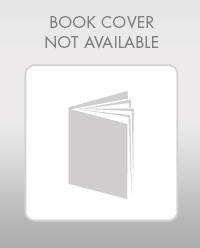
Starting Out With C++: Early Objects (10th Edition)
10th Edition
ISBN: 9780135235003
Author: Tony Gaddis, Judy Walters, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 9, Problem 14PC
Program Plan Intro
Using Files-Birthday Look Up
Program Plan:
- Include the required header files.
- Create a class named “Birthday”.
- Inside the “private” access specifier, declare the variables “name” and “birthday”.
- Declare a variable “TOTAL” as “10”.
- Declare function prototype.
- Define the “main()” function.
- Create an array object for class.
- Call the function “getBirthdate()” function to read the birthday date from the file.
- Call the function “display()” to display the date in ascending order.
- Call the function “getResponse()” to get the response from the user .
- Call the function “Sort_selection()” function to sort the birth date.
- Call the function “find_date()” to print the corresponding name and date.
- Function definition for the function “getBirthdate()”.
- Create an object to read input from the file.
- Open the file and test that it opened correctly.
- Checking condition for opening the file.
- Print the statement.
- Otherwise, read the data form the file.
- Read the name from the file.
- Read the birthday date from the file.
- Close the file.
- Otherwise, read the data form the file.
- Print the statement.
- Function definition for the function “sort_selection ()”.
- Variable to store the start value and the minimum birthday date index.
- Create an object for class.
- Variable to store the minimum date value.
- Iterate from“start” until it reaches “(TOTAL-1)”
- Assign “start” to “ind_val”
- Assign “frds_date[start].birthday” to “min”
- Iterate from “index” until it reaches the “TOTAL”.
- Compare data in the birthday fields
- Swap records in “frds_date[start]” and “array[ind_val]”.
- Function definition for the function “display()”.
- Iterate from the “position” until it reaches the “TOTAL”.
- Set the precision for name and birthday date.
- Iterate from the “position” until it reaches the “TOTAL”.
- Function definition for function “getResponse()”.
- Variable to hold the user response.
- Get the response from the user.
- Check the response for validation.
- Validate the user response.
- Return the response.
- Validate the user response.
- Function definition for “find_date()”.
- Print the corresponding name and birthday date.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Date Printer Write a program that reads a char array from the user containinga date in the form mm/dd/yyyy. It should print the date in the form March 12, 2014.
The variables numberArray1 and numberArray2 reference arrays that have 100 elements each. Write code that copies the values from numberArray1 to numberArray2
Hands-On Activities
Hands-On Activity 10-1
Write a pseudocode program that creates an array, prompts the
user to enter five cities, and displays the array's contents. The
contains five elements. Use a For loop to prompt the user to enter
cities, and then use another For loop to display the contents. Save the
file as cityArray.txt.
array
Chapter 9 Solutions
Starting Out With C++: Early Objects (10th Edition)
Ch. 9.2 - Prob. 9.1CPCh. 9.2 - Prob. 9.2CPCh. 9.2 - Prob. 9.3CPCh. 9.2 - Prob. 9.4CPCh. 9.3 - True or false: Any sort can be modified to sort in...Ch. 9.3 - Prob. 9.6CPCh. 9.3 - Prob. 9.7CPCh. 9.3 - Prob. 9.8CPCh. 9.3 - Prob. 9.9CPCh. 9.6 - Prob. 9.10CP
Ch. 9.6 - Prob. 9.11CPCh. 9.6 - Prob. 9.12CPCh. 9.6 - Prob. 9.13CPCh. 9.6 - Prob. 9.14CPCh. 9.6 - Prob. 9.15CPCh. 9 - Prob. 1RQECh. 9 - Prob. 2RQECh. 9 - Prob. 3RQECh. 9 - Prob. 4RQECh. 9 - Prob. 5RQECh. 9 - Prob. 6RQECh. 9 - Prob. 7RQECh. 9 - A binary search will find the value it is looking...Ch. 9 - The maximum number of comparisons that a binary...Ch. 9 - Prob. 11RQECh. 9 - Prob. 12RQECh. 9 - Bubble sort places ______ number(s) in place on...Ch. 9 - Selection sort places ______ number(s) in place on...Ch. 9 - Prob. 15RQECh. 9 - Prob. 16RQECh. 9 - Why is selection sort more efficient than bubble...Ch. 9 - Prob. 18RQECh. 9 - Prob. 19RQECh. 9 - Prob. 20RQECh. 9 - Prob. 21RQECh. 9 - Charge Account Validation Write a program that...Ch. 9 - Lottery Winners A lottery ticket buyer purchases...Ch. 9 - Lottery Winners Modification Modify the program...Ch. 9 - Batting Averages Write a program that creates and...Ch. 9 - Hit the Slopes Write a program that can be used by...Ch. 9 - String Selection Sort Modify the selectionSort...Ch. 9 - Binary String Search Modify the binarySearch...Ch. 9 - Search Benchmarks Write a program that has at...Ch. 9 - Sorting Benchmarks Write a program that uses two...Ch. 9 - Sorting Orders Write a program that uses two...Ch. 9 - Ascending Circles Program 8-31 from Chapter 8...Ch. 9 - Modified Bin Manager Class Modify the BinManager...Ch. 9 - Using Files-Birthday List Write a program that...Ch. 9 - Prob. 14PCCh. 9 - Using Files-String Selection Sort Modification...Ch. 9 - Using Vectors String Selection Sort Modification...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Write a statement that declares a procedure-level one-dimensional array named intorders. The array should contain 15 elements.arrow_forward(Statistics) Write a program that includes two functions named calcavg() and variance(). The calcavg() function should calculate and return the average of values stored in an array named testvals. The array should be declared in main() and include the values 89, 95, 72, 83, 99, 54, 86, 75, 92, 73, 79, 75, 82, and 73. The variance() function should calculate and return the variance of the data. The variance is obtained by subtracting the average from each value in testvals, squaring the values obtained, adding them, and dividing by the number of elements in testvals. The values returned from calcavg() and variance() should be displayed by using cout statements in main().arrow_forwardProblem Charge Account Validation Create an application that reads the contents of the file into an array or a List . The application should then let the user enter a charge account number. The program should determine whether the number is valid by searching for it in the array or List that contains the valid charge account numbers. If the number is in the array or List , the program should display a message indicating the number is valid. If the number is not in the array or List , the program should display a message indicating the number is invalid. For Visual Studio .Net 4.6.1 current solution on bartleby does not work as it does not actually use a list and does not properly retrieve the file file is a text document with 18 7 digit numbers named ChargeAccounts.txtarrow_forward
- The arrays array1 and array2 each hold 25 integer elements. Write code that copies the values in array1 to array2.arrow_forwardYou can't transfer data from one array to another with the operator in a single sentence.arrow_forwardThe strItems array is declared as follows: Dim strItems(20) As String. The intSub variable keeps track of the array subscripts and is initialized to 0. Which of the following Do clauses will process the loop instructions for each element in the array? a. Do While intSub > 20 b. Do While intSub < 20 c. Do While intSub >= 20 d. Do While intSub <= 20arrow_forward
- Question Write a statement that declares a string arrays initialized with the following strings: Write a loop that display the content of each element in the array declared in question 1 "Monday", "Tuesday", "Wednesday", "Thursday", "Friday", "Saturday" and "Sunday"arrow_forwardAn array can hold as much different data types as you want. True Falsearrow_forward# Declare a named constant for array size here. MAX_AVERAGES = 8 # Declare array here. # Write a loop to get batting averages from user and assign to array. averageString = input("Enter a batting average: ") battingAverage = float(averageString) # Assign value to array. # Assign the first element in the array to be the minimum and the maximum. minAverage = averages[0] maxAverage = averages[0] # Start out your total with the value of the first element in the array. total = averages[0] # Write a loop here to access array values starting with averages[1] # Within the loop test for minimum and maximum batting averages. # Also accumulate a total of all batting averages. # Calculate the average of the 8 batting averages. # Print the batting averages stored in the averages array. # Print the maximum batting average, minimum batting average, and average batting average. This is on mindtap pyton what needs to be fixarrow_forward
- Complete the following in Pseudocode Write the pseudocode for an application that will request three numbers from a user. The application should then determine and display the largest of the three numbers. After that: Declare an array called ‘numbers’ that will contain the following values: 16, 5, 3, 24.• Ask a user whether they would like to search for a particular value. If their answer is “Yes”, the application should allow them to enter a search value and the array must be searched for that value.• Display a message indicating if the value was found in the array or not. Thenarrow_forwardUsing C# in Microsoft Visual Studio Create an application that simulates a game of tic-tac-toe. The application should use a two-dimensional int array to simulate the game board in memory. When the user clicks the New Game button, the application should step through the array, storing a random number in the range of 0 through 1 in each element.The number 0 represents the letter O, and the number 1 represents the letterX. The form should then be updated to display the game board. The application should display a message indicating whether player X won, player Y won, or the game was a tie.arrow_forwardAlphabet Random Walk• Write a program to generate a random walk that spans a 10*10 character array (The initial values of the elements are all.). The program must randomly walk from one element to another, moving one element position up, down, left or right each time. The elements that have been visited are labeled with the letters A through Z in the order in which they were visitedarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage LearningMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
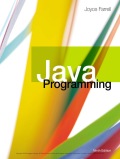
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
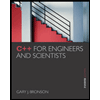
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
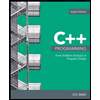
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
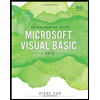
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning
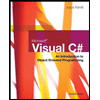
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,