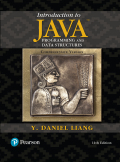
Introduction to Java Programming and Data Structures Comprehensive Version (11th Edition)
11th Edition
ISBN: 9780134700144
Author: Liang
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 23, Problem 23.12PE
Program Plan Intro
Radix sort
Program Plan:
- Import the required packages.
- Create a class “Sorting”:
- Define the main method
- New list gets created.
- Loop that iterates to generate the numbers is defined.
- Add the elements into the list.
- Perform radix sort.
- Display the elements.
- Define a method “radixsort()”
- New bucket list gets created.
- Loop that iterates to add the elements into the bucket are created.
- Loop that iterates to position the digits present in the bucket is defined.
- Position and clear the bucket.
- Loop that iterates for all digits and position the elements and add it to the bucket.
- Define the method “getKey()”
- Declare the required variables.
- Loop that iterates to add the result.
- Return the resultant digit.
- New bucket list gets created.
- Define the main method
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
whats for dinner?
please
Consider the follow program that prints a page number on the left or right side of a page. Define and use a new function, isEven, that returns a Boolean to make the condition in the if statement easier to understand.
ef main() : page = int(input("Enter page number: ")) if page % 2 == 0 : print(page) else : print("%60d" % page)
main()
What is the correct python code for the function def countWords(string) that will return a count of all the words in the string string of workds that are separated by spaces.
Chapter 23 Solutions
Introduction to Java Programming and Data Structures Comprehensive Version (11th Edition)
Ch. 23.2 - Prob. 23.2.1CPCh. 23.2 - Prob. 23.2.2CPCh. 23.2 - Prob. 23.2.3CPCh. 23.3 - Prob. 23.3.1CPCh. 23.3 - Prob. 23.3.2CPCh. 23.3 - Prob. 23.3.3CPCh. 23.4 - Prob. 23.4.1CPCh. 23.4 - Prob. 23.4.2CPCh. 23.4 - What is wrong if lines 615 in Listing 23.6,...Ch. 23.5 - Prob. 23.5.1CP
Ch. 23.5 - Prob. 23.5.2CPCh. 23.5 - Prob. 23.5.3CPCh. 23.5 - Prob. 23.5.4CPCh. 23.6 - Prob. 23.6.1CPCh. 23.6 - Prob. 23.6.2CPCh. 23.6 - Prob. 23.6.3CPCh. 23.6 - Prob. 23.6.4CPCh. 23.6 - Prob. 23.6.5CPCh. 23.6 - Prob. 23.6.6CPCh. 23.6 - Prob. 23.6.7CPCh. 23.6 - Prob. 23.6.8CPCh. 23.6 - Prob. 23.6.9CPCh. 23.7 - Prob. 23.7.1CPCh. 23.7 - Prob. 23.7.2CPCh. 23.8 - Prob. 23.8.1CPCh. 23 - Prob. 23.1PECh. 23 - Prob. 23.2PECh. 23 - Prob. 23.3PECh. 23 - (Improve quick sort) The quick-sort algorithm...Ch. 23 - (Check order) Write the following overloaded...Ch. 23 - Prob. 23.7PECh. 23 - Prob. 23.8PECh. 23 - Prob. 23.10PECh. 23 - Prob. 23.11PECh. 23 - Prob. 23.12PECh. 23 - Prob. 23.13PECh. 23 - (Selection-sort animation) Write a program that...Ch. 23 - (Bubble-sort animation) Write a program that...Ch. 23 - (Radix-sort animation) Write a program that...Ch. 23 - (Merge animation) Write a program that animates...Ch. 23 - (Quicksort partition animation) Write a program...Ch. 23 - (Modify merge sort) Rewrite the mergeSort method...
Knowledge Booster
Similar questions
- Consider the following program that counts the number of spaces in a user-supplied string. Modify the program to define and use a function, countSpaces, instead. def main() : userInput = input("Enter a string: ") spaces = 0 for char in userInput : if char == " " : spaces = spaces + 1 print(spaces) main()arrow_forwardWhat is the python code for the function def readFloat(prompt) that displays the prompt string, followed by a space, reads a floating-point number in, and returns it. Here is a typical usage: salary = readFloat("Please enter your salary:") percentageRaise = readFloat("What percentage raise would you like?")arrow_forwardassume python does not define count method that can be applied to a string to determine the number of occurances of a character within a string. Implement the function numChars that takes a string and a character as arguments and determined and returns how many occurances of the given character occur withing the given stringarrow_forward
- Consider the ER diagram of online sales system above. Based on the diagram answer the questions below, a) Based on the ER Diagram, determine the Foreign Key in the Product Table. Just mention the name of the attribute that could be the Foreign Key. b) Mention the relationship between the Order and Customer Entities. You can use the following: 1:1, 1:M, M:1, 0:1, 1:0, M:0, 0:M c) Is there a direct relationship that exists between Store and Customer entities? Answer Yes/No? d) Which of the 4 Entities mention in the diagram can have a recursive relationship? e) If a new entity Order_Details is introduced, will it be a strong entity or weak entity? If it is a weak entity, then mention its type?arrow_forwardNo aiarrow_forwardGiven the dependency diagram of attributes {C1,C2,C3,C4,C5) in a table shown in the following figure, (the primary key attributes are underlined)arrow_forward
- What are 3 design techniques that enable data representations to be effective and engaging? What are some usability considerations when designing data representations? Provide examples or use cases from your professional experience.arrow_forward2D array, Passing Arrays to Methods, Returning an Array from a Method (Ch8) 2. Read-And-Analyze: Given the code below, answer the following questions. 2 1 import java.util.Scanner; 3 public class Array2DPractice { 4 5 6 7 8 9 10 11 12 13 14 15 16 public static void main(String args[]) { 17 } 18 // Get an array from the user int[][] m = getArray(); // Display array elements System.out.println("You provided the following array "+ java.util.Arrays.deepToString(m)); // Display array characteristics int[] r = findCharacteristics(m); System.out.println("The minimum value is: " + r[0]); System.out.println("The maximum value is: " + r[1]); System.out.println("The average is: " + r[2] * 1.0/(m.length * m[0].length)); 19 // Create an array from user input public static int[][] getArray() { 20 21 PASSTR2222322222222222 222323 F F F F 44 // Create a Scanner to read user input Scanner input = new Scanner(System.in); // Ask user to input a number, and grab that number with the Scanner…arrow_forwardGiven the dependency diagram of attributes C1,C2,C3,C4,C5 in a table shown in the following figure, the primary key attributes are underlined Make a database with multiple tables from attributes as shown above that are in 3NF, showing PK, non-key attributes, and FK for each table? Assume the tables are already in 1NF. Hint: 3 tables will result after deducing 1NF -> 2NF -> 3NFarrow_forward
- Consider the ER diagram of online sales system above. Based on the diagram answer the questions below, 1. Based on the ER Diagram, determine the Foreign Key in the Product Table. Just mention the name of the attribute that could be the Foreign Key 2. Is there a direct relationship that exists between Store and Customer entities? AnswerYes/No?arrow_forwardConsider the ER diagram of online sales system above. Based on the diagram answer thequestions below, 1. Mention the relationship between the Order and Customer Entities. You can use the following: 1:1, 1:M, M:1, 0:1, 1:0, M:0, 0:M 2. Which one of the 4 Entities mention in the diagram can have a recursive relationship? 3. If a new entity Order_Details is introduced, will it be a strong entity or weak entity? If it is a weak entity, then mention its type (ID or Non-ID, also Justify why)? NO AI use pencil and paperarrow_forwardSTEP 1: The skeleton Let's start by creating a skeleton for some of the classes you will need. • Write a class called Tile. You can think of a tile as a square on the board on which the game will be played. We will come back to this class later. For the moment you can leave it empty while you work on creating classes that represents characters in the game. • Write an abstract class Fighter which has the following private fields: - A Tile field named position, representing the fighter's position in the game. A double field named health, representing the fighter's health points (HP). An int field named weaponType, representing the type of weapon the fighter is using. This value is used to rank different weapon types: higher values indicate higher weapon ranks. -An int field named attackDamage, representing the fighter's attack power. The class must also have the following public methods: 3 A constructor that takes as input a Tile indicating the position of the fighter, a double…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage Learning
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
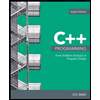
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
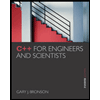
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
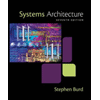
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning
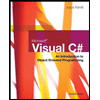
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
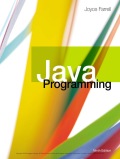
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT