Introduction To Algorithms, Third Edition (international Edition)
3rd Edition
ISBN: 9780262533058
Author: Thomas H. Cormen, Charles E. Leiserson, Ronald L. Rivest, Clifford Stein
Publisher: TRILITERAL
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 31.3, Problem 4E
Program Plan Intro
To prove the expression.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
3.) File Encryption and DecryptionWrite a program that uses a dictionary to assign “codes” to each letter of the alphabet. For example:
codes = { ‘A’ : ‘%’, ‘a’ : ‘9’, ‘B’ : ‘@’, ‘b’ : ‘#’, etc . . .}Using this example, the letter A would be assigned the symbol %, the letter a would be assigned the number 9, the letter B would be assigned the symbol @, and so forth.
The program should open a specified text file, read its contents, then use the dictionary to write an encrypted version of the file’s contents to a second file. Each character in the second file should contain the code for the corresponding character in the first file.
Write a second program that opens an encrypted file and displays its decrypted contents on the screen.
Returns an US standard formatted phone number, in the format of
(xxx) xxx-xxxx
the AreaCode, Prefix and number being each part in order.
Testing Hint: We be exact on the format of the number when
testing this method. Make sure you think about how to convert
33 to 033 or numbers like that when setting your string format. Reminder
the %02d - requires the length to be 2, with 0 padding at the front if a single
digit number is passed in.
The next problem concerns the following C code:
/copy input string x to buf */
void foo (char *x) {
char buf [8];
strcpy((char *) buf, x);
}
void callfoo() {
}
foo("ZYXWVUTSRQPONMLKJIHGFEDCBA");
Here is the corresponding machine code on a Linux/x86 machine:
0000000000400530 :
400530:
48 83 ec 18
sub
$0x18,%rsp
400534:
48 89 fe
mov
%rdi, %rsi
400537:
48 89 e7
mov
%rsp,%rdi
40053a:
e8 d1 fe ff ff
40053f:
48 83 c4 18
add
callq 400410
$0x18,%rsp
400543:
c3
retq
0000000000400544 :
400544:
48 83 ec 08
sub
$0x8,%rsp
400548:
bf 00 06 40 00
mov
$0x400600,%edi
40054d:
e8 de ff ff ff
callq 400530
400552:
48 83 c4 08
add
$0x8,%rsp
400556:
c3
This problem tests your understanding of the program stack. Here are some notes to
help you work the problem:
• strcpy(char *dst, char *src) copies the string at address src (including
the terminating '\0' character) to address dst. It does not check the size of
the destination buffer.
You will need to know the hex values of the following characters:
Chapter 31 Solutions
Introduction To Algorithms, Third Edition (international Edition)
Ch. 31.1 - Prob. 1ECh. 31.1 - Prob. 2ECh. 31.1 - Prob. 3ECh. 31.1 - Prob. 4ECh. 31.1 - Prob. 5ECh. 31.1 - Prob. 6ECh. 31.1 - Prob. 7ECh. 31.1 - Prob. 8ECh. 31.1 - Prob. 9ECh. 31.1 - Prob. 10E
Ch. 31.1 - Prob. 11ECh. 31.1 - Prob. 12ECh. 31.1 - Prob. 13ECh. 31.2 - Prob. 1ECh. 31.2 - Prob. 2ECh. 31.2 - Prob. 3ECh. 31.2 - Prob. 4ECh. 31.2 - Prob. 5ECh. 31.2 - Prob. 6ECh. 31.2 - Prob. 7ECh. 31.2 - Prob. 8ECh. 31.2 - Prob. 9ECh. 31.3 - Prob. 1ECh. 31.3 - Prob. 2ECh. 31.3 - Prob. 3ECh. 31.3 - Prob. 4ECh. 31.3 - Prob. 5ECh. 31.4 - Prob. 1ECh. 31.4 - Prob. 2ECh. 31.4 - Prob. 3ECh. 31.4 - Prob. 4ECh. 31.5 - Prob. 1ECh. 31.5 - Prob. 2ECh. 31.5 - Prob. 3ECh. 31.5 - Prob. 4ECh. 31.6 - Prob. 1ECh. 31.6 - Prob. 2ECh. 31.6 - Prob. 3ECh. 31.7 - Prob. 1ECh. 31.7 - Prob. 2ECh. 31.7 - Prob. 3ECh. 31.8 - Prob. 1ECh. 31.8 - Prob. 2ECh. 31.8 - Prob. 3ECh. 31.9 - Prob. 1ECh. 31.9 - Prob. 2ECh. 31.9 - Prob. 3ECh. 31.9 - Prob. 4ECh. 31 - Prob. 1PCh. 31 - Prob. 2PCh. 31 - Prob. 3PCh. 31 - Prob. 4P
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- A ROP (Return-Oriented Programming) attack can be used to execute arbitrary instructions by chaining together small pieces of code called "gadgets." Your goal is to create a stack layout for a ROP attack that calls a function located at '0x4018bd3'. Below is the assembly code for the function 'getbuf', which allocates 8 bytes of stack space for a 'char' array. This array is then passed to the 'gets' function. Additionally, you are provided with five useful gadgets and their addresses. Use these gadgets to construct the stack layout. Assembly for getbuf 1 getbuf: 2 sub $8, %rsp 3 mov %rsp, %rdi 4 call gets 56 add $8, %rsp ret #Allocate 8 bytes for buffer #Load buffer address into %rdi #Call gets with buffer #Restore the stack pointer #Return to caller. Stack Layout (fill in Gadgets each 8-byte section) Address Gadget Address Value (8 bytes) 0x4006a7 pop %rdi; ret 0x7fffffffdfc0 Ox4006a9 pop %rsi; ret 0x7fffffffdfb8 0x4006ab pop %rax; ret 0x7fffffffdfb0 0x7fffffffdfa8 Ox4006ad mov %rax,…arrow_forwardIn each of the following C code snippets, there are issues that can prevent the compilerfrom applying certain optimizations. For each snippet:• Circle the line number that contains compiler optimization blocker.• Select the best modification to improve optimization.1. Which line prevents compiler optimization? Circle one: 2 3 4 5 6Suggested solution:• Remove printf or move it outside the loop.• Remove the loop.• Replace arr[i] with a constant value.1 int sum( int ∗ ar r , int n) {2 int s = 0 ;3 for ( int i = 0 ; i < n ; i++) {4 s += a r r [ i ] ;5 p r i n t f ( ”%d\n” , s ) ;6 }7 return s ;8 }2. Which line prevents compiler optimization? Circle one: 2 3 4 5 6Suggested solution:• Move or eliminate do extra work() if it’s not necessary inside the loop.• Remove the loop (but what about scaling?).• Replace arr[i] *= factor; with arr[i] = 0; (why would that help?).1 void s c a l e ( int ∗ ar r , int n , int f a c t o r ) {2 for ( int i = 0 ; i < n ; i++) {3 a r r [ i ] ∗= f a c t o r…arrow_forward123456 A ROP (Return-Oriented Programming) attack can be used to execute arbitrary instructions by chaining together small pieces of code called "gadgets." Your goal is to create a stack layout for a ROP attack that calls a function located at 'Ox4018bd3'. Below is the assembly code for the function 'getbuf, which allocates 8 bytes of stack space for a 'char' array. This array is then passed to the 'gets' function. Additionally, you are provided with five useful gadgets and their addresses. Use these gadgets to construct the stack layout. Assembly for getbuf 1 getbuf: sub mov $8, %rsp %rsp, %rdi call gets add $8, %rsp 6 ret #Allocate 8 bytes for buffer #Load buffer address into %rdi #Call gets with buffer #Restore the stack pointer #Return to caller Stack each Layout (fill in Gadgets 8-byte section) Address Gadget Address Value (8 bytes) 0x7fffffffdfc0 0x7fffffffdfb8 0x7fffffffdfb0 0x7fffffffdfa8 0x7fffffffdfa0 0x7fffffffdf98 0x7fffffffdf90 0x7fffffffdf88 Original 0x4006a7 pop %rdi;…arrow_forward
- Character Hex value || Character Hex value | Character Hex value 'A' 0x41 יני Ox4a 'S' 0x53 0x42 'K' 0x4b 'T" 0x54 0x43 'L' Ox4c 0x55 0x44 'M' Ox4d 0x56 0x45 'N' Ox4e 'W' 0x57 0x46 Ox4f 'X' 0x58 0x47 'P' 0x50 'Y' 0x59 'H' 0x48 'Q' 0x51 'Z' Охба 'T' 0x49 'R' 0x52 '\0' 0x00 Now consider what happens on a Linux/x86 machine when callfoo calls foo with the input string "ZYXWVUTSRQPONMLKJIHGFEDCBA". A. On the left draw the state of the stack just before the execution of the instruction at address Ox40053a; make sure to show the frames for callfoo and foo and the exact return address, in Hex at the bottom of the callfoo frame. Then, on the right, draw the state of the stack just after the instruction got executed; make sure to show where the string "ZYXWVUTSRQPONMLKJIHGFEDCBA" is placed and what part, if any, of the above return address has been overwritten. B. Immediately after the ret instruction at address 0x400543 executes, what is the value of the program counter register %rip? (That is…arrow_forwardDraw out the way each of these structs looks in memory, including padding! Number the offsets in memory. 1 struct okay Name 2 { short a; 3 4 long number; 5 int also_a_number; 6 7 }; char* text; 1 struct badName 2 { 3 4 5 }; short s; struct okay Name n;arrow_forwardYou can create your own AutoCorrect entries. Question 19Select one: True Falsearrow_forward
- By default, all text is formatted using the Normal Style. Question 20Select one: True Falsearrow_forwardNode.js, Express.js, MongoDB, and Mongoose: Create, Read, Update, and Delete Operations There is a program similar to this assignment given as the last example, CRUD, in the lecture notes for the week that discusses the introduction to MongoDB. Basically, you need to adapt this example program to the data given in this assignment. This program will take more time that previous assignments. So, hopefully you'll start early and you've kept to the schedule in terms of reading the lecture notes. You can use compass if you want to create this database. Or, when your connection string in the model runs it will create the database for you if one does not yet exist. So, ⚫ create a Mongoose model based on the info given below. The index.html page is given in the same folder as these notes. • When you successfully run index.js and instantiate the model, your database is created. • Once the database is created, you need to perfect the addCar route so you can add data using the index.html page. •…arrow_forward1. Enabled with SSL, HTTPS protocol is widely used to provide secure Web services to Web users using Web browsers on the Internet. How is a secure communication channel established at the start of communication between a Web server running HTTPS and a Web browser? Consider the following threats to Web security and how each of these threats is countered by a particular feature of SSL. Man-in-the-middle attack: An attacker interposes during key exchange, acting as the client to the server and as the server to the client. Password sniffing: Passwords in HTTP or other application traffic are “eavesdropped.” SYN flooding: An attacker sends TCP SYN messages to request a connection but does not respond to the final message to establish the connection fully. The attacked TCP module typically leaves the “half-open” connection around for a few minutes. Repeated SYN messages can clog the TCP module.arrow_forward
- SQL Injection on UPDATE Statement for educational purpose only Based on the information below how do i update this code in order to update the emplyees field, eg admin nickname, email,address, phone number etc? ' ; UPDATE users SET NickName='Hacked' WHERE role='admin' -- If a SQL injection vulnerability happens to an UPDATE statement, the damage will be more severe, because attackers can use the vulnerability to modify databases. In our Employee Management application, there is an Edit Profile page (Figure 2) that allows employees to update their profile information, including nickname, email, address, phone number, and password. To go to this page, employees need to log in first. When employees update their information through the Edit Profile page, the following SQL UPDATE query will be executed. The PHP code implemented in unsafe edit backend.php file is used to update employee’s profile information. The PHP file is located in the /var/www/SQLInjection directory.arrow_forwardAnswer two JAVA OOP questions.arrow_forwardPlease answer two Java OOP questions.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Operations Research : Applications and AlgorithmsComputer ScienceISBN:9780534380588Author:Wayne L. WinstonPublisher:Brooks ColeC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
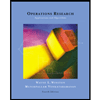
Operations Research : Applications and Algorithms
Computer Science
ISBN:9780534380588
Author:Wayne L. Winston
Publisher:Brooks Cole
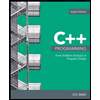
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
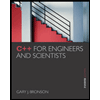
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr