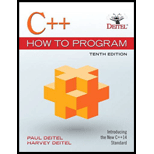
C++ How to Program (Early Objects Version)
10th Edition
ISBN: 9780134448824
Author: Paul Deitel; Harvey M. Deitel
Publisher: Pearson Education (US)
expand_more
expand_more
format_list_bulleted
Textbook Question
Chapter 7, Problem 7.17E
( What Does This Code Do?) What does the following
- // Ex. 7.17: Ex07_17.cpp
- // What does this program do?
- #include <iostream>
- #include <array>
- Using namespace std:
- Const size_t arraySize {10}:
- Inst whatIsThis (const array
&, size_t); // prototype - Int main ( ) {
- Array
a{1,2,3,4,5,6,7,8,9,10}; - Int result {whatIsThis (a, array Size) };
- Cout << “Result is “ << result << end ] ;
- }
- // What does this function do ?
- Int whatIsThis (const array <int, arraySize>& b, size_t size ) {
- If (size==1) { // base case
- Return b[0];
- }
- Else { // recursive step
- Return b[size-1] + whatIs This(b, size-1);
- }
- }
Expert Solution & Answer

Trending nowThis is a popular solution!

Students have asked these similar questions
[In c#]
Write a class with name Arrays . This class has an array which should be initialized by user.Write a method Sum that should sum even numbers in array and return sum. write a function with name numFind in this class with working logic as to find the mid number of an array. After finding this number calculate its factorial.Write function that should display sum and factorial.Don’t use divide operator
c++ :
rewrite this code , to be unique .
In which the output will stay same same.
#include <iostream>#include <stdint.h>using namespace std;
class arr{private: int size_arr; //To store the size of the array char *begin; //Pointer for dynamic allocation of memory int capacity; //To store the capacity of the elements in the arraypublic: arr(int n); //constructor (default) for creating an array int insert_arr(char x); //To insert an element x in the array int remov_arr(int i); //To remove an element from position i void display(); void displayAtPos(int i);//Function to display a character at position i};
arr::arr(int n){ size_arr = n; begin = new char[size_arr]; capacity = 0;}
int arr::insert_arr(const char x){ if(capacity >= size_arr){ cout << "Array Full!" << endl; return -1; } begin[capacity++] = x; //One element is added, so increment the…
1. a) Fill in the blanks in the code below so that, when the code runs, its output is:
1) got null pointer
2) got illegal array store
3) got illegal class cast
public class TestExceptions {
public static void main (String [ ] args) {
try {
} catch (NullPointerException e) {
System.out.println ("got null pointer");
}
try {
} catch (ArrayStoreException e) {
System.out.println ("got illegal array store");
}
Chapter 7 Solutions
C++ How to Program (Early Objects Version)
Ch. 7 - Exercises 7.6(Fill in the Blanks) Fill in the...Ch. 7 - (True or False) Determine whether each of the...Ch. 7 - (Write C++ Statements) Write C++ statements to...Ch. 7 - (Two-Dimensional array Questions) Consider a...Ch. 7 - (Salesperson Salary Ranges) Use a one-dimensional...Ch. 7 - (One-Dimensional array Questions) Write statements...Ch. 7 - (Find the Errors) Find the error(s) in each of the...Ch. 7 - (Duplicate Elimination with array) Use a...Ch. 7 - Prob. 7.14ECh. 7 - Prob. 7.15E
Ch. 7 - (Dice Rolling) Write a program that simulates...Ch. 7 - ( What Does This Code Do?) What does the following...Ch. 7 - (Craps Game Modification) Modify the program of...Ch. 7 - (Converting vector Example of Section 7.10 to...Ch. 7 - Prob. 7.20ECh. 7 - (Sales Summary) Use a two-dimensional array to...Ch. 7 - (Knight's Tour) One of the more interesting...Ch. 7 - (Knight's Tour: Brute Forty Approaches ) In...Ch. 7 - (Eight Queens) Another puzzler for chess buffs is...Ch. 7 - (Eight Queens: Brute Force Approaches) In this...Ch. 7 - Prob. 7.26ECh. 7 - (The Sieve of Eratosthenes) A prime integer is any...Ch. 7 - Prob. 7.28RECh. 7 - (Eight Queens) Modify the Eight Queens program you...Ch. 7 - (Print an array) Write a recursive function...Ch. 7 - Prob. 7.31RECh. 7 - Prob. 7.32RECh. 7 - (Maze Traversal) The grid of hashes (#) and dots...Ch. 7 - Prob. 7.34RECh. 7 - Making a Difference 7.35 (Polling) The Internet...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- // Sharissa Sullivan//January 20 2023// Chapter 9 Array Expander // C ++ #include <iostream>using namespace std;// prototype arrayExpander int* arrayExpander(int[], int size); int main(){ //create an array - orignial array int array[] = { 1,2,3, }; int size = 3; int* arrayPtr = arrayExpander(array, size); // Print out arrays for (int i = 0; i, size; i++) { cout << arrayPtr[i] << endl; } return 0;}// function to create new array int* arrayExpander(int[], int size){ // ponits to new array with * size X 2 , and copied vaules from 1st array with 0 for the values int *expanderArray = new int[size * 2]; // copy orginial array into the second array and doblue the size for (int i = 0; i < size * 2; i++) { if (i < size) { // copies the orignal array expanderArray[i] = array[i]; // LINE 35 } else { //populates the number 0, for the new vaules in…arrow_forward12. Common "The commonality between science and art is in trying to see profoundly - to develop strategies of seeing and showing." -Edward Tufte Write a Java program to find common elements between two given string arrays. Program Description Complete the main function that, given two arrays, arr1, arr2, with their sizes prints an array containing the common elements in these arrays. DO NOT USE ANY JAVA BUILT- IN ARRAY FUNCTIONS TO FIND COMMON ELEMENTS. Constraints • None Input Format For Custom Testing Sample Case 0 Sample Input For Custom Testing 5 1 1 1 1 1 3 1 2 3 Sample Output ['1'] Explanation String value '1' is the only common element between the given two arrays. Sample Case 1arrow_forwardin C++ // praphrase or change this code , while the purpose and output is same . #include <iostream>#include <stdint.h>using namespace std; class arr{private: int size_arr; //To store the size of the array char *begin; //Pointer for dynamic allocation of memory int capacity; //To store the capacity of the elements in the arraypublic: arr(int n); //constructor (default) for creating an array int insert_arr(char x); //To insert an element x in the array int remov_arr(int i); //To remove an element from position i void display(); void displayAtPos(int i);//Function to display a character at position i}; arr::arr(int n){ size_arr = n; begin = new char[size_arr]; capacity = 0;} int arr::insert_arr(const char x){ if(capacity >= size_arr){ cout << "Array Full!" << endl; return -1; } begin[capacity++] = x; //One element is added, so increment the…arrow_forward
- 6.3 Find the error in each of the following program segments. Assume int *zPtr; // zPtr will reference array z int *aPtr = NULL; void *sPtr = NULL; int number; int z[5] = {1, 2, 3, 4, 5}; sPtr = z; a) ++zptr; b) // use pointer to get first value of array; assume zPtr is initialized number = zPtr; c) // assign array element 2 (the value 3) to number; assume zPtr is initialized number = *zPtr[2]; d) // print entire array z; assume zPtr is initialized for (size_t i = 0; i <= 5; ++i) { cout << zPtr[i]; } e) // assign the value pointed to by sPtr to number number = *sPtr; f) ++z;arrow_forwardQ 1) Write a class with name Array. This class has an array which should be initialized by user. Create a function with name sumFind in this class with working logic as sum of all elements of an array, after finding sum, if sum is even calculate factorial otherwise display only sum. ( Note::: subject::c# language )arrow_forwardPsecode code explaination of the problem C++arrow_forward
- 1)Please do it in C++ languagearrow_forwardPlease Help!!!! write in c++ languagearrow_forwardI/ implement copyToVec such that: // 1) receives three parameters, an array, the array's size, and a vector // 2) copy elements from the array to the vector. Copy elements from 0 to size-1 // 3) using the RANGE-BASED for Loop ONLY, add 0.9 to every element in the vector /* implement main such that: 1) crate an array of size 100 and type float 2) prompt the user to enter 50 values 3) create a new instance of type vector that can store float values 4) invoke copyVec using this exact statement: copyToVec(arrFloat, 30, vecFloat); 5) Using the RANGE-BASED for Loop. print the values from the vector such that that the output is formatted as shown below. Use the following input test data: 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 vecFloat[0] vecFloat[1] = 1.290e+01 vecFloat[2] vecFloat[3] vecFloat[4] vecFloat[5] = 1.690e+01 vecFloat[6] = 1.790e+01 vecFloat[7] vecFloat[8] = 1.990e+01…arrow_forward
- [In C#] Write a class named Sorting with static sort function in order to sort the following array as Pakistan. Call sort function in the main function. char[] p= {‘a’, ‘p’, ‘i’, ‘k’, ‘t’, ‘a’, ‘n’, ‘s’};Output as = pakistanarrow_forwardC++arrow_forwardC++ Write a function that accepts an array and its size as arguments, creates a new array that is a copy of the argument array, and returns a pointer to the new array. Display the array in main(). The function will work as follows: Accept an array and its size as arguments. Dynamically allocate a new array that is the same size as the argument array. Copy the elements of the argument array to the new array. Return a new pointer to the new array. // This program uses a function to duplicate// an int array of any size.#include <iostream>using namespace std;// Function prototype_________________________________________________int main(){ // Define constants for the array sizes. const int SIZE1 = 5, SIZE2 = 7, SIZE3 = 10; // Define three arrays of different sizes. int array1[SIZE1] = { 100, 200, 300, 400, 500 }; int array2[SIZE2] = { 10, 20, 30, 40, 50, 60, 70 }; int array3[SIZE3] = { 1, 2, 3, 4, 5, 6, 7, 8, 9, 10 }; // Define three pointers for the duplicate arrays. int *dup1 =…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
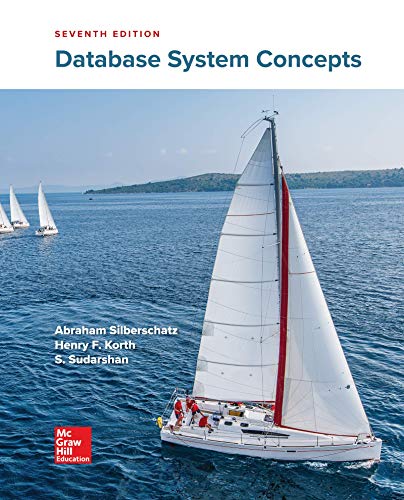
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
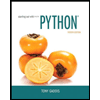
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
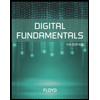
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
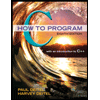
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
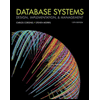
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
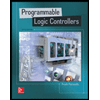
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education
1.1 Arrays in Data Structure | Declaration, Initialization, Memory representation; Author: Jenny's lectures CS/IT NET&JRF;https://www.youtube.com/watch?v=AT14lCXuMKI;License: Standard YouTube License, CC-BY
Definition of Array; Author: Neso Academy;https://www.youtube.com/watch?v=55l-aZ7_F24;License: Standard Youtube License