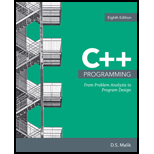
C++ PROGRAMMING:FROM...(LL) >CUSTOM<
8th Edition
ISBN: 9780357019528
Author: Malik
Publisher: CENGAGE C
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 8, Problem 6PE
Program Plan Intro
- Include necessary header files: iostream, fstream, string, and iomanip.
- Define the main() function.
- Declare the necessary variables:
- An ifstream object to read the input file.
- A string variable to hold the correct test answers.
- A string variable to hold each line of student data.
- A string variable to hold the student ID.
- A string variable to hold the student's answers to the test.
- An integer variable to hold the student's score.
- An integer variable to hold the number of questions the student didn't answer.
- An integer variable to hold the total number of questions.
- A double variable to hold the percentage score.
- A char variable to hold the student's grade.
- Open the input file using the ifstream object.
- Read the first line of the file, which contains the correct test answers, and store them in the testAnswers variable.
- Use a loop to read each subsequent line of the file:
- Extract the student ID and answers from the line using the substr() method.
- Initialize the score and notAnswered variables to 0.
- Loop through each answer and compare it to the corresponding correct answer:
- If the answer is correct, increase the score by 2.
- If the answer is incorrect, decrease the score by 1.
- If the question wasn't answered, increment the notAnswered variable.
- Calculate the percentage score and determine the student's grade based on the percentage score.
- Output the student's ID, their answers, their score, their percentage score, and their grade to the console.
- Close the input file.
- End the main() function and the program.
Program Description:
To read student answers from a text file compare them to the correct answers and calculate the students' scores and grades.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
14.
sin² 20 cos³ 20 do
15 OF 25 QUESTIONS REMAININ
Consider the following code. You want to print the array values in the div as an ordered list. What statement would you use to
replace the comment in the code below?
Two
J
// what statement goes here?
-
لبية للالكالا
const app = Vue.createApp({
data ((
return (
lunch: [
'Burrito',
'Soup',
'Pizza',
'Rice'
})
app.mount ('#app6')
-
-
Please answer JAVA OOP problem below:
Assume you have three data definition classes, Person, Student and Faculty. The Student and Faculty classes extend Person. Given the code snippet below, in Java, complete the method determinePersonTypeCount to print out how many Student and Faculty objects exist within the Person array. You may assume that each object within the Person[] is either referencing a Student or Faculty object.
public static void determinePersonTypeCount(Person[] people){
// Place your code here
}
Chapter 8 Solutions
C++ PROGRAMMING:FROM...(LL) >CUSTOM<
Ch. 8 - Mark the following statements as true or false. A...Ch. 8 - Consider the following declaration: (1,2) double...Ch. 8 - Identify error(s), if any, in the following array...Ch. 8 - Determine whether the following array declarations...Ch. 8 - Prob. 5SACh. 8 - Write C+ + statement(s) to do the following: (1,...Ch. 8 - Prob. 7SACh. 8 - Prob. 8SACh. 8 - Prob. 9SACh. 8 - Prob. 10SA
Ch. 8 - Prob. 11SACh. 8 - Correct the following code so that it correctly...Ch. 8 - Prob. 13SACh. 8 - Suppose that points is an array of 10 components...Ch. 8 - Determine whether the following array declarations...Ch. 8 - Prob. 17SACh. 8 - Prob. 19SACh. 8 - Prob. 1PECh. 8 - Prob. 2PECh. 8 - Write a C+ + function, lastLargestIndex that takes...Ch. 8 - Write a program that reads a file consisting of...Ch. 8 - Prob. 6PECh. 8 - Write a program that allows the user to enter the...Ch. 8 - Write a program that uses a two-dimensional array...Ch. 8 - Prob. 12PECh. 8 - Write a program to calculate students average test...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Please answer JAVA OOP question below: Consider the following relationship diagram between the Game and VideoGame data defintion classes. Game has a constructor that takes in two parameters, title (String) and cost (double). The VideoGame constructor has an additional parameter, genre (String). In Java, efficiently write the constructors needed within the Game class and VideoGame classes. Hint: Remember to think about the appropriate validationarrow_forwardIn a shopping cart, there are various items, which can either belong to the category of household items or electronic items. The following UML diagram illustrates the relationship between items, household items, and electronic items. //Implementation Class public class ShoppingCart{ public static void main(String[] args){ final int MAX_ITEM = 50; Item cart = new Item[MAX_ITEM]; addItem(cart); // populate the item array printItem(cart); } } Considering that all the data definition classes and the implementation class are complete, which of the following Object-Oriented Programming (OOP) concepts do you need to use in the above context? i) Polymorphism ii) Method Overloading iii) Method Overriding iv) Dynamic Binding v) Abstract Class Explain, using course terminology, how you would use any of the above concepts to model the given scenario.arrow_forwardAnswer this JAVA OOP question below: An Employee has a name, employee ID, and department. An Employee object must be created with all its attributes. The UML diagram is provided below: - name: String - employeeId: String - department: String + Employee(name: String, employeeId: String, department: String) + setName(name: String): void + setEmployeeId(employeeId: String): void + setDepartment(department: String): void + getName(): String + getEmployeeId(): String + getDepartment(): String + toString(): String A faculty is an Employee with an additional field String field: rank public class TestImplementation{ public static void main(String[] args){ Employee[] allEmployee = new Employee[100]; // create an employee object with name Tom Evan, employee ID 001 and department IST and store it in allEmployee // create a faculty object with name Adam Scott, employee ID 002, department IST and rank Professor and store it in allEmployee } }arrow_forward
- Please answer this JAVA OOP question that is given below: An Employee has a name, employee ID, and department. An Employee object must be created with all its attributes. The UML diagram is provided below: - name: String - employeeId: String - department: String + Employee(name: String, employeeId: String, department: String) + setName(name: String): void + setEmployeeId(employeeId: String): void + setDepartment(department: String): void + getName(): String + getEmployeeId(): String + getDepartment(): String + toString(): String A faculty is an Employee with an additional field String field: rank Assuming the Employee class is fully implemented, define a Professor class in Java with the following: A toString() method that includes both the inherited attributes and the specializationarrow_forwardPlease answer JAVA OOP question below: An Employee has a name, employee ID, and department. An Employee object must be created with all its attributes. The UML diagram is provided below: - name: String - employeeId: String - department: String + Employee(name: String, employeeId: String, department: String) + setName(name: String): void + setEmployeeId(employeeId: String): void + setDepartment(department: String): void + getName(): String + getEmployeeId(): String + getDepartment(): String + toString(): String A faculty is an Employee with an additional field String field: rank Assuming the Employee class is fully implemented, define a Professor class in Java with the following: Instance variable(s) A Constructorarrow_forwardDevelop a C++ program that execute the operation as stated by TM for addition of two binary numbers (see attached image). Your code should receive two binary numbers and output the resulting sum (also in binary). Make sure your code mimics the TM operations (dealing with the binary numbers as a string of characters 1 and 0, and following the logic to increase the first number and decreasing the second one. Try your TM for the following examples: 1101 and 101, resulting 10010; and 1101 and 11, resulting 10000.arrow_forward
- I need to define and discuss the uses of one monitoring or troubleshooting tool in Windows Server 2019. thank youarrow_forwardI would likr toget help with the following concepts: - Windows Server features - Windows Server versus Windows 10 used as a client-server networkarrow_forwardI need to define and discuss the uses of one monitoring or troubleshooting tool in Windows Server 2019. thank youarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageEBK JAVA PROGRAMMINGComputer ScienceISBN:9781305480537Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
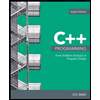
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
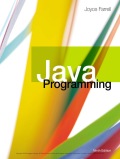
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
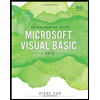
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning
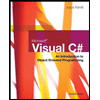
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
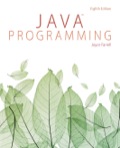
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781305480537
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Constants, Variables, Data types, Keywords in C Programming Language Tutorial; Author: LearningLad;https://www.youtube.com/watch?v=d7tdL-ZEWdE;License: Standard YouTube License, CC-BY