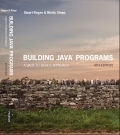
Explanation of Solution
“quadrant” method:
//definition of "Point" class
public class Point
{
//declare the required variables
int x;
int y;
//definition of "quadrant" method
public int quadrant()
{
//check "x" and "y" is greater than 0
if (x > 0 && y > 0)
{
//return "1"
return 1;
}
/*check "x" is less than 0 and "y" is greater than 0*/
else if (x < 0 && y > 0)
{
//return "2"
return 2;
}
//check "x" and "y" is less than 0
else if (x < 0 && y < 0)
{
//return "3"
return 3;
}
/*check "x" is greater than 0 and "y" is less than 0*/
else if (x > 0 && y < 0)
{
//return "4"
return 4;
}
//otherwise
else
{
//return "0"
return 0;
}
}
}
Explanation:
- In the above program, the “Point” is the class name,
- Inside the “Point” class, declare the “x” and “y” variables and the accessor method, the “quadrant” is the method name
- If the “x” and “y” are greater than 0, then return 1 to the called method.
- If the “x” is less than 0 and “y” is greater than 0, then return 2 to the called method.
- If the “x” and “y” are less than 0, then return 3 to the called method.
- If the “x” is greater than 0 and “y” is less than 0, then return 4 to the called method.
Want to see more full solutions like this?
Chapter 8 Solutions
EBK BUILDING JAVA PROGRAMS
- Is developed App in play store much easier than in app store because i look app like human anonymus and like walter labs prioritize iphone app store first is it difficult to developed app on play store ? And btw i want to move to iphone anroid suckarrow_forwardQ12- A three phase transformer 3300/400 V,has D/Y connected and working on 50Hz. The line current on the primary side is 12A and secondary has a balanced load at 0.8 lagging p.f. Determine the i) Secondary phase voltage ii) Line current iii) Output power Ans. (230.95 V, 99.11 A, 54.94 kW)arrow_forwardmake corrections of this program based on the errors shown. this is CIS 227 .arrow_forward
- Create 6 users: Don, Liz, Shamir, Jose, Kate, and Sal. Create 2 groups: marketing and research. Add Shamir, Jose, and Kate to the marketing group. Add Don, Liz, and Sal to the research group. Create a shared directory for each group. Create two files to put into each directory: spreadsheetJanuary.txt meetingNotes.txt Assign access permissions to the directories: Groups should have Read+Write access Leave owner permissions as they are “Everyone else” should not have any access Submit for grade: Screenshot of /etc/passwd contents showing your new users Screenshot of /etc/group contents showing new groups with their members Screenshot of shared directories you created with files and permissionsarrow_forward⚫ your circuit diagrams for your basic bricks, such as AND, OR, XOR gates and 1 bit multiplexers, ⚫ your circuit diagrams for your extended full adder, designed in Section 1 and ⚫ your circuit diagrams for your 8-bit arithmetical-logical unit, designed in Section 2. 1 An Extended Full Adder In this Section, we are going to design an extended full adder circuit (EFA). That EFA takes 6 one bit inputs: aj, bj, Cin, Tin, t₁ and to. Depending on the four possible combinations of values on t₁ and to, the EFA produces 3 one bit outputs: sj, Cout and rout. The EFA can be specified in principle by a truth table with 26 = 64 entries and 3 outputs. However, as the EFA ignores certain inputs in certain cases, it is easier to work with the following overview specification, depending only on t₁ and to in the first place: t₁ to Description 00 Output Relationship Ignored Inputs Addition Mode 2 Coutsjaj + bj + Cin, Tout= 0 Tin 0 1 Shift Left Mode Sj = Cin, Cout=bj, rout = 0 rin, aj 10 1 1 Shift Right…arrow_forwardShow the correct stereochemistry when needed!! mechanism: mechanism: Show the correct stereochemistry when needed!! Br NaOPh diethyl ether substitutionarrow_forward
- In javaarrow_forwardKeanPerson #keanld:int #keanEmail:String #firstName:String #lastName: String KeanAlumni -yearOfGraduation: int - employmentStatus: String + KeanPerson() + KeanPerson(keanld: int, keanEmail: String, firstName: String, lastName: String) + getKeanld(): int + getKeanEmail(): String +getFirstName(): String + getLastName(): String + setFirstName(firstName: String): void + setLastName(lastName: String): void +toString(): String +getParkingRate(): double + KeanAlumni() + KeanAlumni(keanld: int, keanEmail: String, firstName: String, lastName: String, yearOfGraduation: int, employmentStatus: String) +getYearOfGraduation(): int + setYearOfGraduation(yearOfGraduation: int): void +toString(): String +getParkingRate(): double In this question, write Java code to Create and Test the superclass: Abstract KeanPerson and a subclass of the KeanPerson: KeanAlumni. Task 1: Implement Abstract Class KeanPerson using UML (10 points) • Four data fields • Two constructors (1 default and 1 constructor with all…arrow_forwardPlz correct answer by best experts...??arrow_forward
- Q3) using the following image matrix a- b- 12345 6 7 8 9 10 11 12 13 14 15 1617181920 21 22 23 24 25 Using direct chaotic one dimension method to convert the plain text to stego text (hello ahmed)? Using direct chaotic two-dimension method to convert the plain text to stego text?arrow_forward: The Multithreaded Cook In this lab, we'll practice multithreading. Using Semaphores for synchronization, implement a multithreaded cook that performs the following recipe, with each task being contained in a single Thread: 1. Task 1: Cut onions. a. Waits for none. b. Signals Task 4 2. Task 2: Mince meat. a. Waits for none b. Signals Task 4 3. Task 3: Slice aubergines. a. Waits for none b. Signals Task 6 4. Task 4: Make sauce. a. Waits for Task 1, and 2 b. Signals Task 6 5. Task 5: Finished Bechamel. a. Waits for none b. Signals Task 7 6. Task 6: Layout the layers. a. Waits for Task 3, and 4 b. Signals Task 7 7. Task 7: Put Bechamel and Cheese. a. Waits for Task 5, and 6 b. Signals Task 9 8. Task 8: Turn on oven. a. Waits for none b. Signals Task 9 9. Task 9: Cook. a. Waits for Task 7, and 8 b. Signals none At the start of each task (once all Semaphores have been acquired), print out a string of the task you are starting, sleep for 2-11 seconds, then print out a string saying that you…arrow_forwardProgramming Problems 9.28 Assume that a system has a 32-bit virtual address with a 4-KB page size. Write a C program that is passed a virtual address (in decimal) on the command line and have it output the page number and offset for the given address. As an example, your program would run as follows: ./addresses 19986 Your program would output: The address 19986 contains: page number = 4 offset = 3602 Writing this program will require using the appropriate data type to store 32 bits. We encourage you to use unsigned data types as well. Programming Projects Contiguous Memory Allocation In Section 9.2, we presented different algorithms for contiguous memory allo- cation. This project will involve managing a contiguous region of memory of size MAX where addresses may range from 0 ... MAX - 1. Your program must respond to four different requests: 1. Request for a contiguous block of memory 2. Release of a contiguous block of memory 3. Compact unused holes of memory into one single block 4.…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
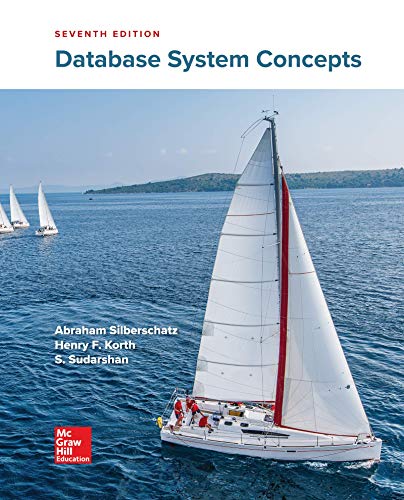
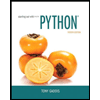
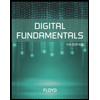
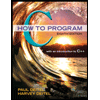
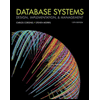
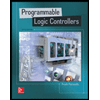