gatorLibrary
py
keyboard_arrow_up
School
University of Florida *
*We aren’t endorsed by this school
Course
6933
Subject
Computer Science
Date
Dec 6, 2023
Type
py
Pages
6
Uploaded by JudgeTank12761
# get the red black and min heap from their files.
from red_black_tree import RedBlackTree
from min_heap import MinHeap, MinHeapNode
import time
import sys
#Create main class GatorLibrary class GatorLibrary:
#initialize the red_black tree for the books we are about to store.
def __init__(self):
self.books = RedBlackTree() # Our first function is going to be the PrintBook
def PrintBook(self, book_id):
book_id=int(book_id)
#Define the node, in red black tree
node = self.books.search(book_id)
# check if it exists
if node:
# Print the information as stored
print(f"BookID = {node.key}")
print(f"Title = '{node.value['title']}'")
print(f"Author = '{node.value['author']}'")
print(f"Availability = '{node.value['availability']}'")
print(f"BorrowedBy = {node.value['borrowed_by']}")
reservations = node.value['reservations']
# Since we have multiple values in reservation, i only want to print the patron id, i seperate and print
if reservations:
reservations_formatted = '[' + ', '.join(str(res.PatronID) if isinstance(res, MinHeapNode) else str(res['patron_id']) for res in reservations) + ']'
print(f"Reservations: {reservations_formatted}\n")
else:
print("Reservations: []\n")
# If not found, print this
else:
print(f"Book {book_id} not found in the Library\n")
#This is our second function, which gets all the book info printed within that range
def PrintBooks(self, book_id1, book_id2):
book_id1=int(book_id1)
book_id2=int(book_id2)
start_id = min(book_id1, book_id2)
end_id = max(book_id1, book_id2)
# Do a for loop and define node and keep priniting all that exists.
for book_id in range(start_id, end_id + 1):
node = self.books.search(book_id)
if node:
self.PrintBook(book_id)
# This is our third function, which tells us to InsertBook with their given values.
def InsertBook(self, book_id, book_name, author_name, availability_status, borrowed_by=None, reservation_heap=None):
# Check if the book already exists
book_id=int(book_id)
existing_book = self.books.search(book_id)
# This i have added since some values were not getting stripped properly in
the file reading part.
availability_status = availability_status.strip('")') #If it exists, print this
if existing_book:
print(f"Book with ID {book_id} already exists in the library.\n")
return
# Otherwise create a new book and insert values.
new_book = {
'title': book_name,
'author': author_name,
'availability': availability_status,
'borrowed_by': borrowed_by,
'reservations': reservation_heap if reservation_heap else []
}
# Do the main insert function linked to RedBlack
self.books.insert(book_id, new_book)
# This is our 4th function which is the BorrowBook, here we assign the borrowed
by and other staus changes and checks
def BorrowBook(self, patron_id, book_id, patron_priority):
# Search for the book requested
book_id=int(book_id)
book_node = self.books.search(book_id)
#If it doesnt exists print this if not book_node:
print(f"Book with ID {book_id} not found in the library.\n")
return
# else get the availibility of that book
if book_node.value['availability'] == "Yes":
# Book is available, update book status and borrower information
book_node.value['availability'] = "No"
book_node.value['borrowed_by'] = patron_id
print(f"Book {book_id} Borrowed by Patron {patron_id}\n")
else:
# Book is unavailable, add reservation to the book's reservation heap
reservations = book_node.value['reservations']
# 20 is limit given to us
if len(reservations) >= 20:
print(f"Reservation limit reached for Book {book_id}. Cannot add more reservations.\n")
return
# Add the new reservation
new_reservation = {
'patron_id': patron_id,
'priority_number': patron_priority,
# Get the timestamp using time package for priorotizing
'time_of_reservation': time.time() }
reservations.append(new_reservation)
# Update the reservations for the book
book_node.value['reservations'] = reservations print(f"Book {book_id} Reserved by Patron {patron_id}\n")
# This is our 5th function, which tracks the return of books and reassigning
def ReturnBook(self, patron_id, book_id):
# Check for book
book_id=int(book_id)
book_node = self.books.search(book_id)
# If it doesnt exists print this if not book_node:
print(f"Book with ID {book_id} not found in the library.\n")
return
# If it does and availability is yes, then print this
if book_node.value['availability'] == "Yes":
print(f"Book {book_id} is already available.\n")
return
# Book is borrowed, update book status and borrower information
book_node.value['availability'] = "Yes"
borrowed_by = book_node.value['borrowed_by']
book_node.value['borrowed_by'] = None
print(f"Book {book_id} Returned by Patron {patron_id}\n")
# Check if there are reservations for the returned book
reservations = book_node.value['reservations']
# Now since the book is returned, we give it to the next person on our list, who requested the book, to check that
if reservations:
# Get the min heap, where reservations is stored and keep inserting to generate one.
min_heap = MinHeap()
for reservation in reservations:
if isinstance(reservation, MinHeapNode):
min_heap.insert(reservation.PatronID, reservation.PriorityNumber, reservation.TimeOfReservation)
else:
min_heap.insert(reservation['patron_id'], reservation['priority_number'], reservation['time_of_reservation'])
# Assign the book to the patron with the highest priority
highest_priority_patron = min_heap.extract_min()
assigned_patron_id = highest_priority_patron.PatronID
print(f"Book {book_id} Allotted to Patron {assigned_patron_id}\n")
# Update book's status and borrower information
book_node.value['availability'] = "No"
book_node.value['borrowed_by'] = assigned_patron_id
# Remove the assigned patron from the reservation heap
book_node.value['reservations'] = min_heap.heap
# This is our 6th function, where we delete the book
def DeleteBook(self, book_id):
# Check if it exists
book_id=int(book_id)
book_node = self.books.search(book_id)
#Pri nt this it it doesnt
if not book_node:
print(f"Book with ID {book_id} not found in the library.\n")
return
# Get book details before deletion for notification
book_title = book_node.value['title']
reservations = book_node.value['reservations']
# Delete the book from the library
self.books.delete(book_id)
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
# Since the book is deleted, all reservations will be cancelled and removed.
if reservations:
reserved_patrons = ', '.join(str(res.PatronID) if isinstance(res, MinHeapNode) else str(res['patron_id']) for res in reservations)
if len(reservations) > 1:
print(f"Book {book_id} is no longer available. Reservations made by
Patrons {reserved_patrons} have been cancelled!\n")
else:
print(f"Book {book_id} is no longer available. Reservation made by Patron {reserved_patrons} has been cancelled!\n")
else:
print(f"Book {book_id} is no longer available.\n")
# This is our 7th function and this is to find the left and right childerns for
a book
def FindClosestBook(self, target_id):
target_id = int(target_id) # Get the lower and higher nodes, using function defined in redblack.
closest_lower = self.books.find_closest_lower(target_id)
closest_higher = self.books.find_closest_higher(target_id)
#if they exists keep printing them
if closest_lower and closest_higher:
lower_key = int(closest_lower.key) higher_key = int(closest_higher.key) # Calculate the difference between target_id and the closest book IDs
lower_diff = target_id - lower_key
higher_diff = higher_key - target_id
# Get the differnts, if lower and higher equal, print all, This is how in red black we know its balanced and all nodes under are printed
if lower_diff == higher_diff:
# If both differences are equal, print both books ordered by bookIDs
self.PrintBook(closest_lower.key)
self.PrintBook(closest_higher.key)
elif lower_diff < higher_diff:
# Print details of the closest lower book
self.PrintBook(closest_lower.key)
else:
# Print details of the closest higher book
self.PrintBook(closest_higher.key)
elif closest_lower:
# Print details of the closest lower book if only lower exists
self.PrintBook(closest_lower.key)
elif closest_higher:
# Print details of the closest higher book if only higher exists
self.PrintBook(closest_higher.key)
# Check if the target ID matches a book ID exactly
exact_match = self.books.search(target_id)
if exact_match:
self.PrintBook(exact_match.key)
# This is the 7th function and this prints the colour flip values
def ColorFlipCount(self):
print('Colour Flip Count: '+str( self.books.get_color_flip_count())+'\n')
# This is the 8th function, which terminates the program.
def Quit(self):
print("Program Terminated!!")
sys.exit()
# This is an extra function, i created to clean and get proper values from our input text data and process them through functions
def process_commands_from_file(self, file_path):
# open the input file
with open(file_path, 'r') as file:
commands = file.readlines()
# get each line and run through loop
for line in commands:
# strip it of ( and the first part of the line is the main command.
parts = line.strip().split('(')
command = parts[0]
# then we slip the rest of the values seperated by , and the spaces.
args = parts[1][:-1].split(',') args = [arg.strip(' ') for arg in args] # Removing the closing bracket and splitting arguments
# Remove quotes from arguments
args = [arg.strip('\"') for arg in args]
# This is how we check the main command and pass the rest as arguments.
if command == 'InsertBook':
self.InsertBook(*args)
elif command == 'PrintBook':
self.PrintBook(*args)
elif command == 'PrintBooks':
self.PrintBooks(*args)
elif command == 'BorrowBook':
self.BorrowBook(*args)
elif command == 'ReturnBook':
self.ReturnBook(*args)
elif command == 'DeleteBook':
self.DeleteBook(*args)
elif command == 'FindClosestBook':
self.FindClosestBook(*args)
elif command == 'ColorFlipCount':
self.ColorFlipCount()
elif command == 'Quit':
self.Quit()
# This is the main function that runs
if __name__ == "__main__":
# Define a gator library function
library = GatorLibrary()
# get the first argument passed as the text file input
a=sys.argv[1]
input_path = a+'.txt'
output_file_path = input_path.replace('.txt', '_output_file.txt') # Create a new output file path
# Redirect output to a file
with open(output_file_path, 'w') as output_file:
sys.stdout = output_file # Redirect standard output to the file
library.process_commands_from_file(input_path)
# Reset standard output to console
sys.stdout = sys.__stdout__
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Recommended textbooks for you
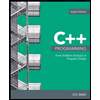
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
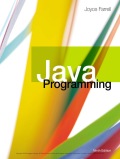
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
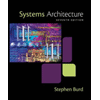
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage Learning
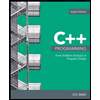
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
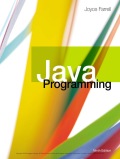
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
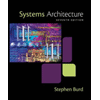
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning