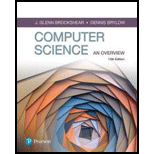
Modify the sequential search function in Figure 5.6 to allow for lists that are not sorted.
def Search(List, TargetValue):
if (List is empty):
Declare search a failure.
else:
Select the first entry in List to be TestEntry.
while (TargetValue > TestEntry and
there remain entries to be considered):
Select the next entry in List as TestEntry.
if (TargetValue = TestEntry):
Declare search a success,
else:
Declare search a failure.

Want to see the full answer?
Check out a sample textbook solution
Chapter 5 Solutions
Computer Science: An Overview (13th Edition) (What's New in Computer Science)
Additional Engineering Textbook Solutions
Degarmo's Materials And Processes In Manufacturing
Java How to Program, Early Objects (11th Edition) (Deitel: How to Program)
SURVEY OF OPERATING SYSTEMS
Starting Out With Visual Basic (8th Edition)
Mechanics of Materials (10th Edition)
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
- Note : It is required to done this by oop in C++. Create a queue, size of queue will be dependent on the user. Insert the numbers in the queue till the queue reaches the size. Create a menu and perform the following function on that queue. Enqueue: Add an element to the end of the queue Dequeue: Remove an element from the front of the queue IsEmpty: Check if the queue is empty IsFull: Check if the queue is full Peek: Get the value of the front of the queue without removing itarrow_forwardCreate a queue, size of queue will be dependent on the user. Insert the numbers in the queue till the queue reaches the size. Create a menu and perform the following function on that queue. This is all done by using oop in C++. Enqueue: Add an element to the end of the queue Dequeue: Remove an element from the front of the queue IsEmpty: Check if the queue is empty IsFull: Check if the queue is full Peek: Get the value of the front of the queue without removing itarrow_forwardOld MathJax webview Old MathJax webview In Java Some methods of the singly linked list listed below can be implemented efficiently (in different respects) (as opposed to an array or a doubly linked list), others not necessarily which are they and why? b. Implement a function to add an element before the first element. c. Implement a function to add an item after the last one element. d. Implement a function to output an element of the list. e. Implement a function to output the entire list. f. Implement a function to output the number of elements. G. Implement a function to delete an item. H. Implement a function to clear the entire list. I. Implement functionality to search for one or more students by first name, last name, matriculation number or course of study. J. Implement functionality to sort the records of the student, matriculation number and course according to two self-selected sorting methods.arrow_forward
- Complete question bcoding:-.arrow_forwardDouble trouble def double_trouble(items, n): Suppose, if just for the sake of argument, that the following operation is repeated n times for the given list of items: remove the first element, and append that same element twice to the end of items. Which one of the items would be removed and copied in the last operation performed? Sure, this problem could be finger-quotes “solved” by actually performing that operation n times, but the point of this exercise is to come up with an analytical solution to compute the result much faster than actually going through that whole rigmarole. To gently nudge you towards thinking in symbolic and analytical solutions, the automated tester is designed so that anybody trying to brute force their way through this problem by performing all n operations one by one for real will run out of time and memory long before receiving the answer, as will the entire universe. To come up with this analytical solution, tabulate some small cases (you can implement the…arrow_forwardDouble trouble def double_trouble(items, n): Suppose, if just for the sake of argument, that the following operation is repeated n times for the given list of items: remove the first element, and append that same element twice to the end of items. Which one of the items would be removed and copied in the last operation performed?Sure, this problem could be finger-quotes “solved” by actually performing that operation n times, but the point of this exercise is to come up with an analytical solution to compute the result much faster than actually going through that whole rigmarole. To gently nudge you towards thinking in symbolic and analytical solutions, the automated tester is designed so that anybody trying to brute force their way through this problem by performing all n operations one by one for real will run out of time and memory long before receiving the answer, as will the entire universe.To come up with this analytical solution, tabulate some small cases (you can implement the…arrow_forward
- Write the following function that merges two sorted lists into a new sorted list:def merge(list1, list2): Implement the function in a way that takes len(list1) + len(list2) comparisons. Write a test program that prompts the user to enter two sorted lists and displays the merged list.arrow_forwardThe following function is designed to take in a nested list structure of unknown depth, where each element at every level is either an integer or a list. The function should return a copy of the nested list structure, but with all integer values increased by 1. Fill in the blanks in the function such that it works as intended. def deep_add(collection): if isinstance (collection, int): #Checks if collection is an int return elif collection return else: == []: return [deep_add(collection [0])] +arrow_forwardDictionary Walk Program Using Java, write a program which takes two words as inputs and walks through the dictionary and creates a list of words between them. Two words are “adjacent” if you can change one word into the other by adding, deleting, or changing a single letter. A “word list” is an ordered list of unique words where successive words are adjacent. Use the official Scrabble word list as your dictionary of valid words. Examples: hate → love: hate, have, hove, love dogs → wolves: dogs, does, doles, soles, solves, wolves man → woman: man, ran, roan, roman, woman flour → flower: flour, lour, dour, doer, dower, lower, flower Questions What is the shortest list between “crawl” and “run”? What is the shortest list between “mouse” and “elephant”? Does your program necessarily return the shortest list? What assumptions did you make in your program? How did you test your program? What is the Big-O complexity of your program? Additional Questions, answer three Suppose…arrow_forward
- Note: Code in c++ Consider the following statements: unorderedLinkedList myList; unorderedLinkedList subList; Suppose myList points to the list with elements 34 65 27 89 12 (in this order). The statement: myList.divideMid(subList); divides myList into two sublists: myList points to the list with the elements 34 65 27, and subList points to the sublist with the elements 89 12.arrow_forwardTrue/False Select true or false for the statements below. Explain your answers if you like to receive partial credit. 3) Which of the following is true about the insertBeforeCurrent function for a CircularLinked List (CLL) like you did in programming exercise 1?a. If the CLL is empty, you need to create the new node, set it to current, andhave its next pointer refer to itselfb. The worst case performance of the function is O(n)c. If you insert a new element with the same data value as the current node, theperformance improves to O(log n)arrow_forwardfor c++ please thank you please type the code so i can copy and paste easily thanks. 4, List search Modify the linked listv class you created in the previous programming challange to include a member function named search that returns the position of a specific value, x, in the lined list. the first node in the list is at position 0, the second node is at position 1, and so on. if x is not found on the list, the search should return -1 test the new member function using an approprate driver program. here is my previous programming challange. #include <iostream> using namespace std; struct node { int data; node *next; }; class list { private: node *head,*tail; public: list() { head = NULL; tail = NULL; } ~list() { } void append() { int value; cout<<"Enter the value to append: "; cin>>value; node *temp=new node; node *s; temp->data = value; temp->next = NULL; s = head; if(head==NULL) head=temp; else { while (s->next != NULL) s = s->next; s->next =…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
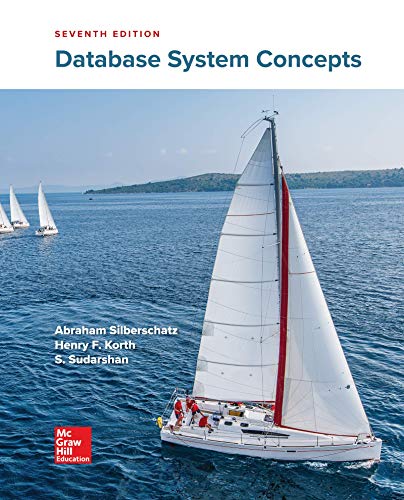
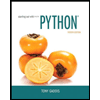
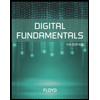
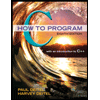
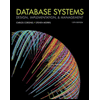
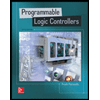