2-more_exercises
pdf
keyboard_arrow_up
School
University of Colorado, Denver *
*We aren’t endorsed by this school
Course
6040
Subject
Computer Science
Date
Feb 20, 2024
Type
Pages
10
Uploaded by hhfehiiue
2/17/24, 7:37 AM
2-more_exercises
https://inst-fs-iad-prod.inscloudgate.net/files/63a3952e-76eb-48fe-8842-92f453f2a0e1/nb1.2 solutions.html?token=eyJ0eXAiOiJKV1QiLCJhbGciOiJIUzUxMiJ9.e…
1/10
Python review: More exercises
This notebook continues the review of Python basics. A key concept is that of a nested
data structure. For
example, the first code cell will define a 2-D "array" as a list of lists.
In [1]:
%
load_ext
autoreload
%
autoreload
2
Consider the following dataset of exam grades, organized as a 2-D table and stored in Python as a "list of lists"
under the variable name, grades
.
In [2]:
grades = [
# First line is descriptive header. Subsequent lines hold data
['Student', 'Exam 1', 'Exam 2', 'Exam 3'],
['Thorny', '100', '90', '80'],
['Mac', '88', '99', '111'],
['Farva', '45', '56', '67'],
['Rabbit', '59', '61', '67'],
['Ursula', '73', '79', '83'],
['Foster', '89', '97', '101']
]
grades
Exercise 0
(
students_test
: 1 point). Complete the function get_students
which takes a nested list
grades
as a parameter and reutrns a new list, students
, which holds the names of the students as they from
"top to bottom" in the table.
Note
: the parameter grades
will be similar to the table above in structure, but the data will be
different.
In [3]:
def
get_students(grades):
###
### YOUR CODE HERE
###
return
[L[0] for
L in
grades[1:]]
Out[2]:
[['Student', 'Exam 1', 'Exam 2', 'Exam 3'],
['Thorny', '100', '90', '80'],
['Mac', '88', '99', '111'],
['Farva', '45', '56', '67'],
['Rabbit', '59', '61', '67'],
['Ursula', '73', '79', '83'],
['Foster', '89', '97', '101']]
2/17/24, 7:37 AM
2-more_exercises
https://inst-fs-iad-prod.inscloudgate.net/files/63a3952e-76eb-48fe-8842-92f453f2a0e1/nb1.2 solutions.html?token=eyJ0eXAiOiJKV1QiLCJhbGciOiJIUzUxMiJ9.e…
2/10
The demo cell below should display ['Thorny', 'Mac', 'Farva', 'Rabbit', 'Ursula', 'Foster']
.
In [4]:
students = get_students(grades)
students
The test cell below will check your solution against several randomly generated test cases. If your solution does
not pass the test (or if you're just curious), you can look at the variables used in the latest test run. They are
automatically imported for you as part of the test.
input_vars
- Dictionary containing all of the inputs to your function. Keys are the parameter names.
original_input_vars
- Dictionary containing a copy of all the inputs to your function. This is useful
for debugging failures related to your solution modifying the input. Keys are the parameter names.
returned_output_vars
- Dictionary containing the outputs your function generated. If there are
multiple outputs, the keys will match the names mentioned in the exercrise instructions.
true_output_vars
- Dictionary containing the outputs your function should have
generated. If there
are multiple outputs, the keys will match the names mentioned in the exercrise instructions.
All of the test cells in this notebook will use the same format, and you can expect a similar format on your
exams as well.
In [5]:
# `students_test`: Test cell
import
nb_1_2_tester
tester = nb_1_2_tester.Tester_1_2_0()
for
_ in
range(20):
try
:
tester.run_test(get_students)
(input_vars, original_input_vars, returned_output_vars, true_o
utput_vars) = tester.get_test_vars()
except
:
(input_vars, original_input_vars, returned_output_vars, true_o
utput_vars) = tester.get_test_vars()
raise
print('Passed. Please submit!')
In [6]:
input_vars
Out[4]:
['Thorny', 'Mac', 'Farva', 'Rabbit', 'Ursula', 'Foster']
Passed. Please submit!
Out[6]:
{'grades': [['Student', 'Exam 1', 'Exam 2', 'Exam 3'],
['Jabba Desilijic Tiure', '50', '93', '93'],
['Nute Gunray', '72', '98', '63'],
['Lando Calrissian', '54', '76', '68'],
['Owen Lars', '84', '93', '101'],
['Shaak Ti', '67', '93', '67'],
['Luminara Unduli', '70', '99', '65'],
['Chewbacca', '74', '97', '57']]}
2/17/24, 7:37 AM
2-more_exercises
https://inst-fs-iad-prod.inscloudgate.net/files/63a3952e-76eb-48fe-8842-92f453f2a0e1/nb1.2 solutions.html?token=eyJ0eXAiOiJKV1QiLCJhbGciOiJIUzUxMiJ9.e…
3/10
Exercise 1
(
assignments_test
: 1 point). Complete the function get_assignments
. The function takes
grades
(a nested list structured similarly to grades
above) as a parameter. It should return a new list
assignments
which holds the names of the class assignments. (These appear in the descriptive header
element of grades
.)
In [7]:
def
get_assignments(grades):
###
### YOUR CODE HERE
###
return
grades[0][1:]
The demo cell below should display ['Exam 1', 'Exam 2', 'Exam 3']
In [8]:
assignments = get_assignments(grades)
assignments
The following test cell will check your function against several randomly generated test cases.
In [9]:
import
nb_1_2_tester
tester = nb_1_2_tester.Tester_1_2_1()
for
_ in
range(20):
try
:
tester.run_test(get_assignments)
(input_vars, original_input_vars, returned_output_vars, true_o
utput_vars) = tester.get_test_vars()
except
:
(input_vars, original_input_vars, returned_output_vars, true_o
utput_vars) = tester.get_test_vars()
raise
print('Passed. Please submit!')
Exercise 2
(
grade_lists_test
: 1 point). Complete the function for build_grade_lists
, again taking
grades
as a parameter. The function should return a new dictionary
, named grade_lists
, that maps names
of students to lists
of their exam grades. The grades should be converted from strings to integers. For instance,
grade_lists['Thorny'] == [100, 90, 80]
.
Out[8]:
['Exam 1', 'Exam 2', 'Exam 3']
Passed. Please submit!
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
2/17/24, 7:37 AM
2-more_exercises
https://inst-fs-iad-prod.inscloudgate.net/files/63a3952e-76eb-48fe-8842-92f453f2a0e1/nb1.2 solutions.html?token=eyJ0eXAiOiJKV1QiLCJhbGciOiJIUzUxMiJ9.e…
4/10
In [10]:
# Create a dict mapping names to lists of grades.
def
build_grade_lists(grades):
###
### YOUR CODE HERE
###
grade_lists = {} # Empty dictionary
for
L in
grades[1:]:
grade_lists[L[0]] = [int(g) for
g in
L[1:]]
return
grade_lists
The demo cell below should display
{'Thorny': [100, 90, 80],
'Mac': [88, 99, 111],
'Farva': [45, 56, 67],
'Rabbit': [59, 61, 67],
'Ursula': [73, 79, 83],
'Foster': [89, 97, 101]}
In [11]:
grade_lists = build_grade_lists(grades)
grade_lists
In [12]:
import
nb_1_2_tester
tester = nb_1_2_tester.Tester_1_2_2()
for
_ in
range(20):
try
:
tester.run_test(build_grade_lists)
(input_vars, original_input_vars, returned_output_vars, true_o
utput_vars) = tester.get_test_vars()
except
:
(input_vars, original_input_vars, returned_output_vars, true_o
utput_vars) = tester.get_test_vars()
raise
print('Passed. Please submit!')
Exercise 3
(
grade_dicts_test
: 2 points). Complete the function build_grade_dicts
, again taking
grades
as a parameter and returning new dictionary, grade_dicts
, that maps names of students to
dictionaries
containing their scores. Each entry of this scores dictionary should be keyed on assignment name
and hold the corresponding grade as an integer. For instance, grade_dicts['Thorny']['Exam 1'] == 100
. You may find solutions to earlier exercises useful for completing this one. Feel free to use them!
Out[11]:
{'Thorny': [100, 90, 80],
'Mac': [88, 99, 111],
'Farva': [45, 56, 67],
'Rabbit': [59, 61, 67],
'Ursula': [73, 79, 83],
'Foster': [89, 97, 101]}
Passed. Please submit!
2/17/24, 7:37 AM
2-more_exercises
https://inst-fs-iad-prod.inscloudgate.net/files/63a3952e-76eb-48fe-8842-92f453f2a0e1/nb1.2 solutions.html?token=eyJ0eXAiOiJKV1QiLCJhbGciOiJIUzUxMiJ9.e…
5/10
In [13]:
def
build_grade_dicts(grades):
###
### YOUR CODE HERE
###
assignments = get_assignments(grades)
grade_dicts = {} # Empty
for
L in
grades[1:]:
grade_dicts[L[0]] = dict(zip(assignments, [int(g) for
g in
L
[1:]]))
return
grade_dicts
The demo cell below should display
{'Thorny': {'Exam 1': 100, 'Exam 2': 90, 'Exam 3': 80},
'Mac': {'Exam 1': 88, 'Exam 2': 99, 'Exam 3': 111},
'Farva': {'Exam 1': 45, 'Exam 2': 56, 'Exam 3': 67},
'Rabbit': {'Exam 1': 59, 'Exam 2': 61, 'Exam 3': 67},
'Ursula': {'Exam 1': 73, 'Exam 2': 79, 'Exam 3': 83},
'Foster': {'Exam 1': 89, 'Exam 2': 97, 'Exam 3': 101}}
In [14]:
grade_dicts = build_grade_dicts(grades)
grade_dicts
In [15]:
import
nb_1_2_tester
tester = nb_1_2_tester.Tester_1_2_3()
for
_ in
range(20):
try
:
tester.run_test(build_grade_dicts)
(input_vars, original_input_vars, returned_output_vars, true_o
utput_vars) = tester.get_test_vars()
except
:
(input_vars, original_input_vars, returned_output_vars, true_o
utput_vars) = tester.get_test_vars()
raise
print('Passed. Please submit!')
Out[14]:
{'Thorny': {'Exam 1': 100, 'Exam 2': 90, 'Exam 3': 80},
'Mac': {'Exam 1': 88, 'Exam 2': 99, 'Exam 3': 111},
'Farva': {'Exam 1': 45, 'Exam 2': 56, 'Exam 3': 67},
'Rabbit': {'Exam 1': 59, 'Exam 2': 61, 'Exam 3': 67},
'Ursula': {'Exam 1': 73, 'Exam 2': 79, 'Exam 3': 83},
'Foster': {'Exam 1': 89, 'Exam 2': 97, 'Exam 3': 101}}
Passed. Please submit!
2/17/24, 7:37 AM
2-more_exercises
https://inst-fs-iad-prod.inscloudgate.net/files/63a3952e-76eb-48fe-8842-92f453f2a0e1/nb1.2 solutions.html?token=eyJ0eXAiOiJKV1QiLCJhbGciOiJIUzUxMiJ9.e…
6/10
Exercise 4
(
avg_grades_by_student_test
: 1 point). Complete the function build_avg_by_student
,
taking grades
as a parameter and returning a dictionary named avg_by_student
that maps each student to
his or her average exam score. For instance, avg_grades_by_student['Thorny'] == 90
. You may find
solutions to earlier exercises useful for completing this one. Feel free to use them!
Hint.
The statistics
(https://docs.python.org/3.5/library/statistics.html) module of Python
has at least one helpful function.
In [16]:
# Create a dict mapping names to grade averages.
def
build_avg_by_student(grades):
###
### YOUR CODE HERE
###
grade_lists = build_grade_lists(grades)
from
statistics
import
mean
return
{n: mean(G) for
n, G in
grade_lists.items()}
The demo cell below should display
{'Thorny': 90,
'Mac': 99.33333333333333,
'Farva': 56,
'Rabbit': 62.333333333333336,
'Ursula': 78.33333333333333,
'Foster': 95.66666666666667}
In [17]:
avg_grades_by_student = build_avg_by_student(grades)
avg_grades_by_student
Out[17]:
{'Thorny': 90,
'Mac': 99.33333333333333,
'Farva': 56,
'Rabbit': 62.333333333333336,
'Ursula': 78.33333333333333,
'Foster': 95.66666666666667}
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
2/17/24, 7:37 AM
2-more_exercises
https://inst-fs-iad-prod.inscloudgate.net/files/63a3952e-76eb-48fe-8842-92f453f2a0e1/nb1.2 solutions.html?token=eyJ0eXAiOiJKV1QiLCJhbGciOiJIUzUxMiJ9.e…
7/10
In [18]:
import
nb_1_2_tester
tester = nb_1_2_tester.Tester_1_2_4()
for
_ in
range(20):
try
:
tester.run_test(build_avg_by_student)
(input_vars, original_input_vars, returned_output_vars, true_o
utput_vars) = tester.get_test_vars()
except
:
(input_vars, original_input_vars, returned_output_vars, true_o
utput_vars) = tester.get_test_vars()
raise
print('Passed. Please submit!')
Exercise 5
(
grades_by_assignment_test
: 2 points). Complete the function build_grade_by_asn
, which
takes grades
as a parameter and returns a dictionary named grade_by_asn
, whose keys are assignment
(exam) names and whose values are lists of scores over all students on that assignment. For instance,
grades_by_assignment['Exam 1'] == [100, 88, 45, 59, 73, 89]
. You may find solutions to
earlier exercises useful for completing this one. Feel free to use them!
In [19]:
def
build_grade_by_asn(grades):
###
### YOUR CODE HERE
###
assignments = get_assignments(grades)
grades_by_assignment = {} # Empty dictionary
for
k, a in
enumerate(assignments): # (0, 'Exam 1'), ...
grades_by_assignment[a] = [int(L[k+1]) for
L in
grades[1:]]
return
grades_by_assignment
The demo cell below should display
{'Exam 1': [100, 88, 45, 59, 73, 89],
'Exam 2': [90, 99, 56, 61, 79, 97],
'Exam 3': [80, 111, 67, 67, 83, 101]}
In [20]:
grades_by_assignment = build_grade_by_asn(grades)
grades_by_assignment
Passed. Please submit!
Out[20]:
{'Exam 1': [100, 88, 45, 59, 73, 89],
'Exam 2': [90, 99, 56, 61, 79, 97],
'Exam 3': [80, 111, 67, 67, 83, 101]}
2/17/24, 7:37 AM
2-more_exercises
https://inst-fs-iad-prod.inscloudgate.net/files/63a3952e-76eb-48fe-8842-92f453f2a0e1/nb1.2 solutions.html?token=eyJ0eXAiOiJKV1QiLCJhbGciOiJIUzUxMiJ9.e…
8/10
In [21]:
import
nb_1_2_tester
tester = nb_1_2_tester.Tester_1_2_5()
for
_ in
range(20):
try
:
tester.run_test(build_grade_by_asn)
(input_vars, original_input_vars, returned_output_vars, true_o
utput_vars) = tester.get_test_vars()
except
:
(input_vars, original_input_vars, returned_output_vars, true_o
utput_vars) = tester.get_test_vars()
raise
print('Passed. Please submit!')
Exercise 6
(
avg_grades_by_assignment_test
: 1 point). Complete the function build_avg_by_asn
,
which takes grades
as a parameter and returns a dictionary, avg_by_asn
, which maps each exam to its
average score. You may find solutions to earlier exercises useful for completing this one. Feel free to use them!
In [22]:
# Create a dict mapping items to average for that item across all stud
ents.
def
build_avg_by_asn(grades):
###
### YOUR CODE HERE
###
from
statistics
import
mean
grades_by_assignment = build_grade_by_asn(grades)
return
{a: mean(G) for
a, G in
grades_by_assignment.items()}
The demo cell below should display
{'Exam 1': 75.66666666666667,
'Exam 2': 80.33333333333333,
'Exam 3': 84.83333333333333}
In [23]:
avg_grades_by_assignment = build_avg_by_asn(grades)
avg_grades_by_assignment
Passed. Please submit!
Out[23]:
{'Exam 1': 75.66666666666667,
'Exam 2': 80.33333333333333,
'Exam 3': 84.83333333333333}
2/17/24, 7:37 AM
2-more_exercises
https://inst-fs-iad-prod.inscloudgate.net/files/63a3952e-76eb-48fe-8842-92f453f2a0e1/nb1.2 solutions.html?token=eyJ0eXAiOiJKV1QiLCJhbGciOiJIUzUxMiJ9.e…
9/10
In [24]:
import
nb_1_2_tester
tester = nb_1_2_tester.Tester_1_2_6()
for
_ in
range(20):
try
:
tester.run_test(build_avg_by_asn)
(input_vars, original_input_vars, returned_output_vars, true_o
utput_vars) = tester.get_test_vars()
except
:
(input_vars, original_input_vars, returned_output_vars, true_o
utput_vars) = tester.get_test_vars()
raise
print('Passed. Please submit!')
Exercise 7
(
rank_test
: 2 points). Complete the function get_ranked_students
which takes grades
as an
argument and returns a new list, ranked_students
, which contains the names of students in order by
decreasing
score. That is, ranked_students[0]
should contain the name of the top student (highest average
exam score), and ranked_students[-1]
should have the name of the bottom student (lowest average exam
score). You may find solutions to earlier exercises useful for completing this one. Feel free to use them!
In [25]:
def
get_ranked_students(grades):
###
### YOUR CODE HERE
###
avg_grades_by_student = build_avg_by_student(grades)
return
sorted(avg_grades_by_student, key=avg_grades_by_student.ge
t, reverse=
True
)
The demo cell below shuould display ['Mac', 'Foster', 'Thorny', 'Ursula', 'Rabbit', 'Farva']
In [26]:
rank = get_ranked_students(grades)
rank
In [27]:
import
nb_1_2_tester
tester = nb_1_2_tester.Tester_1_2_7()
for
i in
range(5):
try
:
tester.run_test(get_ranked_students)
(input_vars, original_input_vars, returned_output_vars, true_o
utput_vars) = tester.get_test_vars()
except
:
(input_vars, original_input_vars, returned_output_vars, true_o
utput_vars) = tester.get_test_vars()
raise
print('Passed. Please submit!')
Passed. Please submit!
Out[26]:
['Mac', 'Foster', 'Thorny', 'Ursula', 'Rabbit', 'Farva']
Passed. Please submit!
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
2/17/24, 7:37 AM
2-more_exercises
https://inst-fs-iad-prod.inscloudgate.net/files/63a3952e-76eb-48fe-8842-92f453f2a0e1/nb1.2 solutions.html?token=eyJ0eXAiOiJKV1QiLCJhbGciOiJIUzUxMiJ9.…
10/10
Fin!
You've reached the end of this part. Don't forget to restart and run all cells again to make sure it's all
working when run in sequence; and make sure your work passes the submission process. Good luck!
Related Documents
Recommended textbooks for you
Np Ms Office 365/Excel 2016 I Ntermed
Computer Science
ISBN:9781337508841
Author:Carey
Publisher:Cengage
COMPREHENSIVE MICROSOFT OFFICE 365 EXCE
Computer Science
ISBN:9780357392676
Author:FREUND, Steven
Publisher:CENGAGE L
Microsoft Windows 10 Comprehensive 2019
Computer Science
ISBN:9780357392607
Author:FREUND
Publisher:Cengage
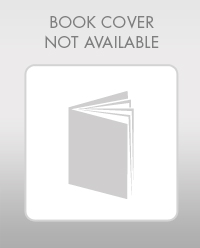
A+ Guide To It Technical Support
Computer Science
ISBN:9780357108291
Author:ANDREWS, Jean.
Publisher:Cengage,
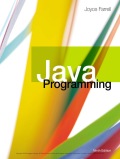
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
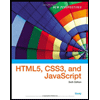
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:9781305503922
Author:Patrick M. Carey
Publisher:Cengage Learning
Recommended textbooks for you
- Np Ms Office 365/Excel 2016 I NtermedComputer ScienceISBN:9781337508841Author:CareyPublisher:CengageCOMPREHENSIVE MICROSOFT OFFICE 365 EXCEComputer ScienceISBN:9780357392676Author:FREUND, StevenPublisher:CENGAGE LMicrosoft Windows 10 Comprehensive 2019Computer ScienceISBN:9780357392607Author:FREUNDPublisher:Cengage
- A+ Guide To It Technical SupportComputer ScienceISBN:9780357108291Author:ANDREWS, Jean.Publisher:Cengage,EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTNew Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage Learning
Np Ms Office 365/Excel 2016 I Ntermed
Computer Science
ISBN:9781337508841
Author:Carey
Publisher:Cengage
COMPREHENSIVE MICROSOFT OFFICE 365 EXCE
Computer Science
ISBN:9780357392676
Author:FREUND, Steven
Publisher:CENGAGE L
Microsoft Windows 10 Comprehensive 2019
Computer Science
ISBN:9780357392607
Author:FREUND
Publisher:Cengage
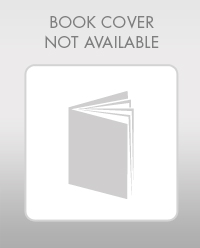
A+ Guide To It Technical Support
Computer Science
ISBN:9780357108291
Author:ANDREWS, Jean.
Publisher:Cengage,
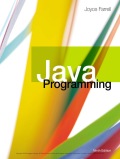
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
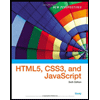
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:9781305503922
Author:Patrick M. Carey
Publisher:Cengage Learning