C Programming: From Problem Analysis to Program Design
8th Edition
ISBN: 9780357238547
Author: D. S. Malik
Publisher: Cengage Limited
expand_more
expand_more
format_list_bulleted
Question
Chapter 6, Problem 18SA
(a)
Program Plan Intro
To determine the possible output, when the function call mystery(4, -5) will be generated.
(b)
Program Plan Intro
To determine the possible output, when the function call mystery(-8, 9) will be generated.
(c)
Program Plan Intro
To determine the possible output, when the function call mystery(2,3) will be generated.
(d)
Program Plan Intro
To determine the possible output, when the function call mystery(-2,-4) will be generated.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Consider the following C++ function. int mystery(int num1, int num2){if (num1 > 0){for (int i = 1; i <= num1; i++)num2 = num2 * i;return num2;}else if (num2 > 0){for (int i = 0; i <= num2; i++)num1 = num1 + i;return num1;}return 0;}What is the output of the following statements?a. cout << mystery(4, -5) << endl;b. cout << mystery(-8, 9) << endl;c. cout << mystery(2, 3) << endl;d. cout << mystery(-2, -4) << endl;
C Program:
An integer number is said to be a perfect number if its factors, including 1 (but not the number itself), sum to the number. For example, 6 is a perfect number because 6 = 1 + 2 + 3. I have written a function called isPerfect (see below), that determines whether parameter passed to the function is a perfect number. Use this function in a C program that determines and prints all the perfect numbers between 1 and 1000. Print the factors of each perfect number to confirm that the number is indeed perfect.
// isPerfect returns true if value is perfect integer,
// i.e., if value is equal to sum of its factors
int isPerfect(int value)
{
int factorSum = 1; // current sum of factors
// loop through possible factor values
for (int i = 2; i <= value / 2; ++i) {
// if i is factor
if (value % i == 0) {
factorSum += i; // add to sum
}
}
// return true if value is equal to sum of factors
if (factorSum == value) {
return…
Write the reccurence relation for the following function (in C-style code)
void T(n){
if(n>1){
int j;
for(j = n; j>1; j = j/2);
T(n/2); T(n/2);
}
}
Chapter 6 Solutions
C Programming: From Problem Analysis to Program Design
Ch. 6 - Mark the following statements as true or false:
a....Ch. 6 - Determine the value of each of the following...Ch. 6 - Determine the value of each of the following...Ch. 6 - Consider the following function definition. (4, 6)...Ch. 6 - Consider the following statements:
Which of the...Ch. 6 - Prob. 8SACh. 6 - Prob. 9SACh. 6 - Why do you need to include function prototypes in...Ch. 6 - Prob. 11SACh. 6 - Consider the following function: (4)...
Ch. 6 - Prob. 15SACh. 6 - What is the output of the following program? (4)
Ch. 6 - Write the definition of a function that takes as...Ch. 6 - Prob. 18SACh. 6 - How would you use a return statement in a void...Ch. 6 - Prob. 20SACh. 6 - Prob. 21SACh. 6 - What is the output of the following program?...Ch. 6 - Write the definition of a void function that takes...Ch. 6 - Write the definition of a void function that takes...Ch. 6 - Prob. 8PECh. 6 - The following formula gives the distance between...Ch. 6 - Write a program that takes as input five numbers...Ch. 6 - When you borrow money to buy a house, a car, or...Ch. 6 - Consider the definition of the function main:...Ch. 6 - The statements in the following program are not in...Ch. 6 - Write a program that outputs inflation rates for...Ch. 6 - Write a program to convert the time from 24-hour...Ch. 6 - Jason opened a coffee shop at the beach and sells...
Knowledge Booster
Similar questions
- C PROGRAM Reverse + Random Formula In the code editor, there's already an initial code that contains the function declaration of the function, computeReverseNumber(int n) and an empty main() function. For this problem, you are task to implement the computeReverseNumber function. This function will take in an integer n as its parameter. This will get the reverse of the passed integer and will then compute and return the value: result = (original_number + reverse_of_the_number) / 3 In the main() function, ask the user to input the value of n, call the function computeReverseNumber and pass the inputted value of n, and print the result in the main() with two (2) decimal places. SAMPLE: Input n: 20 Output: 7.33 Input n: 123 Output: 148.00arrow_forward*In C program, what is wrong with the following function? int integer_add( int x, int y, int z ) { int sum; sum = x + y + z: return sum; }arrow_forwardC++arrow_forward
- In C programming, if the return type of a function is Void how can you implement assert statements? For example, Void ceasar(int n, char*x); //if char = c and n = 3 output == f how do i use assert statements as this does not work assert (caesar(3,c)==f);arrow_forwardfloat theRealQuestion (int x, float y) { int z; if (x > 15) z = x; else z = y; return z; } Code the function prototype for the given function.arrow_forwardimplement pass-by-value parameter passing method.USE C LANGUAGEarrow_forward
- Consider the function void change(int *p) { *p = 20; }Show how to call the change function so that it sets the integer variable int i;to 20.arrow_forwardWrite a C++ function weird_sum that takes two integer parameters, n1 and n2. The function returns the sum of n1 and n2, as long as their sum is not a multiple of 13. If their sum is a multiple of 13, the function returns 3 less than the sum of n1 and n2.arrow_forwardProgramming Language :- C 7. A function is defined for a positive integer n as follows: 3n + 1, if n is odd f(n) = { n if n is even 2 We consider the repeated application of the function starting with a given integer n, as follows: f(n), f(f(n)), f(f(f(n))), It is conjectured that no matter which positive integer n you start from; this sequence eventually will reach to 1. If n = 13, the sequence will be 13, 40, 20, 10, 5, 16, 8, 4, 2, 1. Thus if you start from n = 13, you need to apply function 10 times to reach 1. Write a recursive function that takes n as an input number and returns how many times function f has to be applied repeatedly to reach 1.arrow_forward
- write code in c++ pleasearrow_forwardINSTRUCTIONS: Write a C++ script/code to do the given problems. MOVIE PROBLEM: Write a function that checks whether a person can watch an R18+ rated movie. One of the following two conditions is required for admittance: The person is atleast 18 years old. • They have parental supervision. The function accepts two parameters, age and isSupervised. Return a boolean. Example: acceptIntoMovie(14, true) → true acceptIntoMovie(14, false) → falsearrow_forwardC++ Write a function for the function prototype int triple(int);. The function should return the cube of the parameter. int triple(int); // prototypearrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
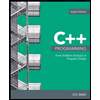
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
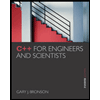
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr