lab_1_learning_python
py
keyboard_arrow_up
School
University of Texas, Rio Grande Valley *
*We aren’t endorsed by this school
Course
2350
Subject
Civil Engineering
Date
Apr 3, 2024
Type
py
Pages
3
Uploaded by suckmyassbuddy
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Recommended textbooks for you
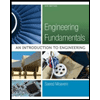
Engineering Fundamentals: An Introduction to Engi...
Civil Engineering
ISBN:9781305084766
Author:Saeed Moaveni
Publisher:Cengage Learning
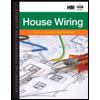
Residential Construction Academy: House Wiring (M...
Civil Engineering
ISBN:9781285852225
Author:Gregory W Fletcher
Publisher:Cengage Learning
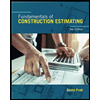
Fundamentals Of Construction Estimating
Civil Engineering
ISBN:9781337399395
Author:Pratt, David J.
Publisher:Cengage,
Recommended textbooks for you
- Engineering Fundamentals: An Introduction to Engi...Civil EngineeringISBN:9781305084766Author:Saeed MoaveniPublisher:Cengage LearningResidential Construction Academy: House Wiring (M...Civil EngineeringISBN:9781285852225Author:Gregory W FletcherPublisher:Cengage LearningFundamentals Of Construction EstimatingCivil EngineeringISBN:9781337399395Author:Pratt, David J.Publisher:Cengage,
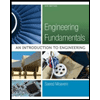
Engineering Fundamentals: An Introduction to Engi...
Civil Engineering
ISBN:9781305084766
Author:Saeed Moaveni
Publisher:Cengage Learning
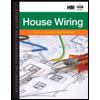
Residential Construction Academy: House Wiring (M...
Civil Engineering
ISBN:9781285852225
Author:Gregory W Fletcher
Publisher:Cengage Learning
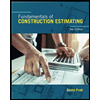
Fundamentals Of Construction Estimating
Civil Engineering
ISBN:9781337399395
Author:Pratt, David J.
Publisher:Cengage,