CSE_232_Exams-28
pdf
keyboard_arrow_up
School
Michigan State University *
*We aren’t endorsed by this school
Course
232
Subject
Computer Science
Date
Jan 9, 2024
Type
Pages
10
Uploaded by BailiffHawk7826
Exam 3
CSE 232 (Introduction to Programming II)
Fall 2023
ANSWERS VERSION 3
Full Name:
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
Student Number:
. . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . . .
Instructions:
•
DO NOT START/OPEN THE EXAM UNTIL TOLD TO DO SO.
•
You may however write and bubble in your name, student number and exam
VERSION/FORM
NUMBER
(with a #2 pencil) on the front of the printed exam and bubble sheet prior to the exam
start. This exam is Answers Version 3. Your section doesn’t matter and can be ignored.
•
Present your MSU ID (or other photo ID) when returning your bubble sheet and printed exam.
•
Only choose one option for each question.
•
Assume any needed
#include
s and
using std::...;
namespace declarations are performed for the
code samples.
•
Every question is worth the same amount of points. There are 55 questions, but you only need 50
questions correct for a perfect score.
•
No electronics are allowed to be used or worn during the exam. This means smart-watches, phones and
headphones need to be placed away in your bag.
•
The exam is open note, meaning that any paper material (notes, slides, prior exams, assignments, books,
etc.) are all allowed. Please place all such material on your desk prior to the start of the exam, (so you
won’t need to rummage in your bag during the exam).
•
If you have any questions during the exam or finish the exam early, please raise your hand and a proctor
will assist you.
http://xkcd.com/499/
Answers Version 3
Page 1 of 10
1. What advantage is there in dynamically al-
locating an array?
(a) Then the array can hold elements of
any size.
(b) Then the array can be resized with
the
std::resize
function.
(c) Then the size of the array doesn’t
need to be known at compile time.
(d) Then the array will not decay into a
pointer when passed as an argument.
(e) Two of (a-d) are correct.
(f) Three of (a-d) are correct.
Correct answers:
(c)
2. For a
vector
named
v
, if
v.front() ==
v.back()
, which of the following must be
true?
(a) v must have a capacity of 1.
(b) v’s begin and and end iterators must
also be equal.
(c) v must have a size of exactly 1.
(d) v must have invoked the
push
back
member function.
(e) v must be empty.
(f) None of the above.
Correct answers:
(f)
3. Why should you often make data members
private?
(a) Because data members have to be pri-
vate in a class.
(b) To
limit
outside
code’s
ability
to
break your class.
(c) Because getters and setters can only
work on private data members.
(d) Because friend functions can only ac-
cess private data members.
(e) None of the above.
Correct answers:
(b)
4. Which of the following are safe to do with
a null pointer?
(a) Make a copy of it.
(b) Store a memory address in it.
(c) Make a const reference to it.
(d) Dereference it.
(e) Only (a) and (b) are safe.
(f) Only (a), (b) and (c) are safe.
(g) All of (a), (b), (c), and (d) are safe.
Correct answers:
(f)
5. Assuming
x
is an array, the expression
x[7]++
is equivalent to which expression?
(a)
(*(x++ + 7))
(b)
*((x + 7)++)
(c)
(*x)++ + 7
(d)
(*(x + 7++))
(e)
(*(x + 7))++
(f) None of the above
Correct answers:
(e)
6. Assuming
vec
is a
vector<T>
, what is this
code doing?
copy(
vec.begin(),
vec.end(),
ostream
iterator<T>(x, ",")
);
(a) Adding a comma to each element of
the vector (except for the last)
(b) Writing the number of commas in a
string to a file.
(c) Writing to a stream named
x
(d) Converting the vector to a string
(e) Duplicating a vector
(f) None of the above
Correct answers:
(c)
Answers Version 3
Page 2 of 10
7. Which techniques are ways for a function to
communicate information?
(a) Returning a value
(b) Writing to standard output
(c) Throwing an exception
(d) Assigning to a dereferenced pointer
argument
(e) Changing a non-const reference argu-
ment
(f) All of the above
Correct answers:
(f)
8. Why
do
you
need
to
use
the
first
set
of parentheses in the following expression
(presuming
x
is a pointer to a
vector
)?
(*x).clear();
(a) Because they are needed to avoid a
syntax error.
(b) Because otherwise the behavior would
not be the same as
operator->
.
(c) Because the dot operator has a higher
precedence than the dereference oper-
ator.
(d) Because without them, the compiler
would think you are trying to call
clear
on a pointer.
(e) All of the above.
Correct answers:
(e)
9. What
is
the
primary
benefit
for
using
lambda functions?
(a) Because lambda functions can have
multiple parameters.
(b) Because lambda functions can access
private data members.
(c) To be able to define a single-use func-
tion right where it is needed.
(d) Because
many
STL
algorithms
re-
quire lambda functions to work.
(e) None of the above.
Correct answers:
(c)
10. What is the difference between
x
and
y
?
for (auto x : vec) ...
for (auto & y : vec) ...
(a)
x
is a copy of each element, where as
y
is a reference.
(b) If
y
is altered, the element in
vec
changes. Not so for
x
.
(c) Both of the above are true.
(d) None of the above are true.
Correct answers:
(c)
11. Which
git
command
is
needed
to
send
your
local
repo’s
changes/commits
to
a
remote
repo
(like
one
hosted
on
GitHub)?
(a)
git add
(b)
git pull
(c)
git push
(d)
git commit
(e) None of the above.
Correct answers:
(c)
12. Which of the following algorithms can work
with data stored in arrays?
(a)
std::transform
(b)
std::find
if
(c)
std::accumulate
(d)
std::remove
(e)
std::sort
(f)
std::copy
(g) All of the above.
Correct answers:
(g)
Answers Version 3
Page 3 of 10
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
13. What benefit is there to using a pointer in-
stead of a reference?
(a) A pointer can more safely refer to
an object because it can be declared
const.
(b) A pointer can be used in a friend func-
tion to access an objects private at-
tributes, whereas a reference can not.
(c) A pointer can be used to do bit-wise
arithmetic, where as a reference is
limited to integer arithmetic.
(d) A pointer can refer to two objects at
the same time because it can hold
multiple addresses, whereas a refer-
ence can only return to one object.
(e) A
pointer
can
point
to
objects
and
fundamental/primitive
types,
whereas a reference can only refer to
objects of custom classes.
(f) None of the above.
Correct answers:
(f)
14. What is does it mean if a logical operator
short-circuits?
(a) It means that the second operand
wont be evaluated.
(b) It means that the result returned is
true.
(c) It means that the logical operator has
no effect (no operation).
(d) It means that the logical operator is
undefined.
(e) It
means
that
the
operator
might
damage the hardware.
(f) None of the above
Correct answers:
(a)
15. Which of the following iterators support the
decrement operator?
(a) Bidirectional iterators
(b) Forward iterators
(c) Random access iterators
(d) (a) and (b)
(e) (b) and (c)
(f) (a) and (c)
(g) (a), (b) and (c)
(h) None of the above
Correct answers:
(f)
16. What does the following program output?
vector<int> vec
{
1, 2, 3
}
;
vec[vec.size()] = 0;
cout << vec.size();
(a)
3
(b) Unknown, because it has undefined
behavior.
(c)
4
(d) Unknown, because the size of the vec-
tor is unsigned.
(e) None of the above.
Correct answers:
(b)
17. What is the primary downside to using
#pragma once
instead of the traditional
header guards?
(a)
gcc
/
g++
doesnt support it.
(b) It only works if each header file has
its own name.
(c) It disables compiler warnings.
(d) It isnt part of the C++ standard.
(e) It interferes with the
gdb
debugger.
(f) It
doesn’t
work
for
function
tem-
plates.
(g) None of the above.
Correct answers:
(d)
Answers Version 3
Page 4 of 10
18. What
is
the
purpose
of
the
std::string::npos
identifier?
(a) It is the index one-past-the-end of a
C-style string.
(b) It indicates that an index isnt found
in the string.
(c) It is the value that is given to a string
method when you want to indicate
that negative positions/indices should
be used.
(d) It represents an empty string cant be
used with that function/method.
(e) It is an exception that is thrown when
invalid data is given to a string.
Correct answers:
(b)
19. What is the type of x?
int z = 7;
int * const y = &z;
auto x = y;
(a)
const int
(b)
auto
(c)
int *
(d)
const int *
(e)
int
(f)
int * const
(g) None of the above
Correct answers:
(c)
20. What key can you press to auto-complete
a partially typed filename at the command
line?
(a) Return
(b) Tab
(c) Control-Q
(d) Control-A
(e) Control-D
(f) None of the above.
Correct answers:
(b)
21. Which of the following statements are true,
assuming
v1
and
v2
are both non-empty
vector<int>
s?
std::copy(v1.begin(), v1.end(),
v2.begin());
std::copy(v1.begin(), v1.end(),
back
inserter(v2));
(a) The
first
statement
requires
that
v2.size() >= v1.size()
.
(b) The second statement enlarges
v2
as
needed.
(c) The first statement will replace exist-
ing elements of
v2
.
(d) The second statement appends each
element of
v1
to the end of
v2
in or-
der.
(e) Two of (a-d) are true.
(f) Three of (a-d) are true.
(g) Four of (a-d) are true.
Correct answers:
(g)
22. What advantage is there in returning a ref-
erence type (i.e.
string & func(string &
x);
) instead of a regular type (i.e.
string
func(string & x);
)?
(a) It allows the function to return a
pointer to a dynamically allocated ar-
ray instead of a staticly allocated one.
(b) They are identical to each other.
(c) It allows the return type to alter the
object that is created in the function.
(d) It avoids an unnecessary copy.
(e) None of the above.
Correct answers:
(d)
Answers Version 3
Page 5 of 10
23. Why should you never return the address
(a pointer) to a local variable?
(a) Because the local variable will be de-
stroyed when it goes out of scope (of-
ten when the function ends).
(b) Because
the
addresses
of
objects
change within functions depending on
if there are loops.
(c) Because doing so makes unnecessary
copies of the objects involved.
(d) Because it leaks memory.
(e) (a) and (b)
(f) (b) and (c)
(g) (c) and (d)
(h) (b) and (d)
Correct answers:
(a)
24. Which of the following exceptions will be
raised if allocated memory is not deleted?
(a)
std::logic
error
(b)
std::system
error
(c)
std::runtime
error
(d)
std::bad
alloc
(e) None of the above.
Correct answers:
(e)
25. What does the following code output?
map<string, string> name
to
city =
{{
"Josh", "EL"
}
,
{
"Emily", "CL"
}}
;
if (name
to
city["Mal"] == "DC")
cout << "In DC ";
cout << name
to
city.size();
(a)
3
(b) Undefined Behavior
(c)
2
(d) Compiler Error
(e)
In DC 2
Correct answers:
(a)
26. What property do pointers have that refer-
ences do not?
(a) Pointers can be made
const
so that
they can point at
const
objects.
(b) Pointers can be passed to a function
to avoid unneeded copies.
(c) Pointers can alter the object they
point at.
(d) Pointers can be changed to point at
different objects.
(e) None of the above.
Correct answers:
(d)
27. Why is proper indentation important for a
C++ source code?
(a) It doesn’t matter because whitespace
isn’t needed to have correct code.
(b) Because it improves readability for
humans reading the code.
(c) Because
indentation
is
needed
to
change
the
scope
(so
that
non-
dynamically allocated objects can be
destroyed).
(d) Because
properly
indented
code
is
easier for the compiler to optimize.
(e) Because spaces are better than tabs
because they allow for smaller file
sizes.
Correct answers:
(b)
28. A const member function has what prop-
erty that distinguishes it from a non-const
member function?
(a) It can be called on const objects.
(b) It is a getter member function.
(c) It returns a const object.
(d) It has only const parameters.
(e) It returns a reference to a const ob-
ject.
(f) All of the above.
Correct answers:
(a)
Answers Version 3
Page 6 of 10
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
29. What happens if an exception is thrown in
a program, but it is never caught?
(a) It generates undefined behavior.
(b) It causes a runtime error.
(c) It causes a compile time error, but
only if a debugger is being used.
(d) It causes a compile time error, but
only if a debugger is NOT being used.
(e) The main function will catch it and
prompt the user for direction.
(f) None of the above.
Correct answers:
(b)
30. Which of the following expressions have
type
int
, assuming
vec
is a 2-dimensional
table with 3 rows and 2 columns and has
type
vector<vector<int>>
.
(a)
vec.size()
(b)
vec[1].size()
(c)
vec[1][0]
(d)
vec[0]
(e) Two of (a-d) have type
int
.
(f) Three of (a-d) have type
int
.
(g) Four of (a-d) have type
int
.
Correct answers:
(c)
31. Why should you often mark converting con-
structors as
explicit
?
(a) Because converting constructors must
be marked explicit by the language
standard.
(b) To avoid unintended implicit casts
from your class to other types.
(c) To avoid unintended implicit casts to
your class.
(d) All of the above.
Correct answers:
(c)
32. How many times will
func
be invoked?
vector<int> v =
{
1, 2, 3
}
;
for (
auto i = v.size() - 1;
i >= 0;
--i)
{
func(v);
}
(a) Impossible to say as the code can’t
compile.
(b) More than 3
(c) 3
(d) 2
(e) 1
(f) 0
Correct answers:
(b)
33. How do you stop fall-through behavior?
(a) By using a conditional statement to
check for const expressions.
(b) By specifying a zero case for your con-
ditional expression.
(c) By ensuring that you have a break at
the end of each case.
(d) You cant stop fall-through, it is man-
dated by the C++ language.
(e) By ensuring that you have a default
case.
(f) None of the above
Correct answers:
(c)
34. What does the
const
in the following dec-
laration imply about
x
?
long * const x = &y;
(a) That the value of x (the address) can-
not be changed to point at a different
position in memory.
(b) That the value pointed at by x (the
long) cannot be changed to be a dif-
ferent value.
(c) Both of the above.
(d) Neither of the above.
Correct answers:
(a)
Answers Version 3
Page 7 of 10
35. In the copy-swap idiom, how was the copy
accomplished?
(a) By making the
operator=
take its ar-
gument by value.
(b) By enforcing the Rule of Three
(c) By copying the code from a previous
member function.
(d) By using the
std::copy
function.
(e) None of the above
Correct answers:
(a)
36. If the following line of code is legal, which
of the following are possible types for
x
?
auto y = x->begin();
(a)
vector<string> * x;
(b)
map<int, double> * x;
(c)
vector<int, double> x;
(d)
map<int, double> x;
(e) (a) and (b)
(f) (c) and (d)
(g) (a) and (c)
(h) None of the above.
Correct answers:
(e)
37. If a function is said to be overloaded, that
means that there are two functions that
share what property?
(a) The same header file
(b) The same body (using templates)
(c) The same libraries
(d) The same return type
(e) The same name
(f) The same arguments
(g) The same parameters
Correct answers:
(e)
38. Which of the following is FALSE regarding
std::map
?
(a) A map can have duplicate keys.
(b) A map can have a pointer to it.
(c) A map can be const.
(d) A map can have char values.
(e) A maps value type can be another
map.
(f) A map can have iterators to it.
Correct answers:
(a)
39. When are automatic variables (variables
that
arent
dynamically
allocated)
de-
stroyed?
(a) When their copy constructor is called.
(b) When they are deleted.
(c) When they are no longer needed.
(d) When they fall out of scope.
(e) When are passed to a function.
(f) When the loop iteration ends.
Correct answers:
(d)
40. What is the primary benefit to writing tem-
plated functions?
(a) So that the function compiles faster.
(b) So that the function is composited.
(c) So that the function is generic.
(d) So that the function is throws excep-
tions.
(e) So that the function runs faster.
(f) None of the above.
Correct answers:
(c)
41. Why should you declare a parameter
const
even though the compiler doesnt require it?
(a) To enforce runtime assertions.
(b) To avoid unnecessary copies.
(c) To allow the code to compile faster.
(d) To allow the function to be used in a
debugger.
(e) To allow the compiler to enforce a
guarantee made by the developer.
(f) All of the above
Correct answers:
(e)
Answers Version 3
Page 8 of 10
42. For a class named
Thing
, what is the type
of the implicit
this
?
(a)
const Thing * const
(b)
Thing
(c)
Thing *
(d)
Thing * const
(e) Depends on if the member function is
const
.
Correct answers:
(e)
43. What is the primary situation (at least in
this course) when the Rule of Three func-
tions should be implemented?
(a) When the class has dynamically allo-
cated memory.
(b) When the class has a default con-
structor.
(c) When the class has private data mem-
bers.
(d) (a) and (b)
(e) (a) and (c)
(f) (b) and (c)
(g) All of the above.
(h) None of the above.
Correct answers:
(a)
44. What is the name of the operation to com-
bine two different git branches into one?
(a) root
(b) concatenate
(c) stage
(d) squash
(e) commit
(f) merge
(g) branch
(h) fork
Correct answers:
(f)
45. Which of the following types is guaranteed
to be strictly the largest in size?
(a)
char
(b)
unsigned int
(c)
int
(d)
long
(e)
long long
(f) None of the above
Correct answers:
(f)
46. What property must the containers be-
ing
used
as
input
for
a
set
algo-
rithm
(like
std::set
difference
)
sat-
isfy?
(a) They must be non-empty
(b) They must be sorted
(c) They must not have duplicates
(d) They must be sets
(e) None of the above
Correct answers:
(b)
47. When you use a range-based for loop on a
map<string, int>
, what type is each ele-
ment?
(a) You can’t use such a loop on a map.
(b)
map<string, int>
(c)
string
(d)
int
(e)
pair<const string, int>
Correct answers:
(e)
48. Which of the following options allow you
to write output from your program to a
file?
(a) Command line IO redirection
(b) Using the PATH environment vari-
able
(c)
std::ofstream
(d) Wildcards
(e) All of the above
(f) (a) and (b)
(g) (a) and (c)
(h) (b) and (c)
(i) (a) and (b) and (c)
Correct answers:
(g)
Answers Version 3
Page 9 of 10
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
49. What is the purpose of header guards?
(a) To allow templates to be instantiated.
(b) To allow for faster compilation.
(c) To
avoid
redeclaration/redefinition
errors.
(d) To ensure that a class’s privacy is
maintained.
(e) None of the above
Correct answers:
(c)
50. Which of the following member functions
should usually be marked
const
?
(a) Getters
(b) Setters
(c) Copy Constructors
(d) Converting Constructors
(e) Destructors
(f) All of the above.
(g) None of the above.
Correct answers:
(a)
51. Which of the following are
NOT
legal, in-
teger literals?
(a)
098
(b)
0
(c)
125
(d)
-56
(e)
int x;
(f) All of the above are legal.
(g) Both (d) and (e) are not legal.
(h) Both (a) and (e) are not legal.
Correct answers:
(h)
52. Why should you generally use STL algo-
rithms instead of implementing your own
with loops?
(a) STL
algorithms
are
often
more
generic.
(b) STL algorithms are often more read-
able.
(c) STL algorithms are often faster.
(d) STL
algorithms
are
often
better
tested for correctness.
(e) All of the above.
Correct answers:
(e)
53. Which of the following can you do with a
pointer from a dynamically allocated de-
cayed array?
(a) Delete the array.
(b) Make the array const.
(c) Change the type of the array.
(d) Change the size of an array.
(e) None of the above.
Correct answers:
(a)
54. What happens if code in a
try
raises an
exception that isn’t the one a
catch
block
specifies?
(a) The
try
block finishes, then the ex-
ception is raised again.
(b) The
try
block ceases executing at the
point of the raised exception.
(c) The
catch
block executes, but the
same exception is raised at the end.
(d) The
what
member function on the ex-
ception is called.
(e) None of the above.
Correct answers:
(b)
55. Which of the following operations are legal
(i.e. will not cause an error or undefined be-
havior)?
vector<int> x
{
1, 2, 3
}
;
auto y = x.cend();
(a)
y[0];
(b)
*y;
(c)
--y;
(d)
y = 5;
(e) None of the above.
Correct answers:
(c)
Answers Version 3
Page 10 of 10
Related Documents
Recommended textbooks for you
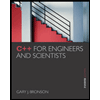
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
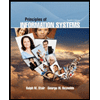
Principles of Information Systems (MindTap Course...
Computer Science
ISBN:9781285867168
Author:Ralph Stair, George Reynolds
Publisher:Cengage Learning
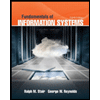
Fundamentals of Information Systems
Computer Science
ISBN:9781305082168
Author:Ralph Stair, George Reynolds
Publisher:Cengage Learning
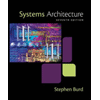
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning
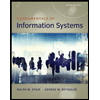
Fundamentals of Information Systems
Computer Science
ISBN:9781337097536
Author:Ralph Stair, George Reynolds
Publisher:Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
Recommended textbooks for you
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrPrinciples of Information Systems (MindTap Course...Computer ScienceISBN:9781285867168Author:Ralph Stair, George ReynoldsPublisher:Cengage LearningFundamentals of Information SystemsComputer ScienceISBN:9781305082168Author:Ralph Stair, George ReynoldsPublisher:Cengage Learning
- Systems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage LearningFundamentals of Information SystemsComputer ScienceISBN:9781337097536Author:Ralph Stair, George ReynoldsPublisher:Cengage LearningProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
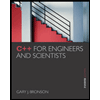
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
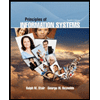
Principles of Information Systems (MindTap Course...
Computer Science
ISBN:9781285867168
Author:Ralph Stair, George Reynolds
Publisher:Cengage Learning
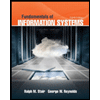
Fundamentals of Information Systems
Computer Science
ISBN:9781305082168
Author:Ralph Stair, George Reynolds
Publisher:Cengage Learning
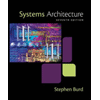
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning
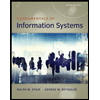
Fundamentals of Information Systems
Computer Science
ISBN:9781337097536
Author:Ralph Stair, George Reynolds
Publisher:Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage