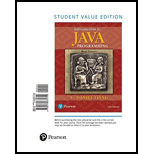
(Reverse an array) The reverse method in Section 7.7 reverses an array by copying it to a new array. Rewrite the method that reverses the array passed in the argument and returns this array. Write a test

Want to see the full answer?
Check out a sample textbook solution
Chapter 7 Solutions
Introduction to Java Programming and Data Structures: Brief Version (11th Global Edition)
Additional Engineering Textbook Solutions
Java How to Program, Early Objects (11th Edition) (Deitel: How to Program)
Elementary Surveying: An Introduction To Geomatics (15th Edition)
Management Information Systems: Managing The Digital Firm (16th Edition)
SURVEY OF OPERATING SYSTEMS
Starting Out With Visual Basic (8th Edition)
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
- (Intro to Java) Open a new Java file called ArrayMethods.java. Read the Javadoc comment for each method below and write the method according to the description in the comment Once you are getting the correct output for all tests inside of main, upload your source file to Canvas public class ArrayMethods { /** * Displays the contents of an array on the console * with each element separated by a blank space * Prints a new line character last * @param nums the array to print to the console */ public static void printArray(int[] nums) { return; } /** * Assuming an array of integers, return true if 1 * is the first element or 1 is the last element * Note: you may assume that you will be given an array * of at least length 1 * is1FirstLast([1, 2, 10]) → true * is1FirstLast([10, 1, 2, 1]) → true * is1FirstLast([13, 10, 1, 2, 3]) → false * @param numbers the array of int numbers * @return whether or not 1 is the first or…arrow_forward(Java 4 intellij) Part1: Read an integer input from the user and save it to a variable 'size'. - Use the variable 'size' to initialize an array of length size - Next read in integer inputs from the user and save each incoming integer to the next available position in your array. - Continue until your array is full. - Note: You will need to conditionally check if you have received the number of inputs you need from the user to fill the array. Print the contents of the full array. Part 2: Create a method which generates and prints “n” random numbers. The method takes an int parameter indicating how many random numbers to print. Use a loop. arrow_forward(Intro to Java) Explain the answers to the below questions. include a written answer to the question using step-by-step explanation 4. Write the linearSearch method as follows using the algorithm provided in class: The method is named linearSearch It takes on array of doubles as a parameter It takes a double value to locate in the array as a second parameter It returns the integer location (index) in the array where the value is located Or, it returns -1 if the value cannot be foundarrow_forward
- Question 2 : (Reverse an array) Write a program Reverse.java that prompts the user to enter ten numbers, reverses an array by copying it to a new array and displays the numbers. The array is an attribute of the main class. Enter 10 elements of T ... 1 2 3 4 5 6 7 8 9 10 10 9 8 7 6 5 4 3 2 1arrow_forward(Same-number subsequence) JAVA Class Name: Exercise22_05 Write an O(n) program that prompts the user to enter a sequence of integers ending with 0 and finds the longest subsequence with the same number. Sample Run 1 Enter a series of numbers ending with 0:2 4 4 8 8 8 8 2 4 4 0The longest same number sequence starts at index 3 with 4 values of 8 Sample Run 2 Enter a series of numbers ending with 0: 34 4 5 4 3 5 5 3 2 0 The longest same number sequence starts at index 5 with 2 values of 5arrow_forward1.Answer the following questions: 1(a) Write a method to compute and return the value of maxEven and minOdd where maxEven is the largest even element of an int array and minOdd is the smallest odd element of the int array. The method is passed the int array and an int value that denotes the number of elements in the array. You may assume that the int array has at least 1 element in it. 1(b) Write a method to compute and return the value of countEven and countOdd where countEven is the number even elements in an int array and countOdd is the number of odd elements in the int array. The method is passed the int array and an int value that denotes the number of elements in the array. You may assume that the int array has at least 1 element in it.arrow_forward
- (Write in java) Declare an array that represents 100 students. Assign twodifferent questions between 1 and 20 randomly to eachstudent. Display the students’ questions for each studentarrow_forwardUsing Java: (Sum the areas of geometric objects) Write a method that sums the areas of all thegeometric objects in an array. The method signature is:public static double sumArea(GeometricObject[] a) Write a test program that creates an array of four objects (two circles and tworectangles) and computes their total area using the sumArea methodarrow_forward(Convert decimals to fractions) Write a program that prompts the user to enter a decimal number and displays the number in a fraction. Hint: read the decimal number as a string, extract the integer part and fractional part from the string, and use the Rational class to obtain a rational number for the decimal number. Sample Run 1Enter a decimal number: 3.25 The fraction number is 13/4 Sample Run 2Enter a decimal number: -0.45452 The fraction number is -11363/25000arrow_forward
- (Sort three numbers) Write the following function to display three numbers in increasing order: def displaySortedNumbers(num1, num2, num3): Write a test program that prompts the user to enter three numbers and invokes the function to display them in increasing order. Here are some sample runs:arrow_forwardPlease help me with this , I am stuck ! thanks 1) bagUnion: The union of two bags is a new bag containing the combined contents of the original two bags. Design and specify a method union for the ArrayBag that returns as a new bag the union of the bag receiving the call to the method and the bag that is the method's parameter. The method signature (which should appear in the .h file and be implemented in the .cpp file is: ArrayBag<T> bagUnion(const ArrayBag<T> &otherBag) const; This method would be called in main() with: ArrayBag<int> bag1u2 = bag1.bagUnion(bag2); Note that the union of two bags might contain duplicate items. For example, if object x occurs five times in one bag and twice in another, the union of these bags contains x seven times. The union does not affect the contents of the original bags. Here is the all of the file: ArrayBag.cpp: #include <string>#include "ArrayBag.h" template <class T>ArrayBag<T>::ArrayBag() :…arrow_forward.arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
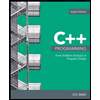