 3:0 0 4
X 30 05
X300 6
x3000
x3006
x3002
What is that instruction in binary? (You may use any number of spaces to organize the bits.)
address instruction
х3002
arrow_forward
I need to implement the 1-4 with 1-bit demultiplexer in a diagram. How to draw it. and how to do the final equation
arrow_forward
use the assembly language write a program in subject MicroController,
that Take input from P1 display it on P3 such that you have to take an initial value lets say 50 or 90(your choice) and increment it till 100 and display it till 100Take another input from P2 and display it on P3 such that you have to decrement it from 100 to the number you have initialized (50 or 90)
(Assembly language)
(MicroController)
arrow_forward
An 8085 assembly language program is given below.
Line 1: MVI A, B5H
2: MVI B, OEH
3: XRI 69H
4: ADD B
5: ANI 9BH
6: CPI 9FH
7: STA 3010H
8: HLT
The contents of the accumulator just execution of the ADD instruction in line
4 will be
arrow_forward
2-A) What's the name of the opamp circuit below? Briefly explain the logic.
2-B) Why is there a DC 1 volt offset in Vs sine signal?
arrow_forward
To select the ROM the address lines A10-A9 should be:
A. 00
B. 01
C. 10
D.10
arrow_forward
The numbers from 0-9 and a no characters
is the Basic 1 digit seven segment display
* .can show
False
True
In a (CA) method of 7 segments, the
anodes of all the LED segments are
* "connected to the logic "O
False
True
Some times may run out of pins on your
Arduino board and need to not extend it
* .with shift registers
True
False
arrow_forward
Here is the data flow diagram for a particular instruction. The various values shown indicate the state of the machine after the action of this
instruction.
GatePC
DR 101
GateMARMUX
RO
OnFFFO
OxFFFE
PC X
RI
MARMUX
TRAPVECTOR
R2
OxFFFD
X:0:0
PCMUX
R3
OFFFB
EXT
R4
ODFFA
OFFSET6 OR
R5
OXFFFD
PCOFPSET
PC OR BASER
R6
OcFFFC
(7:0)
R7
OscOFO8
ADDR2MUX
ADDRIMUX
SR2
SRI
010
[100]
SEXT
IMMS
(8:0)
SEXT
SEXT
FINITE
SRZMUX
[50]
SEXT
STATE
[4:0|
2
MACHINE
Arith/Logic Operation
AL select
A/L RESULT
LOGIC
GateALU
Gate MDR
16
MDR
MAR
16
OUTPUT
INPUT
MEMORY
XFE02
XFE06
What is that instruction in binary? (You may use any number of spaces to organize the bits.)
address instruction
x3002
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
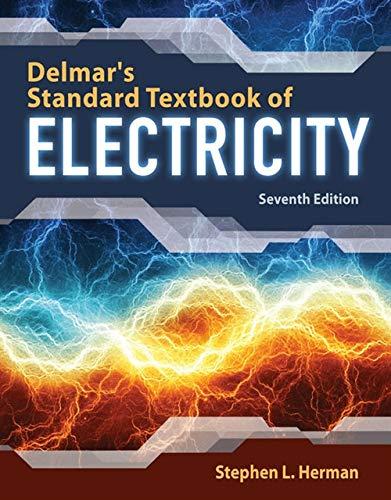
Delmar's Standard Textbook Of Electricity
Electrical Engineering
ISBN:9781337900348
Author:Stephen L. Herman
Publisher:Cengage Learning
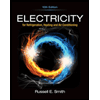
Electricity for Refrigeration, Heating, and Air C...
Mechanical Engineering
ISBN:9781337399128
Author:Russell E. Smith
Publisher:Cengage Learning
Related Questions
- Please help me to do thisarrow_forwardHere is the data flow diagram for a particular instruction. The various values shown indicate the state of the machine after the action of this instruction. The question mark locates this instruction in memory. GatePC DR 100 GateMARMUX RO X2FFF PC X RI x3006 MARMUX TRAPVECTOR R2 x3005 X0:0 PCMUX R3 x3004 ZEXT R4 x3003 OFPSETŐ OR R5 x3002 PC OR BASER R6 x3001 PCOFFSET (7:0) R7 x3000 ADDR2MUX ADDRIMUX SR2 SRI (100 SEXT IMMS SEXT SRZMUX SEXT FINITE [50] SEXT STATE [40] MACHINE Arith/Logic Operation AL select IRO01 A/L RESULT LOGIC GateALU Gate MDR 16 16 MDR MAR T16 МЕМORY x 30 0O x 30 0 1 X 3:0:0 2 x3003 x3004 x 30 03 x3001 x: 3:0 0 4 X 30 05 X300 6 x3000 x3006 x3002 What is that instruction in binary? (You may use any number of spaces to organize the bits.) address instruction х3002arrow_forwardI need to implement the 1-4 with 1-bit demultiplexer in a diagram. How to draw it. and how to do the final equationarrow_forward
- use the assembly language write a program in subject MicroController, that Take input from P1 display it on P3 such that you have to take an initial value lets say 50 or 90(your choice) and increment it till 100 and display it till 100Take another input from P2 and display it on P3 such that you have to decrement it from 100 to the number you have initialized (50 or 90) (Assembly language) (MicroController)arrow_forwardAn 8085 assembly language program is given below. Line 1: MVI A, B5H 2: MVI B, OEH 3: XRI 69H 4: ADD B 5: ANI 9BH 6: CPI 9FH 7: STA 3010H 8: HLT The contents of the accumulator just execution of the ADD instruction in line 4 will bearrow_forward2-A) What's the name of the opamp circuit below? Briefly explain the logic. 2-B) Why is there a DC 1 volt offset in Vs sine signal?arrow_forward
- To select the ROM the address lines A10-A9 should be: A. 00 B. 01 C. 10 D.10arrow_forwardThe numbers from 0-9 and a no characters is the Basic 1 digit seven segment display * .can show False True In a (CA) method of 7 segments, the anodes of all the LED segments are * "connected to the logic "O False True Some times may run out of pins on your Arduino board and need to not extend it * .with shift registers True Falsearrow_forwardHere is the data flow diagram for a particular instruction. The various values shown indicate the state of the machine after the action of this instruction. GatePC DR 101 GateMARMUX RO OnFFFO OxFFFE PC X RI MARMUX TRAPVECTOR R2 OxFFFD X:0:0 PCMUX R3 OFFFB EXT R4 ODFFA OFFSET6 OR R5 OXFFFD PCOFPSET PC OR BASER R6 OcFFFC (7:0) R7 OscOFO8 ADDR2MUX ADDRIMUX SR2 SRI 010 [100] SEXT IMMS (8:0) SEXT SEXT FINITE SRZMUX [50] SEXT STATE [4:0| 2 MACHINE Arith/Logic Operation AL select A/L RESULT LOGIC GateALU Gate MDR 16 MDR MAR 16 OUTPUT INPUT MEMORY XFE02 XFE06 What is that instruction in binary? (You may use any number of spaces to organize the bits.) address instruction x3002arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Delmar's Standard Textbook Of ElectricityElectrical EngineeringISBN:9781337900348Author:Stephen L. HermanPublisher:Cengage LearningElectricity for Refrigeration, Heating, and Air C...Mechanical EngineeringISBN:9781337399128Author:Russell E. SmithPublisher:Cengage Learning
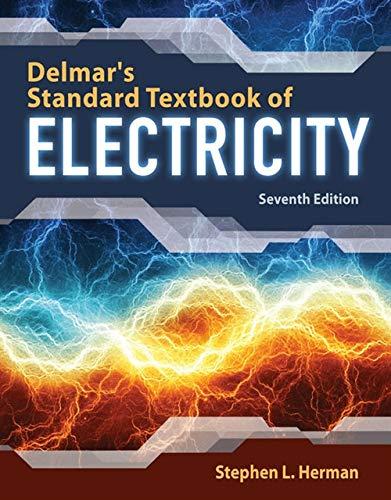
Delmar's Standard Textbook Of Electricity
Electrical Engineering
ISBN:9781337900348
Author:Stephen L. Herman
Publisher:Cengage Learning
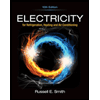
Electricity for Refrigeration, Heating, and Air C...
Mechanical Engineering
ISBN:9781337399128
Author:Russell E. Smith
Publisher:Cengage Learning