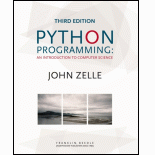
Draw a face
Program plan:
- Import the header file.
- Define the “drawFace” method
- Get the “x” and “y” positions
- Get the “p1” and “p2” positions
- Call the “Oval” method for drawing the face
- Set the color to the face
- Draw the face
- Get the “lc” and “rc” position
- Call the “Circle” method
- Set the color to the face
- Call the “draw” method
- Call the “Circle” method
- Set the color to the face
- Call the “draw” method
- Get the “m1”, “m2”, and “m3” values
- Call the “Rectangle” method
- Set the color to the face
- Call the “draw” method
- Call the “Line” method
- Get the center position
- Get the “x” and “y” position
- Call the “Point” method
- Call the “Line” method
- Call the “draw” method
- Get the “x” and “y” position for different variables
- Calculate “t” and “t1” value
- Call the “Rectangle” method
- Call the “draw” method
- Iterate “i” until it reaches 7
- Clone the teeth by calling “clone” method
- Move the face by calling “move” method
- Call the “draw” method
- Define the main method.
- Read the filename from the user
- Get the image width and height from the file
- Set the graph win size
- Set the coords for graph
- Draw the face
- Get the number of faces from the user
- Iterate “i” until it reaches “n” value
- Get the mouse action
- Call the “drawFace” method
- Call the function “main()”.

This Python program is used to get the GIF (Graphic Interchange format) file and draw the smileys when the user clicking the GIF image.
Explanation of Solution
Program:
#import the header file
from graphics import *
#definition of "drawFace" method
def drawFace(center, size, window):
#get the "x" value
x1 = center.getX()
#get the "y" value
y1 = center.getY()
#get the "p1" value
p1 = Point(x1-(.7 * size), y1 - size)
#get the "p2" value
p2 = Point(x1+(.7 * size), y1 + size)
#call the "Oval" method
head = Oval(p1, p2)
#fill the color
head.setFill("white")
#call the "draw" method
head.draw(window)
#get the "lc" value
lc = Point(x1 - .2 * size, y1 + .6 * size)
#get the "rc" value
rc = Point(x1 + .2 * size, y1 + .6 * size)
#call the "Circle" method
leftEye = Circle(lc, .13 * size)
#fill the color
leftEye.setFill("white")
#call the "draw" method
leftEye.draw(window)
#call the "Circle" method
rightEye = Circle(rc, .13 * size)
#fill the color
rightEye.setFill("white")
#call the "draw" method
rightEye.draw(window)
#get the "m1" value
m1 = Point(x1 - .3 * size, y1 - .5 * size)
#get the "m2" value
m2 = Point(x1 + .3 * size, y1 - .25 * size)
#get the "m3" value
m3 = Point(x1 + .3 * size, y1 - .5 * size)
#call the "Rectangle" method
mouth = Rectangle(m1, m2)
#fill the color
mouth.setFill("white")
#call the "draw" method
mouth.draw(window)
#call the "Line" method
leftLip = Line(m1, Point(x1- .3 * size, y1 - .25 * size))
#get the center position
mLeftCent = leftLip.getCenter()
#get the "x" position
mLCx = mLeftCent.getX()
#get the "y" position
mLCy = mLeftCent.getY()
#call the "Point" method
mRightCent = Point(x1 + .3 * size, mLCy)
#call the "Line" method
lip = Line(mLeftCent, mRightCent)
#call the "draw" method
lip.draw(window)
#get the "x" positions
m1x = m1.getX()
m3x = m3.getX()
#get the "y" positions
m1y = m1.getY()
m2y = m2.getY()
#calculate the "t" value
t = m1x - m3x
#calculate the "t1" value
t1 = Point(m1x - (1/8 * t), m2y)
#call the "Rectangle" method
teeth = Rectangle(m1, t1)
#call the "draw" method
teeth.draw(window)
#iterate "i" until it reaches 7
for i in range (7):
#clone the face
t2 = teeth.clone()
#call the "move" method
t2.move(-i * (1/8 * t), 0)
#call the "draw" method
t2.draw(window)
#definition of main method
def main():
#get the input filename from the user
fname = input("Enter filename: ")
infile = Image(Point(10, 10), fname)
#get the width of the image
wWidth = infile.getWidth()
#get the height of the image
wHeight = infile.getHeight()
#set graph win name
window = GraphWin('Smile!', wWidth,wHeight)
#set the coords
window.setCoords(0, 0, 20, 20)
#draw a smiley
infile.draw(window)
#get how many faces the user wants to draw
n = eval(input("How many faces should we block? "))
#iterate "i" until it reaches "n"
for i in range(n):
#get the mouse action
point = window.getMouse()
#draw a face by calling the method "drawFace"
drawFace(point, 3, window)
#call the "main" method
main()
Output:
Enter filename: test_img.gif
How many faces should we block? 2
>>>
Screenshot of “Smile!” window
Want to see more full solutions like this?
Chapter 6 Solutions
Python Programming: An Introduction to Computer Science
- how to read log logsarrow_forwardDiscrete Mathematics for Computer Engineeringarrow_forwardQuestion 1 - Array Iterators Like the JS on A2, there is no visual component to this question. The HTML really just needs to load the JavaScript, everything else will output to the console. The JS file should the completion of the task, and all necessary testing, so that just loading the file will complete the task with enough different inputs to ensure it works. Even Numbers [3 marks] Create a function that determines if a provided number is even. Define an array of numbers, then on the array use the appropriate array iterator to determine if the array contains only even numbers using the function you defined. Output the results, and test with several arrays. Long Names [3 marks] Define an array of names. Use an iterator to retrieve a new array containing only the names longer then 12 characters. Your iterator should be passed an anonymous arrow function. Test with several different arrays First Names [3 marks] Define an array called fullNames that contains 7 javascript objects of…arrow_forward
- Discrete Mathematics for Computer Engineeringarrow_forwardthis module is java 731 . make sure my answers are 1005 correct and the layout and structure is perfect and also include all comments etc. thank you i have attached question 1 (40 marks) and question 2 (30 marks ) this is question 3: Question3: (30 MARKS) Passenger Rail Agency for South Africa Train Scheduling System Problem Statement Design and implement a train scheduling system for Prasa railway network. The system should handle the following functionalities: 1. Scheduling trains: Allow the addition of train schedules, ensuring that no two trains use the same platform at the same time at any station. 2. Dynamic updates: Enable adding new train schedules and canceling existing ones. 3. Real-time simulation: Use multithreading to simulate the operation of trains (e.g., arriving, departing). 4. Data management: Use ArrayList to manage train schedules and…arrow_forwardthis module is java 731 . make sure my answers are 1005 correct and the layout and structure is perfect and also include all comments etc. thank you i have attached question 1 (40 marks) and question 2 (30 marks ) this is question 3: Question3: (30 MARKS) Passenger Rail Agency for South Africa Train Scheduling System Problem Statement Design and implement a train scheduling system for Prasa railway network. The system should handle the following functionalities: 1. Scheduling trains: Allow the addition of train schedules, ensuring that no two trains use the same platform at the same time at any station. 2. Dynamic updates: Enable adding new train schedules and canceling existing ones. 3. Real-time simulation: Use multithreading to simulate the operation of trains (e.g., arriving, departing). 4. Data management: Use ArrayList to manage train schedules and…arrow_forward
- this module is java 731 . make sure my answers are 1005 correct and the layout and structure is perfect and also include all comments etc. thank you i have attached question 1 (40 marks) and question 2 (30 marks ) this is question 3: Question3: (30 MARKS) Passenger Rail Agency for South Africa Train Scheduling System Problem Statement Design and implement a train scheduling system for Prasa railway network. The system should handle the following functionalities: 1. Scheduling trains: Allow the addition of train schedules, ensuring that no two trains use the same platform at the same time at any station. 2. Dynamic updates: Enable adding new train schedules and canceling existing ones. 3. Real-time simulation: Use multithreading to simulate the operation of trains (e.g., arriving, departing). 4. Data management: Use ArrayList to manage train schedules and…arrow_forwardDiscrete Mathematics for Computer Engineeringarrow_forwardTask 1: Write an abstract class Method +: public -: private #: protected Underline: static # input: int # output:String Method + isHard():boolean + specificWay():String + Method() + Method(input: int, output: String) + getInput(): int + setInput(input: int): void + getOutput(): String + setOutput(output: String): void +toString(): String Question Task 2: Write a class ReadMethod that extends the Method class. +: public -: private #: protected Underline: static -language: String ReadMethod Question + ReadMethod() + ReadMethod(input: int, output: String, language: String) + isHard():boolean + specific Way(): String +toString(): String + getLanguage(): String + setLanguage(language: String): voidarrow_forward
- i have attatched my java question , please make sure it is answered correct, include all comments etc, thank youarrow_forwardi have attached my 2 java questions . please answer them correctly, add all comments etc . thank you.arrow_forwardCan you help me solve this problem using Master's Theorem:Solve the recurrence relation f(n) = 3af(n/a) + (n + a)2 with f(1) = 1 and a > 1 byfinding an expression for f(n) in big-Oh notation.arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
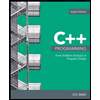
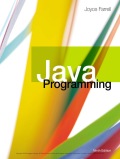
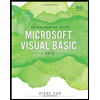
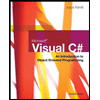
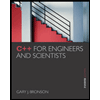