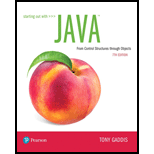
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
7th Edition
ISBN: 9780134802213
Author: Tony Gaddis
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Textbook Question
Chapter 9, Problem 8AW
Look at the following string:
“cookies>milk>fudge:cake:ice cream”
Write code using the String class’s split method that can be used to extract the following tokens from the string: cookies, milk, fudge, cake, and ice cream.
Expert Solution & Answer

Learn your wayIncludes step-by-step video

schedule07:09
Students have asked these similar questions
I help understanding this question
d'y + 4dy +3y = a, Initial Conditions: y(0) = 5 & y'(0)=0
Where a = 10
a) Find y(t) =yh(t) +yp(t) in time domainIs the system over-damped, under-damped, or critical?
b) Find y(t) using Laplace Transforms
Given f(t)=a sin(ßt)
a = 10 & ß = 23
Find the Laplace Transform using the definition F(s) = ∫f(t)e-stdt
Please do not use any AI tools to solve this question.
I need a fully manual, step-by-step solution with clear explanations, as if it were done by a human tutor.
No AI-generated responses, please.
Chapter 9 Solutions
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
Ch. 9.2 - Prob. 9.1CPCh. 9.2 - Write an if statement that displays the word digit...Ch. 9.2 - Prob. 9.3CPCh. 9.2 - Write a loop that asks the user, Do you want to...Ch. 9.2 - Prob. 9.5CPCh. 9.2 - Write a loop that counts the number of uppercase...Ch. 9.3 - Prob. 9.7CPCh. 9.3 - Modify the method you wrote for Checkpoint 9.7 so...Ch. 9.3 - Look at the following declaration: String cafeName...Ch. 9.3 - Prob. 9.10CP
Ch. 9.3 - Prob. 9.11CPCh. 9.3 - Prob. 9.12CPCh. 9.3 - Prob. 9.13CPCh. 9.3 - Look at the following code: String str1 = To be,...Ch. 9.3 - Prob. 9.15CPCh. 9.3 - Assume that a program has the following...Ch. 9.4 - Prob. 9.17CPCh. 9.4 - Prob. 9.18CPCh. 9.4 - Prob. 9.19CPCh. 9.4 - Prob. 9.20CPCh. 9.4 - Prob. 9.21CPCh. 9.4 - Prob. 9.22CPCh. 9.4 - Prob. 9.23CPCh. 9.4 - Prob. 9.24CPCh. 9.5 - Prob. 9.25CPCh. 9.5 - Prob. 9.26CPCh. 9.5 - Look at the following string:...Ch. 9.5 - Prob. 9.28CPCh. 9.6 - Write a statement that converts the following...Ch. 9.6 - Prob. 9.30CPCh. 9.6 - Prob. 9.31CPCh. 9 - The isDigit, isLetter, and isLetterOrDigit methods...Ch. 9 - Prob. 2MCCh. 9 - The startsWith, endsWith, and regionMatches...Ch. 9 - The indexOf and lastIndexOf methods are members of...Ch. 9 - Prob. 5MCCh. 9 - Prob. 6MCCh. 9 - Prob. 7MCCh. 9 - Prob. 8MCCh. 9 - Prob. 9MCCh. 9 - Prob. 10MCCh. 9 - To delete a specific character in a StringBuilder...Ch. 9 - Prob. 12MCCh. 9 - This String method breaks a string into tokens. a....Ch. 9 - These static final variables are members of the...Ch. 9 - Prob. 15TFCh. 9 - Prob. 16TFCh. 9 - True or False: If toLowerCase methods argument is...Ch. 9 - True or False: The startsWith and endsWith methods...Ch. 9 - True or False: There are two versions of the...Ch. 9 - Prob. 20TFCh. 9 - Prob. 21TFCh. 9 - Prob. 22TFCh. 9 - Prob. 23TFCh. 9 - int number = 99; String str; // Convert number to...Ch. 9 - Prob. 2FTECh. 9 - Prob. 3FTECh. 9 - Prob. 4FTECh. 9 - The following if statement determines whether...Ch. 9 - Write a loop that counts the number of space...Ch. 9 - Prob. 3AWCh. 9 - Prob. 4AWCh. 9 - Prob. 5AWCh. 9 - Modify the method you wrote for Algorithm...Ch. 9 - Prob. 7AWCh. 9 - Look at the following string:...Ch. 9 - Assume that d is a double variable. Write an if...Ch. 9 - Write code that displays the contents of the int...Ch. 9 - Prob. 1SACh. 9 - Prob. 2SACh. 9 - Prob. 3SACh. 9 - How can you determine the minimum and maximum...Ch. 9 - Prob. 1PCCh. 9 - Prob. 2PCCh. 9 - Prob. 3PCCh. 9 - Prob. 4PCCh. 9 - Prob. 5PCCh. 9 - Prob. 6PCCh. 9 - Check Writer Write a program that displays a...Ch. 9 - Prob. 8PCCh. 9 - Prob. 9PCCh. 9 - Word Counter Write a program that asks the user...Ch. 9 - Sales Analysis The file SalesData.txt, in this...Ch. 9 - Prob. 12PCCh. 9 - Alphabetic Telephone Number Translator Many...Ch. 9 - Word Separator Write a program that accepts as...Ch. 9 - Pig Latin Write a program that reads a sentence as...Ch. 9 - Prob. 16PCCh. 9 - Lottery Statistics To play the PowerBall lottery,...Ch. 9 - Gas Prices In the student sample program files for...
Additional Engineering Textbook Solutions
Find more solutions based on key concepts
This optional Google account security feature sends you a message with a code that you must enter, in addition ...
SURVEY OF OPERATING SYSTEMS
What happens when you serialize an object? What happens when you deserialize an object?
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
Why must the parameter of a copy constructor be a reference?
Starting Out with C++ from Control Structures to Objects (9th Edition)
In Exercises 41 through 46, identify the errors. Dim9WAsDouble9W=2*9WIstoutput.Items.Add(9W)
Introduction To Programming Using Visual Basic (11th Edition)
Explain how each of the following types of integrity constraints is enforced in the SQL CREATE TABLE commands: ...
Modern Database Management
Write a void method called display such that the invocation display (a) will display the contents of the array ...
Java: An Introduction to Problem Solving and Programming (8th Edition)
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Obtain the MUX design for the function F(X,Y,Z) = (0,3,4,7) using an off-the-shelf MUX with an active low strobe input (E).arrow_forwardI cannot program smart home automation rules from my device using a computer or phone, and I would like to know how to properly connect devices such as switches and sensors together ? Cisco Packet Tracer 1. Smart Home Automation:o Connect a temperature sensor and a fan to a home gateway.o Configure the home gateway so that the fan is activated when the temperature exceedsa set threshold (e.g., 30°C).2. WiFi Network Configuration:o Set up a wireless LAN with a unique SSID.o Enable WPA2 encryption to secure the WiFi network.o Implement MAC address filtering to allow only specific clients to connect.3. WLC Configuration:o Deploy at least two wireless access points connected to a Wireless LAN Controller(WLC).o Configure the WLC to manage the APs, broadcast the configured SSID, and applyconsistent security settings across all APs.arrow_forwardusing r language for integration theta = integral 0 to infinity (x^4)*e^(-x^2)/2 dx (1) use the density function of standard normal distribution N(0,1) f(x) = 1/sqrt(2pi) * e^(-x^2)/2 -infinity <x<infinity as importance function and obtain an estimate theta 1 for theta set m=100 for the estimate whatt is the estimate theta 1? (2)use the density function of gamma (r=5 λ=1/2)distribution f(x)=λ^r/Γ(r) x^(r-1)e^(-λx) x>=0 as importance function and obtain an estimate theta 2 for theta set m=1000 fir the estimate what is the estimate theta2? (3) use simulation (repeat 1000 times) to estimate the variance of the estimates theta1 and theta 2 which one has smaller variance?arrow_forward
- using r language A continuous random variable X has density function f(x)=1/56(3x^2+4x^3+5x^4).0<=x<=2 (1) secify the density g of the random variable Y you find for the acceptance rejection method. (2) what is the value of c you choose to use for the acceptance rejection method (3) use the acceptance rejection method to generate a random sample of size 1000 from the distribution of X .graph the density histogram of the sample and compare it with the density function f(x)arrow_forwardusing r language a continuous random variable X has density function f(x)=1/4x^3e^-(pi/2)^4,x>=0 derive the probability inverse transformation F^(-1)x where F(x) is the cdf of the random variable Xarrow_forwardusing r language in an accelerated failure test, components are operated under extreme conditions so that a substantial number will fail in a rather short time. in such a test involving two types of microships 600 chips manufactured by an existing process were tested and 125 of them failed then 800 chips manufactured by a new process were tested and 130 of them failed what is the 90%confidence interval for the difference between the proportions of failure for chips manufactured by two processes? using r languagearrow_forward
- I want a picture of the tools and the pictures used Cisco Packet Tracer Smart Home Automation:o Connect a temperature sensor and a fan to a home gateway.o Configure the home gateway so that the fan is activated when the temperature exceedsa set threshold (e.g., 30°C).2. WiFi Network Configuration:o Set up a wireless LAN with a unique SSID.o Enable WPA2 encryption to secure the WiFi network.o Implement MAC address filtering to allow only specific clients to connect.3. WLC Configuration:o Deploy at least two wireless access points connected to a Wireless LAN Controller(WLC).o Configure the WLC to manage the APs, broadcast the configured SSID, and applyconsistent security settings across all APs.arrow_forwardA. What will be printed executing the code above?B. What is the simplest way to set a variable of the class Full_Date to January 26 2020?C. Are there any empty constructors in this class Full_Date?a. If there is(are) in which code line(s)?b. If there is not, how would an empty constructor be? (create the code lines for it)D. Can the command std::cout << d1.m << std::endl; be included after line 28 withoutcausing an error?a. If it can, what will be printed?b. If it cannot, how could this command be fixed?arrow_forwardCisco Packet Tracer Smart Home Automation:o Connect a temperature sensor and a fan to a home gateway.o Configure the home gateway so that the fan is activated when the temperature exceedsa set threshold (e.g., 30°C).2. WiFi Network Configuration:o Set up a wireless LAN with a unique SSID.o Enable WPA2 encryption to secure the WiFi network.o Implement MAC address filtering to allow only specific clients to connect.3. WLC Configuration:o Deploy at least two wireless access points connected to a Wireless LAN Controller(WLC).o Configure the WLC to manage the APs, broadcast the configured SSID, and applyconsistent security settings across all APs.arrow_forward
- Transform the TM below that accepts words over the alphabet Σ= {a, b} with an even number of a's and b's in order that the output tape head is positioned over the first letter of the input, if the word is accepted, and all letters a should be replaced by the letter x. For example, for the input aabbaa the tape and head at the end should be: [x]xbbxx z/z,R b/b,R F ① a/a,R b/b,R a/a, R a/a,R b/b.R K a/a,R L b/b,Rarrow_forwardGiven the C++ code below, create a TM that performs the same operation, i.e., given an input over the alphabet Σ= {a, b} it prints the number of letters b in binary. 1 #include 2 #include 3 4- int main() { std::cout > str; for (char c : str) { if (c == 'b') count++; 5 std::string str; 6 int count = 0; 7 char buffer [1000]; 8 9 10 11- 12 13 14 } 15 16- 17 18 19 } 20 21 22} std::string binary while (count > 0) { binary = std::to_string(count % 2) + binary; count /= 2; std::cout << binary << std::endl; return 0;arrow_forwardConsidering the CFG described below, answer the following questions. Σ = {a, b} • NT = {S} Productions: P1 S⇒aSa P2 P3 SbSb S⇒ a P4 S⇒ b A. List one sequence of productions that can accept the word abaaaba; B. Give three 5-letter words that can be accepted by this CFG; C. Create a Pushdown automaton capable of accepting the language accepted by this CFG.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage LearningC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
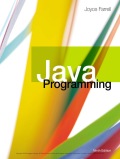
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
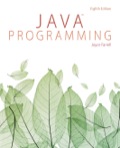
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781305480537
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
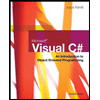
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
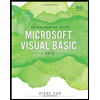
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning
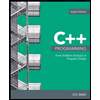
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
Program to find HCF & LCM of two numbers in C | #6 Coding Bytes; Author: FACE Prep;https://www.youtube.com/watch?v=mZA3cdalYN4;License: Standard YouTube License, CC-BY