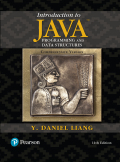
Introduction to Java Programming and Data Structures Comprehensive Version (11th Edition)
11th Edition
ISBN: 9780134700144
Author: Liang
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 27, Problem 27.10PE
Program Plan Intro
MyHashSet.java
Program Plan:
- Include a class name named “Exercise_10”.
- Declare the main() method.
- Shuffle the data.
- Create a new arrayList.
- Create a new HashSet.
- Add integers into the hash set.
- Add current time.
- Add integers into the arraylist.
- Close the main() method.
- Close the class.
- Include a static class “MyArrayList”.
- Declare all the data types of the variables.
- Create data array.
- Create a default list constructor.
- Create a list from an array of objects.
- User shouldn't add super(objects)!
- Override method to add a new element at the specified index.
- The elements are moved to the right after the specified index.
- New elements are inserted to data[index].
- Declare a method to create a new larger array and double its current size + 1.
- Override method to clear the list.
- Override method to return true if this list contains the element.
- Override method to return the element at the specified index.
- Override method to return the index of the first matching element in this list and return -1 if no match.
- Override method to return the index of the last matching element in this list and return -1 if no match.
- Override method to replace the element at the specified position in this list with the specified element.
- Declare a Method to trim the capacity to current size.
- Close class.
- Include a class “Iterator”.
- Declare current index.
- Declare a Boolean method hasNext().
- Declare a method remove().
- Close class.
- Include an interface “MyList”.
- Declare a Method to add a new element at the end of this list.
- Declare a method to add a new element at the specified index in this list.
- Declare a method to clear the list.
- Declare a Method to return true if this list contains the element.
- Declare a Method to return the element from this list at the specified index.
- Declare a method to remove the first occurrence of the element o from this list. Shift any subsequent elements to the left. Return true if the element is removed.
- Declare a Method to remove the element at the specified position in this list Shift any subsequent elements to the left. Return the element that was removed from the list.
- Declare a method to replace the element at the specified position in this list with the specified element and returns the new set.
- Include a static abstract class “ MyAbstractList”.
- Declare the size of the list.
- Define a Constructor to create a default list.
- Define a constructor to create a list from an array of objects.
- Declare an override method to add a new element at the end of this list.
- Declare an override method to return the number of elements in this list.
- Declare an override method to remove the first occurrence of the element e from this list. Shift any subsequent elements to the left. Return true if the element is removed
- Declare a method that adds the elements in otherList to this list. Returns true if this list changed as a result of the call.
- Declare a method that removes all the elements in otherList from this list Returns true if this list changed as a result of the call.
- Include a static class “MyHashSet”.
- Define the default hash table size. Must be a power of 2.
- Define the maximum hash table size. 1 << 30 is same as 2^30.
- Declare current hash table capacity. Capacity is a power of 2.
- Define default load factor.
- Specify a load factor threshold used in the hash table.
- Declare a Hash table that is an array with each cell that is a linked list.
- Declare a constructor to Construct a set with the default capacity and load factor.
- Declare a constructor to Construct a set with the specific capacity and load factor.
- Declare an override method that Remove all elements from this set.
- Declare an override method that Return true if the element is in the set.
- Declare an override method that adds an element to the set.
- Create a linked list for the bucket if it is not created.
- Declare an override method that Remove the element from the set.
- Declare an override method that Return the number of elements in the set.
- Declare an override method to return the number of iterators.
- Include an inner class named “MyHashSetIterator”.
- Declare an inner class for iterator.
- Store the elements in a list.
- Declare a constructor to create a list from the set.
- Declare an override the next element for traversing.
Declare an override method that remove the current element and refresh the list.
- Declare a Method to ensure the hashing is evenly distributed.
- Declare a Method to return a power of 2 for initial Capacity.
- Declare a method to Remove all e from each bucket.
- Declare a method to Rehash the set
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
how are you
need help with thi
Next, you are going to combine everything you've learned about HTML and CSS to make a static site portfolio piece.
The page should first introduce yourself. The content is up to you, but should include a variety of HTML elements, not just text.
This should be followed by an online (HTML-ified) version of your CV (Resume).
The following is a minimum list of requirements you should have across all your content:
Both pages should start with a CSS reset (imported into your CSS, not included in your HTML)
Semantic use of HTML5 sectioning elements for page structure
A variety other semantic HTML elements
Meaningful use of Grid, Flexbox and the Box Model as appropriate for different layout components
A table
An image
Good use of CSS Custom Properties (variables)
Non-trivial use of CSS animation
Use of pseudeo elements
An accessible colour palette
Use of media queries
The focus of this course is development, not design. However, being able to replicate a provided design…
Using the notation
Chapter 27 Solutions
Introduction to Java Programming and Data Structures Comprehensive Version (11th Edition)
Ch. 27.2 - Prob. 27.2.1CPCh. 27.3 - Prob. 27.3.1CPCh. 27.3 - Prob. 27.3.2CPCh. 27.3 - Prob. 27.3.3CPCh. 27.3 - Prob. 27.3.4CPCh. 27.3 - Prob. 27.3.5CPCh. 27.3 - Prob. 27.3.6CPCh. 27.3 - If N is an integer power of the power of 2, is N /...Ch. 27.3 - Prob. 27.3.8CPCh. 27.3 - Prob. 27.3.9CP
Ch. 27.4 - Prob. 27.4.1CPCh. 27.4 - Prob. 27.4.2CPCh. 27.4 - Prob. 27.4.3CPCh. 27.4 - Prob. 27.4.4CPCh. 27.4 - Prob. 27.4.5CPCh. 27.4 - Prob. 27.4.6CPCh. 27.5 - Prob. 27.5.1CPCh. 27.6 - Prob. 27.6.1CPCh. 27.6 - Prob. 27.6.2CPCh. 27.6 - Prob. 27.6.3CPCh. 27.7 - Prob. 27.7.1CPCh. 27.7 - What are the integers resulted from 32 1, 32 2,...Ch. 27.7 - Prob. 27.7.3CPCh. 27.7 - Describe how the put(key, value) method is...Ch. 27.7 - Prob. 27.7.5CPCh. 27.7 - Show the output of the following code:...Ch. 27.7 - If x is a negative int value, will x (N 1) be...Ch. 27.8 - Prob. 27.8.1CPCh. 27.8 - Prob. 27.8.2CPCh. 27.8 - Can lines 100103 in Listing 27.4 be removed?Ch. 27.8 - Prob. 27.8.4CPCh. 27 - Prob. 27.1PECh. 27 - Prob. 27.2PECh. 27 - (Modify MyHashMap with duplicate keys) Modify...Ch. 27 - Prob. 27.6PECh. 27 - Prob. 27.7PECh. 27 - Prob. 27.8PECh. 27 - Prob. 27.10PECh. 27 - Prob. 27.11PECh. 27 - (setToList) Write the following method that...Ch. 27 - (The Date class) Design a class named Date that...Ch. 27 - (The Point class) Design a class named Point that...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- you can select multipy optionsarrow_forwardFor each of the following, decide whether the claim is True or False and select the True ones: Suppose we discover that the 3SAT can be solved in worst-case cubic time. Then it would mean that all problems in NP can also be solved in cubic time. If a problem can be solved using Dynamic Programming, then it is not NP-complete. Suppose X and Y are two NP-complete problems. Then, there must be a polynomial-time reduction from X to Y and also one from Y to X.arrow_forwardMaximum Independent Set problem is known to be NP-Complete. Suppose we have a graph G in which the maximum degree of each node is some constant c. Then, is the following greedy algorithm guaranteed to find an independent set whose size is within a constant factor of the optimal? 1) Initialize S = empty 2) Arbitrarily pick a vertex v, add v to S delete v and its neighbors from G 3) Repeat step 2 until G is empty Return S Yes Noarrow_forward
- Please help me answer this coding question in the images below for me(it is not a graded question):write the code using python and also provide the outputs requiredarrow_forwardWhat does the reduction showing Vertex Cover (VC) is NP-Complete do: Transforms any instance of VC to an instance of 3SAT Transforms any instance of 3SAT to an instance of VC Transforms any instance of VC to an instance of 3SAT AND transforms any instance of 3SAT to an instance of VC none of the abovearrow_forwardPlease assist me by writing out the code with its output (in python) using the information provided in the 2 images below.for the IP Address, it has been changed to: 172.21.5.204the serve code has not been open yet though but the ouput must be something along these lines(using command prompt):c:\Users\japha\Desktop>python "Sbongakonke.py"Enter the server IP address (127.0.0.1 or 172.21.5.199): 172.21.5.204Enter your student number: 4125035Connected to server!It's your turn to pour! Enter the amount to your pour (in mL):Please work it out until it gets the correct outputsNB: THIS QUESTION IS NOT A GRADED QUESTIONarrow_forward
- need help with a html code and css code that will match this image.arrow_forwardneed help with a html code and css code that will match this image. Part B - A Navigation Part B is the navigation component of a page. Information you need includes: Color Codes: Visiting links: #ff6666 Unvisited links: #ccff66 Hovered links: white Search box: #2ec4b6 rebeccapurple white Font: Google Font (Roboto) Icons: Font Awesome (fa-quidditch, fa-search) This is a flexbox based navigation menu. Other then padding, all spacing/positioning should be controlled using flex properties. The home link in the nav should point to your assignment file (to triggers visited styling). In the "state" screenshot below, Home is visited, Services is hovered (the mouse doesn't show up in the screenshot) and Products is unvisited.arrow_forwardMGMT SS STATS, an umbrella body that facilitates and serves various Social Security Organizations/Departments within the Caribbean territories, stood poised to meet the needs of its stakeholders by launching an online database. The database will provide members and the public access to the complete set of services that can (also) be initiated face-to-face, and it will provide managed, private, secure access to a repository of public and/or personal information. Ideally, the database will have basic details of pension plans recorded in the registry, member plan statistics, and cash inflows and outflows from pension funds.For example, insured persons accumulate contributions. Records for these persons will include information on the insured persons able to acquire various benefits once work is interrupted due to sickness, death, retirement, and maternity or employment injury. They will also include information on pensions such as invalidity, disability, and survivors that stem from one…arrow_forward
- Why all appvif i want to sign in its required phone number why not using google or apple its make me frustratedarrow_forwardWhy is the accuracy of time important in data visualizations? Detail a scenario from your professional experience in which time was structured poorly in a data visualization. How did this affect the understanding of the data presented? How do you think this error or oversight occurred?arrow_forwardWrite the KeanStudent class. The UML diagram of the class is represented below: KeanStudent - fullName: String - keanID: int -keanEmailAddress: String cellPhoneNumber: String + numberOfStudent: int + KeanStudent() + KeanStudent(fullName: String, keanID: int, keanEmailAddress: String, cellPhoneNumber: String) +getFullName(): String +setFullName(newFullName: String): void +getKeanIDO): int +getKeanEmailAddress(): String +getCellPhoneNumber(): String + setCellPhoneNumber(newCellPhoneNumber: String): void +toString(): String 1. Implement the KeanStudent class strictly according to its UML one-to-one (do not include anything extra, do not miss any data fields or methods) 2. Implement a StudentTest class to test the class KeanStudent you just created. • Create two KeanStudent objects using a no-args constructor and one from the constructor with all fields. o Print the contents of both objects. 。 Print numberOfStudent. 3. Add comments to your program (mark where data fields, constructors,…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage Learning
- New Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage LearningMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
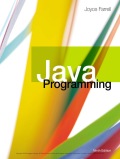
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
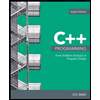
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
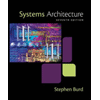
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning
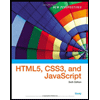
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:9781305503922
Author:Patrick M. Carey
Publisher:Cengage Learning
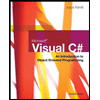
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage