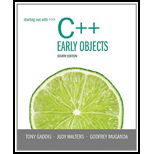
Starting Out with C++: Early Objects
8th Edition
ISBN: 9780133360929
Author: Tony Gaddis, Judy Walters, Godfrey Muganda
Publisher: Addison-Wesley
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 8.12, Problem 8.37CP
Program Plan Intro
Array of structures:
In C++, it is possible to create an array of structure; it is used to store a group of records contains multiple data members of various data type. A single array of structure can replace many arrays of regular type variable.
- Array of structures can be defined like normal array.
The structure array is declared through the following format:
struct_name variable[size];
Consider the following array definition:
//Declaration of structure array
Car values[5];
- In the above example, “Car” represents the structure type of the array, “values[]” is the array name, and the number inside the brackets is the size declaratory of the array.
Subscript of an array:
In C++, array elements can be accessed using a subscript. In an array, each element assigned with the unique number, which is specified inside the brackets, is referred as a subscript.
- By default, structure members are defined as “public”. Hence structure members can be accessed by place the dot operator (.) and member name after the array subscript.
Example:
//Assign the value for model member of values[2].
values[2].model = "New"
- In above statement, the value “New” is assigned in the third element of the “values” array for model member.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Describe three (3) Multiplexing techniques common for fiber optic links
Could you help me to know features of the following concepts:
- commercial CA
- memory integrity
- WMI filter
Briefly describe the issues involved in using ATM technology in Local Area Networks
Chapter 8 Solutions
Starting Out with C++: Early Objects
Ch. 8.3 - Define the following arrays: A) empNum, a 100...Ch. 8.3 - Prob. 8.2CPCh. 8.3 - Prob. 8.3CPCh. 8.3 - Prob. 8.4CPCh. 8.3 - What is array bounds checking? Does C++ perform...Ch. 8.3 - What is the output of the following code? int...Ch. 8.3 - Complete the following program skeleton so it will...Ch. 8.6 - Define the following arrays: A) ages, a 10-element...Ch. 8.6 - Indicate if each of the following array...Ch. 8.6 - Prob. 8.10CP
Ch. 8.6 - Given the following array definition: int values...Ch. 8.6 - Prob. 8.12CPCh. 8.6 - Prob. 8.13CPCh. 8.6 - What is the output of the following code? const...Ch. 8.8 - Write a typedef statement that makes the name...Ch. 8.8 - Prob. 8.16CPCh. 8.8 - What is the output of the following program...Ch. 8.8 - The following program segments, when completed,...Ch. 8.10 - Prob. 8.19CPCh. 8.10 - Prob. 8.20CPCh. 8.10 - Prob. 8.21CPCh. 8.10 - Prob. 8.22CPCh. 8.10 - Prob. 8.23CPCh. 8.10 - Fill in the empty table below so it shows the...Ch. 8.10 - Write a function called displayArray7. The...Ch. 8.10 - Prob. 8.26CPCh. 8.11 - Prob. 8.27CPCh. 8.11 - Write definition statements for the following...Ch. 8.11 - Define gators to be an empty vector of ints and...Ch. 8.12 - True or false: The default constructor is the only...Ch. 8.12 - True or false: All elements in an array of objects...Ch. 8.12 - What will the following program display on the...Ch. 8.12 - Complete the following program so that it defines...Ch. 8.12 - Add two constructors to the Product structure...Ch. 8.12 - Prob. 8.35CPCh. 8.12 - Prob. 8.36CPCh. 8.12 - Prob. 8.37CPCh. 8.12 - Write the definition for an array of five Product...Ch. 8.12 - Write a structure declaration called Measurement...Ch. 8.12 - Write a structure declaration called Destination ,...Ch. 8.12 - Define an array of 20 Destination structures (see...Ch. 8 - The ________ indicates the number of elements, or...Ch. 8 - The size declarator must be a(n) _______ with a...Ch. 8 - Prob. 3RQECh. 8 - Prob. 4RQECh. 8 - The number inside the brackets of an array...Ch. 8 - C++ has no array ________ checking, which means...Ch. 8 - Prob. 7RQECh. 8 - If a numeric array is partially initialized, the...Ch. 8 - If the size declarator of an array definition is...Ch. 8 - Prob. 10RQECh. 8 - Prob. 11RQECh. 8 - Prob. 12RQECh. 8 - Arrays are never passed to functions by _______...Ch. 8 - To pass an array to a function, pass the ________...Ch. 8 - A(n) ________ array is like several arrays of the...Ch. 8 - Its best to think of a two -dimensional array as...Ch. 8 - Prob. 17RQECh. 8 - Prob. 18RQECh. 8 - When a two -dimensional array is passed to a...Ch. 8 - Prob. 20RQECh. 8 - Look at the following array definition. int values...Ch. 8 - Given the following array definition: int values...Ch. 8 - Prob. 23RQECh. 8 - Assume that array1 and array2 are both 25-element...Ch. 8 - Prob. 25RQECh. 8 - How do you establish a parallel relationship...Ch. 8 - Look at the following array definition. double...Ch. 8 - Prob. 28RQECh. 8 - Prob. 29RQECh. 8 - Prob. 30RQECh. 8 - Prob. 31RQECh. 8 - The following code totals the values in each of...Ch. 8 - In a program you need to store the identification...Ch. 8 - Prob. 34RQECh. 8 - Prob. 35RQECh. 8 - Prob. 36RQECh. 8 - Prob. 37RQECh. 8 - Prob. 38RQECh. 8 - Each of the following functions contains errors....Ch. 8 - Soft Skills Diagrams are an important means of...Ch. 8 - Perfect Scores 1. Write a modular program that...Ch. 8 - Roman Numeral Converter Write a program that...Ch. 8 - Chips and Salsa Write a program that lets a maker...Ch. 8 - Monkey Business A local zoo wants to keep track of...Ch. 8 - Rain or Shine An amateur meteorologist wants to...Ch. 8 - Lottery Write a program that simulates a lottery....Ch. 8 - Rainfall Statistics Write a modular program that...Ch. 8 - Chips and Salsa Version 2 Revise Programming...Ch. 8 - Stats Class and Rainfall Statistics Create a Stats...Ch. 8 - Stats Class and Track Statistics Write a client...Ch. 8 - Prob. 11PCCh. 8 - Drivers License Exam The State Department of Motor...Ch. 8 - Array of Payro11 Objects Design a PayRoll class...Ch. 8 - Drink Machine Simulator Create a class that...Ch. 8 - Bin Manager Class Design and write an object...Ch. 8 - Tic-Tac-Toe Game Write a modular program that...Ch. 8 - Theater Ticket Sales Create a TicketManager class...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- For this question you will perform two levels of quicksort on an array containing these numbers: 59 41 61 73 43 57 50 13 96 88 42 77 27 95 32 89 In the first blank, enter the array contents after the top level partition. In the second blank, enter the array contents after one more partition of the left-hand subarray resulting from the first partition. In the third blank, enter the array contents after one more partition of the right-hand subarray resulting from the first partition. Print the numbers with a single space between them. Use the algorithm we covered in class, in which the first element of the subarray is the partition value. Question 1 options: Blank # 1 Blank # 2 Blank # 3arrow_forward1. Transform the E-R diagram into a set of relations. Country_of Agent ID Agent H Holds Is_Reponsible_for Consignment Number $ Value May Contain Consignment Transports Container Destination Ф R Goes Off Container Number Size Vessel Voyage Registry Vessel ID Voyage_ID Tonnagearrow_forwardI want to solve 13.2 using matlab please helparrow_forward
- a) Show a possible trace of the OSPF algorithm for computing the routing table in Router 2 forthis network.b) Show the messages used by RIP to compute routing tables.arrow_forwardusing r language to answer question 4 Question 4: Obtain a 95% standard normal bootstrap confidence interval, a 95% basic bootstrap confidence interval, and a percentile confidence interval for the ρb12 in Question 3.arrow_forwardusing r language to answer question 4. Question 4: Obtain a 95% standard normal bootstrap confidence interval, a 95% basic bootstrap confidence interval, and a percentile confidence interval for the ρb12 in Question 3.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Programming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningNew Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage Learning
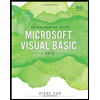
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning
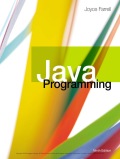
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
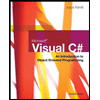
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
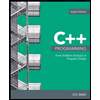
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
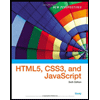
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:9781305503922
Author:Patrick M. Carey
Publisher:Cengage Learning