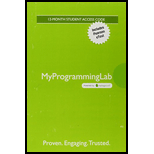
MyLab Programming with Pearson eText -- Access Card -- for Introduction to Java Programming and Data Structures, Comprehensive Version
11th Edition
ISBN: 9780134672816
Author: Liang
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 23, Problem 23.3PE
Program Plan Intro
Generic quick sort
Program Plan:
- Import the required packages.
- Create a class “Sorting”:
- Method to sort the numbers gets defined.
- Call the method quick sort that implements the comparable interface.
- Method to sort the string given.
- Define the main method
- Initialize the list that needs to be sorted.
- Call the quick sort.
- Display the sorted list.
- Initialize list that contain strings.
- Call the method quick sort.
- Display the sorted list.
- Define the method “quickSort()”
- Condition to validate the first element and last element.
- Partition the list .
- Sort the list by calling the method quick sort.
- Define the method “partition()”
- Perform swap operation by comparing the list.
- Loop that iterates to validate the first and last elements of the list.
- Return the begin and last value after validating.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Design a dynamic programming algorithm for the Coin-change problem described below:
Input: An amount of money C and a set of n possible coin values with an unlimited supply of each
kind of coin.
Output: The smallest number of coins that add up to C exactly, or output that no such set exists.
The algorithm must take O(n C) time. You must also write and explain the recurrence.
Example 1:
Input: C24, Coin values = = [1, 5, 10, 25, 50]
Output: 6 (since 24 = 10+ 10+1+1 +1 + 1)
Example 2:
Input: C = 86, Coin values = [1, 5, 6, 23, 35, 46, 50]
Output: 2 (since 86 = 46+35+5)
Design a dynamic programming algorithm for the Longest Common Subsequence problem de-
scribed below
Input: Two strings x = x1x2 xm and y = Y1Y2... Yn
Output: The length of the longest subsequence that is common to both x and y.
.
The algorithm must take O(m n) time. You must also write and explain the recurrence.
(I want the largest k such that there are 1 ≤ i₁ < ... < ik ≤ m and 1 ≤ j₁ < ... < jk ≤ n such that
Xi₁ Xi2 Xik = Yj1Yj2 ··· Yjk)
Example 1:
Input: x = 'abcdefghijklmnopqrst' and y = 'ygrhnodsh ftw'
Output: 6 ('ghnost' is the longest common subsequence to both strings)
Example 2:
Input: x = 'ahshku' and y = ‘asu'
Output: 3 ('asu' is the longest common subsequence to both strings)
Design a dynamic programming algorithm for the problem described below
Input: A list of numbers A = = [a1,..., an].
Output: A contiguous subsequence of numbers with the maximum sum.
The algorithm must take O(n) time. You must also write and explain the recurrence.
(I am looking for an i ≥ 1 and k ≥ 0 such that a + ai+1 + ···ai+k has the largest possible sum among all
possible values for i and k.)
Example 1:
Input: A[5, 15, -30, 10, -5, 40, 10].
Output: [10, 5, 40, 10]
Example 2:
Input: A = [7, 5, 7, 4, -20, 6, 9, 3, -4, -8, 4]
Output: [6,9,3]
Chapter 23 Solutions
MyLab Programming with Pearson eText -- Access Card -- for Introduction to Java Programming and Data Structures, Comprehensive Version
Ch. 23.2 - Prob. 23.2.1CPCh. 23.2 - Prob. 23.2.2CPCh. 23.2 - Prob. 23.2.3CPCh. 23.3 - Prob. 23.3.1CPCh. 23.3 - Prob. 23.3.2CPCh. 23.3 - Prob. 23.3.3CPCh. 23.4 - Prob. 23.4.1CPCh. 23.4 - Prob. 23.4.2CPCh. 23.4 - What is wrong if lines 615 in Listing 23.6,...Ch. 23.5 - Prob. 23.5.1CP
Ch. 23.5 - Prob. 23.5.2CPCh. 23.5 - Prob. 23.5.3CPCh. 23.5 - Prob. 23.5.4CPCh. 23.6 - Prob. 23.6.1CPCh. 23.6 - Prob. 23.6.2CPCh. 23.6 - Prob. 23.6.3CPCh. 23.6 - Prob. 23.6.4CPCh. 23.6 - Prob. 23.6.5CPCh. 23.6 - Prob. 23.6.6CPCh. 23.6 - Prob. 23.6.7CPCh. 23.6 - Prob. 23.6.8CPCh. 23.6 - Prob. 23.6.9CPCh. 23.7 - Prob. 23.7.1CPCh. 23.7 - Prob. 23.7.2CPCh. 23.8 - Prob. 23.8.1CPCh. 23 - Prob. 23.1PECh. 23 - Prob. 23.2PECh. 23 - Prob. 23.3PECh. 23 - (Improve quick sort) The quick-sort algorithm...Ch. 23 - (Check order) Write the following overloaded...Ch. 23 - Prob. 23.7PECh. 23 - Prob. 23.8PECh. 23 - Prob. 23.10PECh. 23 - Prob. 23.11PECh. 23 - Prob. 23.12PECh. 23 - Prob. 23.13PECh. 23 - (Selection-sort animation) Write a program that...Ch. 23 - (Bubble-sort animation) Write a program that...Ch. 23 - (Radix-sort animation) Write a program that...Ch. 23 - (Merge animation) Write a program that animates...Ch. 23 - (Quicksort partition animation) Write a program...Ch. 23 - (Modify merge sort) Rewrite the mergeSort method...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Design a dynamic programming algorithm for the Longest Increasing Subsequence problem described below: Input: A sequence of n integers Output: The length of the longest increasing subsequence among these integers. The algorithm must take O(n²) time. You must also write and explain the recurrence. Example 1: Input: [5, 3, 6, 8, 4, 6, 2, 7, 9, 5] Output: 5 ([3, 4, 6, 7, 9]) Example 2: Input: [12, 42, 66, 73, 234, 7, 543, 16] Output: 6 ([42, 66, 73, 234, 543])arrow_forwardDesign a dynamic programming algorithm for the Subset Sum problem described below: Input: A set of n integers A and an integer s Output: A subset of A whose numbers add up to s, or that no such set exists. The algorithm must take O(n·s) time. You must also write and explain the recurrence. Example 1: Input: A = {4, 7, 5, 2, 3}, s = 12 Output: {7,2,3} Example 2: Input: A{4, 7, 5,3}, s = 6 Output: 'no such subset'arrow_forwardTECNOLOGIE DEL WEB 2023/2023 (VER 1.1) Prof. Alfonso Pierantonio 1. Project Requirements The project consists in designing and implementing a Web application according to the methodology and the technologies illustrated and developed during the course. This document describe cross-cutting requirements the application must satisfy. The application must be realized with a combination of the following technologies: PHP MySQL HTML/CSS JavaScript, jQuery, etc templating The requirements are 2. Project size The application must have at least 18 SQL tables The number of SQL tables refers to the overall number of tables (including relation normalizations). 3. Methodology The application must be realized by adopting separation of logics, session management, and generic user management (authentication/permissions). Missing one of the above might correspond to a non sufficient score for the project. More in details: 3.1 Separation of Logics The separation of logics has to be realizse by using…arrow_forward
- Write a C program to calculate the function sin(x) or cos(x) using a Taylor series expansion around the point 0. In other words, you will program the sine or cosine function yourself, without using any existing solution. You can enter the angles in degrees or radians. The program must work for any input, e.g. -4500° or +8649°. The function will have two arguments: float sinus(float radians, float epsilon); For your own implementation, use one of the following relations (you only need to program either sine or cosine, you don't need both): Tip 1: Of course, you cannot calculate the sum of an infinite series indefinitely. You can see (if not, look in the program) that the terms keep getting smaller, so there will definitely be a situation where adding another term will not change the result in any way (see problem 1.3 – machine epsilon). However, you can end the calculation even earlier – when the result changes by less than epsilon (a pre-specified, sufficiently small number, e.g.…arrow_forwardWrite a C program that counts the number of ones (set bits) in the binary representation of a given number. Example:Input: 13 (binary 1101)Output: 3 unitsarrow_forwardI need help to resolve or draw the diagrams. thank youarrow_forward
- You were requested to design IP addresses for the following network using the addressblock 166.118.10.0/8, connected to Internet with interface 168.118.40.17 served by the serviceprovider with router 168.118.40.1/20.a) Specify an address and net mask for each network and router interface in the table provided. b) Give the routing table at Router 1.c) How will Router 1 route the packets with destinationi) 168.118.10.5ii) 168.118.10.103iii) 168.119.10.31iii) 168.118.10.153arrow_forwardI would like to get help to draw an object relationship diagram for a typical library system.arrow_forwardGiven the network of bridges in figure, and assuming that LAN ports on A, B, C, D, E, J are 10 Mbs (cost 100 for ports) except for ports on F, G, I, H, K which are 100Mbps LANs (cost 19 for ports) Draw the obtained spanning tree, cross the blocking state ports, and circle the designated ports and write the best cost broadcasted by each router next to its root port. list in logic level detail the expected last STP messages that will define the final status at each router.arrow_forward
- Next, you are going to combine everything you've learned about HTML and CSS to make a static site portfolio piece. The page should first introduce yourself. The content is up to you, but should include a variety of HTML elements, not just text. This should be followed by an online (HTML-ified) version of your CV (Resume). The following is a minimum list of requirements you should have across all your content: Both pages should start with a CSS reset (imported into your CSS, not included in your HTML) Semantic use of HTML5 sectioning elements for page structure A variety other semantic HTML elements Meaningful use of Grid, Flexbox and the Box Model as appropriate for different layout components A table An image Good use of CSS Custom Properties (variables) Non-trivial use of CSS animation Use of pseudeo elements An accessible colour palette Use of media queries The focus of this course is development, not design. However, being able to replicate a provided design for the web is…arrow_forwardI would like to get help to draw an object relationship diagram for a typical library system.arrow_forwardhttps://docs.google.com/document/d/1lk0DgaWfVezagyjAEskyPoe9Ciw3J2XUH_HQfnWSmwU/edit?usp=sharing using the link for the case study answer the below questionarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
- New Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage LearningSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
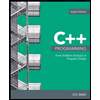
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
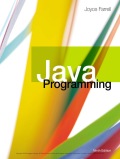
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
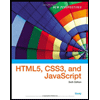
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:9781305503922
Author:Patrick M. Carey
Publisher:Cengage Learning
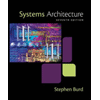
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning
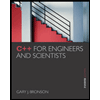
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr