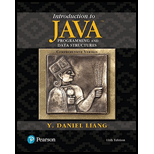
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
11th Edition
ISBN: 9780134670942
Author: Y. Daniel Liang
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 27.4, Problem 27.4.5CP
Program Plan Intro
Open Addressing:
- Open Addressing is a method of finding an open location in the hash table at the time of collision.
- There are several variations for open addressing such as linear probing, quadratic probing, and double hashing.
Quadratic Probing:
- Quadratic probing is one other variation of open addressing.
- It is introduced to avoid the clustering problem in linear probing.
- Quadratic probing will look at the cells at indices (k + j2) % n, for j ≥ 0, i.e., k, (k + 1) % n, (k + 4) % n, (k + 9) % n, ..., and so on.
- Starting from the initial index, quadratic probing will add an increment of 2 to k to define a search sequence.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Asap, i will rate positive for correct , else negative rate.
A linear probing hash table of length 10 uses the hash function h(x) = x mod 10 + 1. mod is the reminder operator. For example the h(42) = 42 mod 10 + 1 = 2 + 1 = 3 so we insert 42 in the position 3 in the array.
After inserting 6 integer keys into an initially empty hash table, the array of keys is…
Consider a simple hash function as "key mod 8" and a sequence of keys as
75, 89, 42, 58, 94, 38, 32, 21. What will be the final sequence if you insert
those keys in an array of size 8 using linear probe.
Chapter 27 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Ch. 27.2 - Prob. 27.2.1CPCh. 27.3 - Prob. 27.3.1CPCh. 27.3 - Prob. 27.3.2CPCh. 27.3 - Prob. 27.3.3CPCh. 27.3 - Prob. 27.3.4CPCh. 27.3 - Prob. 27.3.5CPCh. 27.3 - Prob. 27.3.6CPCh. 27.3 - If N is an integer power of the power of 2, is N /...Ch. 27.3 - Prob. 27.3.8CPCh. 27.3 - Prob. 27.3.9CP
Ch. 27.4 - Prob. 27.4.1CPCh. 27.4 - Prob. 27.4.2CPCh. 27.4 - Prob. 27.4.3CPCh. 27.4 - Prob. 27.4.4CPCh. 27.4 - Prob. 27.4.5CPCh. 27.4 - Prob. 27.4.6CPCh. 27.5 - Prob. 27.5.1CPCh. 27.6 - Prob. 27.6.1CPCh. 27.6 - Prob. 27.6.2CPCh. 27.6 - Prob. 27.6.3CPCh. 27.7 - Prob. 27.7.1CPCh. 27.7 - What are the integers resulted from 32 1, 32 2,...Ch. 27.7 - Prob. 27.7.3CPCh. 27.7 - Describe how the put(key, value) method is...Ch. 27.7 - Prob. 27.7.5CPCh. 27.7 - Show the output of the following code:...Ch. 27.7 - If x is a negative int value, will x (N 1) be...Ch. 27.8 - Prob. 27.8.1CPCh. 27.8 - Prob. 27.8.2CPCh. 27.8 - Can lines 100103 in Listing 27.4 be removed?Ch. 27.8 - Prob. 27.8.4CPCh. 27 - Prob. 27.1PECh. 27 - Prob. 27.2PECh. 27 - (Modify MyHashMap with duplicate keys) Modify...Ch. 27 - Prob. 27.6PECh. 27 - Prob. 27.7PECh. 27 - Prob. 27.8PECh. 27 - Prob. 27.10PECh. 27 - Prob. 27.11PECh. 27 - (setToList) Write the following method that...Ch. 27 - (The Date class) Design a class named Date that...Ch. 27 - (The Point class) Design a class named Point that...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Take a look at a straightforward hash function like "key mod 8" and a series of keys like 75, 89, 42, 58, 94, 38, 32, and 21. What would the finished sequence look like if you use a linear probe to insert those keys into an 8-by-8 array?arrow_forwardUsing a hash table size of 11, modulo hash method and linear probing, what does the hash table look like (show me the 11 entries) after you add the following keys: 21, 54, 60, 43, 27, 44, 55, 66, 77. write your answer using - to represent an entry with no value Write your answer with a single space between the keys. example: 21 54 60 43 - - 27 44 55 66 77 for the 11 entriesarrow_forwardImplement a commonly used hash table in a program that handles collision using linear probing. Using (K mod 13) as the hash function, store the following elements in the table: {1, 5, 21, 26, 39, 14, 15, 16, 17, 18, 19, 20, 111, 145, 146}.arrow_forward
- 2-A linear probing hash table of length 10 uses the hash function h(x) = x mod 10 + 1. mod is the reminder opertatro. For example the h(42) = 42 mod 10 + 1 = 2 + 1 = 3 so we insert 42 in t he position 3 in the array. After inserting 6 integer keys into an initially empty hash table, the array of keys is 1 2 3 4 5 7 8 9. 42 23 34 52 46 33 a- insert the key 35 b- insert the key 12 c- insert the key 10arrow_forwardGiven a hash table of size 9 with hash function x%9. Write the element order of the linear probe hash table when these keys are inserted in the given order. 1,20,11,28,25,9,18 Answer is just a sequence of elements in the same format. Use hypen - to indicate empty space. For example a table like below must be written as in the following: 11 28 9,28,-,11,1,-,- Cevap: Kalan Süre 1:37:31arrow_forwardSuppose you have a hash table of size N = 256, and you are using double hashing. The keys in your hash are 4-digit integers (0000 through 9999) and your hash function is h(k) = (k²)%N The second hash function (used for probing) is h₂(k) = (sum-of-digits (k) * 8)%N What are the first 4 values in the search sequence (starting with the home position) for a record with key k-2025?arrow_forward
- How would the table look like if we used separate chaining (open hashing)arrow_forwardConsider a hash table of size 100 named marks Table that uses linear probing and a hash function of key % 5. What would be the hash table index (0-based) of key 46? marks Table.insert(47); marks Table.insert(41); marks Table.insert(42); marks Table.insert(44); marks Table.insert(46);arrow_forwardWhat is the average-case runtime required for a successful operation in a hash table using chaining where there are m buckets and n items in the hash table? Select one: a. O( n/m ) b. O(n) The answer depends on both n and m c. O( 1) d. O(m)arrow_forward
- Using chained hashing. You have a Hash table of size 7. You will add the following elements in the order given using the function %5. 18 - 44 - 31 - 11 - 8 А. 1 44 31 18 11 В. 3 4 6. 44 11 18 31 8 С. 1 3 4 6. 31 18 44 11 8. D. The correct answer is not listed 00 00 B.arrow_forwardRehash the hash table below (separate chaining, length 5) to a new length of 10. Draw the new hash table after rehashing, Assume that hashCode (x) = x for this question. Assume that we always traverse a chain from head to tail and always insert at the head of the chain example 0:15->30 1:6->21->11 2: 3: A 4:-14arrow_forwardGenerate 11 unique random elements and perform the extendible hashing. The bucket size is three (3) and the Hash function is the following: H(X) => Suppose the global depth is X. Then the Hash Functi9on returns X LSBs. Make sure that in every hashing step there is an equivalent explanation.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
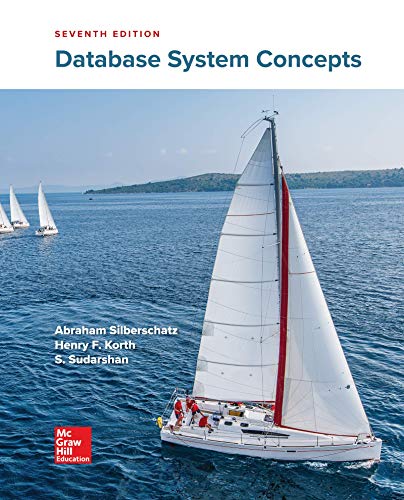
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
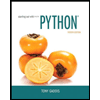
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
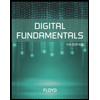
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
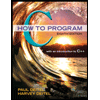
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
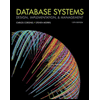
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
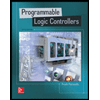
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education