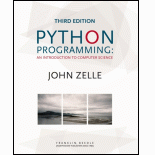
Python Programming: An Introduction to Computer Science
3rd Edition
ISBN: 9781590282779
Author: John Zelle
Publisher: Franklin Beedle & Associates
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 3, Problem 12PE
Program Plan Intro
Sum of cubes of first n natural numbers
- Define the main function.
- Get the value of “n” from the user.
- Declare a variable “sum” and assign 0 to it.
- Loop from 0 through n.
- Find the cube of a number and store it in a variable “cube”.
- Calculate the value of sum by adding “sum” and “cube” and store it in a variable “sum”.
- Print the value of “sum”.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Of the five primary components of an information system (hardware, software, data, people, process), which do you think is the most important to the success of a business organization?
Part A - Define each primary component of the information system.
Part B - Include your perspective on why your selection is most important.
Part C - Provide an example from your personal experience to support your answer.
Management Information Systems
Q2/find the transfer function C/R for the system shown in the figure
Re
ད
Chapter 3 Solutions
Python Programming: An Introduction to Computer Science
Ch. 3 - Prob. 1TFCh. 3 - Prob. 2TFCh. 3 - Prob. 3TFCh. 3 - Prob. 4TFCh. 3 - Prob. 5TFCh. 3 - Prob. 6TFCh. 3 - Prob. 7TFCh. 3 - Prob. 8TFCh. 3 - Prob. 9TFCh. 3 - Prob. 10TF
Ch. 3 - Prob. 1MCCh. 3 - Prob. 2MCCh. 3 - Prob. 3MCCh. 3 - Prob. 4MCCh. 3 - Prob. 5MCCh. 3 - Prob. 6MCCh. 3 - Prob. 7MCCh. 3 - Prob. 8MCCh. 3 - Prob. 9MCCh. 3 - Prob. 10MCCh. 3 - Prob. 1DCh. 3 - Prob. 3DCh. 3 - Prob. 4DCh. 3 - Prob. 6DCh. 3 - Prob. 1PECh. 3 - Prob. 2PECh. 3 - Prob. 3PECh. 3 - Prob. 4PECh. 3 - Prob. 5PECh. 3 - Prob. 6PECh. 3 - Prob. 7PECh. 3 - Prob. 8PECh. 3 - Prob. 9PECh. 3 - Prob. 10PECh. 3 - Prob. 11PECh. 3 - Prob. 12PECh. 3 - Prob. 13PECh. 3 - Prob. 14PECh. 3 - Prob. 15PECh. 3 - Prob. 16PECh. 3 - Prob. 17PE
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Please original work select a topic related to architectures or infrastructures (Data Lakehouse Architecture). Discussing how you would implement your chosen topic in a data warehouse project Please cite in text references and add weblinksarrow_forwardPlease original work What topic would be related to architectures or infrastructures. How you would implement your chosen topic in a data warehouse project. Please cite in text references and add weblinksarrow_forwardWhat is cloud computing and why do we use it? Give one of your friends with your answer.arrow_forward
- What are triggers and how do you invoke them on demand? Give one reference with your answer.arrow_forwardDiscuss with appropriate examples the types of relationships in a database. Give one reference with your answer.arrow_forwardDetermine the velocity error constant (k,) for the system shown. + R(s)- K G(s) where: K=1.6 A(s+B) G(s) = as²+bs C(s) where: A 14, B =3, a =6. and b =10arrow_forward
- • Solve the problem (pls refer to the inserted image)arrow_forwardWrite .php file that saves car booking and displays feedback. There are 2 buttons, which are <Book it> <Select a date>. <Select a date> button gets an input from the user, start date and an end date. Book it button can be pressed only if the start date and ending date are chosen by the user. If successful, it books cars for specific dates, with bookings saved. Booking should be in the .json file which contains all the bookings, and have the following information: Start Date. End Date. User Email. Car ID. If the car is already booked for the selected period, a failure message should be displayed, along with a button to return to the homepage. In the booking.json file, if the Car ID and start date and end date matches, it fails Use AJAX: Save bookings and display feedback without page refresh, using a custom modal (not alert).arrow_forwardWrite .php file with the html that saves car booking and displays feedback. Booking should be in the .json file which contains all the bookings, and have the following information: Start Date. End Date. User Email. Car ID. There are 2 buttons, which are <Book it> <Select a date> Book it button can be pressed only if the start date and ending date are chosen by the user. If successful, book cars for specific dates, with bookings saved. If the car is already booked for the selected period, a failure message should be displayed, along with a button to return to the homepage. Use AJAX: Save bookings and display feedback without page refresh, using a custom modal (not alert). And then add an additional feature that only free dates are selectable (e.g., calendar view).arrow_forward
- • Solve the problem (pls refer to the inserted image) and create line graph.arrow_forwardwho started the world wide webarrow_forwardQuestion No 1: (Topic: Systems for collaboration and social business The information systems function in business) How does Porter's competitive forces model help companies develop competitive strategies using information systems? • List and describe four competitive strategies enabled by information systems that firms can pursue. • Describe how information systems can support each of these competitive strategies and give examples.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
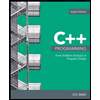
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
Constants, Variables, Data types, Keywords in C Programming Language Tutorial; Author: LearningLad;https://www.youtube.com/watch?v=d7tdL-ZEWdE;License: Standard YouTube License, CC-BY