Consider the following program written in C syntax:
void fun (int first, int second) {
first += first;
second += second;
}
Void main () {
int list [2] = {1, 3};
fun (list [0], list[1]);
}
For each of the following parameter-passing methods, what are the values of the list array after execution?
- a. Passed by value
- b. Passed by reference
- c. Passed by value-result

Trending nowThis is a popular solution!

Chapter 9 Solutions
Concepts Of Programming Languages
Additional Engineering Textbook Solutions
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
Elementary Surveying: An Introduction To Geomatics (15th Edition)
Thinking Like an Engineer: An Active Learning Approach (4th Edition)
Management Information Systems: Managing The Digital Firm (16th Edition)
- emacs/lisp function Write a function in Lisp that takes one parameter which we can assume to be a list. The function should print all the elements of the list using the function princ and a space between them. Use the function mapc for this purpose.Show a few examples of testing this function. Show one example using the function apply and one using the function funcall.arrow_forwardvoid listEmployees (void) { for (int i=0; i 10000. Make a guess about why the comparison function takes 2 struct Employee parameters (as opposed to struct Employee *) **arrow_forwardComputer science JAVA programming languagearrow_forward
- in haskell: Write a function sumNums that adds the items in a list and returns a list of the running totals. For example: sumNums [2,3,4,5] returns [2,5,9,14].arrow_forwardclass Solution(object): def longestCommonPrefix(self, strs): result ="" for i in strs[0]: for j in strs[1]: for k in strs[2]: if i == j and i == k: result+=i if len(result) >0: return result else: return result IndexError: list index out of range for j in strs[1]: Line 5 in longestCommonPrefix (Solution.py) ret = Solution().longestCommonPrefix(param_1) Line 31 in _driver (Solution.py) _driver() Line 41 in <module> (Solution.py) can someone explain why this is wrong?arrow_forwardWrite JAVA code General Problem Description: It is desired to develop a directory application based on the use of a double-linked list data structure, in which students are kept in order according to their student number. In this context, write the Java codes that will meet the requirements given in detail below. Requirements: Create a class named Student to represent students. In the Student class; student number, name and surname and phone numbers for communication are kept. Student's multiple phones number (multiple mobile phones, home phones, etc.) so phone numbers information will be stored in an “ArrayList”. In the Student class; parameterless, taking all parameters and It is sufficient to have 3 constructor methods, including a copy constructor, get/set methods and toString.arrow_forward
- Consider the following program written in C syntax: void fun (int first, int second) { first += first; second += second; } void main() { int list[2] = {1, 3}; fun(list[0], list[1]); } For each of the following parameter-passing methods, what are the values of the list array after execution? Passed by value Passed by reference Passed by value-resultarrow_forwardobject oriented c++arrow_forwardOld MathJax webview Old MathJax webview In Java Some methods of the singly linked list listed below can be implemented efficiently (in different respects) (as opposed to an array or a doubly linked list), others not necessarily which are they and why? b. Implement a function to add an element before the first element. c. Implement a function to add an item after the last one element. d. Implement a function to output an element of the list. e. Implement a function to output the entire list. f. Implement a function to output the number of elements. G. Implement a function to delete an item. H. Implement a function to clear the entire list. I. Implement functionality to search for one or more students by first name, last name, matriculation number or course of study. J. Implement functionality to sort the records of the student, matriculation number and course according to two self-selected sorting methods.arrow_forward
- in Haskell: Write a function diffList with two lists as parameters that deletes every occurrence of every element of the second list from the first list. This is analogous to a set difference.arrow_forwardC++ Data structure question, pleaze help me quickly, I'm in an exam. ?arrow_forwardTopic: SIngly Linked ListUsing C++ Programming, improve the implemented following function. Initial code is attached below. int count(int num) This will return the count of the instances of the element num in the list. In the linked list in removeAll method, having the method count(10) will return 3 as there are three 10's in the linked list. int count(int num) { int count = 0; for(int i=1; i<index;i++){ if(get(i)== num){ count++; } } return count; } OUTPUT: 1 2 3 4 5 6 7 8 9 2 0 0 10 -> 30 -> 40 -> 10 -> 20 -> 40 -> 10 EXPECTED OUT PUT: 1 2 3 4 5 6 7 8 9 2 10 40 10 -> 30 -> 40 -> 10 -> 20arrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
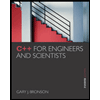