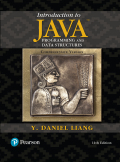
Introduction to Java Programming and Data Structures Comprehensive Version (11th Edition)
11th Edition
ISBN: 9780134700144
Author: Liang
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 22.10, Problem 22.10.2CP
Program Plan Intro
Convex hull:
- The convex hull is otherwise named as “convex envelope” or “convex closure”.
- The convex hull is represented as convex polygon with all the given points.
- This
algorithm takes the input as an array of points through respective “x” and “y” co-ordinates and displays the convex hull with a set of points.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Write the SQL code that permits to implement the tables: Student and Transcript. NB: Add the constraints on the attributes – keys and other.
Draw an ERD that will involve the entity types: Professor, Student, Department and Course. Be sure to add relationship types, key attributes, attributes and multiplicity on the ERD.
Draw an ERD that represents a book in a library system. Be sure to add relationship types, key attributes, attributes and multiplicity on the ERD.
Chapter 22 Solutions
Introduction to Java Programming and Data Structures Comprehensive Version (11th Edition)
Ch. 22.2 - Prob. 22.2.1CPCh. 22.2 - What is the order of each of the following...Ch. 22.3 - Count the number of iterations in the following...Ch. 22.3 - How many stars are displayed in the following code...Ch. 22.3 - Prob. 22.3.3CPCh. 22.3 - Prob. 22.3.4CPCh. 22.3 - Example 7 in Section 22.3 assumes n = 2k. Revise...Ch. 22.4 - Prob. 22.4.1CPCh. 22.4 - Prob. 22.4.2CPCh. 22.4 - Prob. 22.4.3CP
Ch. 22.4 - Prob. 22.4.4CPCh. 22.4 - Prob. 22.4.5CPCh. 22.4 - Prob. 22.4.6CPCh. 22.5 - Prob. 22.5.1CPCh. 22.5 - Why is the recursive Fibonacci algorithm...Ch. 22.6 - Prob. 22.6.1CPCh. 22.7 - Prob. 22.7.1CPCh. 22.7 - Prob. 22.7.2CPCh. 22.8 - Prob. 22.8.1CPCh. 22.8 - What is the difference between divide-and-conquer...Ch. 22.8 - Prob. 22.8.3CPCh. 22.9 - Prob. 22.9.1CPCh. 22.9 - Prob. 22.9.2CPCh. 22.10 - Prob. 22.10.1CPCh. 22.10 - Prob. 22.10.2CPCh. 22.10 - Prob. 22.10.3CPCh. 22 - Program to display maximum consecutive...Ch. 22 - (Maximum increasingly ordered subsequence) Write a...Ch. 22 - (Pattern matching) Write an 0(n) time program that...Ch. 22 - (Pattern matching) Write a program that prompts...Ch. 22 - (Same-number subsequence) Write an O(n) time...Ch. 22 - (Execution time for GCD) Write a program that...Ch. 22 - (Geometry: gift-wrapping algorithm for finding a...Ch. 22 - (Geometry: Grahams algorithm for finding a convex...Ch. 22 - Prob. 22.13PECh. 22 - (Execution time for prime numbers) Write a program...Ch. 22 - (Geometry: noncrossed polygon) Write a program...Ch. 22 - (Linear search animation) Write a program that...Ch. 22 - (Binary search animation) Write a program that...Ch. 22 - (Find the smallest number) Write a method that...Ch. 22 - (Game: Sudoku) Revise Programming Exercise 22.21...Ch. 22 - (Bin packing with smallest object first) The bin...Ch. 22 - Prob. 22.27PE
Knowledge Booster
Similar questions
- 2:21 m Ο 21% AlmaNet WE ARE HIRING Experienced Freshers Salesforce Platform Developer APPLY NOW SEND YOUR CV: Email: hr.almanet@gmail.com Contact: +91 6264643660 Visit: www.almanet.in Locations: India, USA, UK, Vietnam (Remote & Hybrid Options Available)arrow_forwardProvide a detailed explanation of the architecture on the diagramarrow_forwardhello please explain the architecture in the diagram below. thanks youarrow_forward
- Complete the JavaScript function addPixels () to calculate the sum of pixelAmount and the given element's cssProperty value, and return the new "px" value. Ex: If helloElem's width is 150px, then calling addPixels (hello Elem, "width", 50) should return 150px + 50px = "200px". SHOW EXPECTED HTML JavaScript 1 function addPixels (element, cssProperty, pixelAmount) { 2 3 /* Your solution goes here *1 4 } 5 6 const helloElem = document.querySelector("# helloMessage"); 7 const newVal = addPixels (helloElem, "width", 50); 8 helloElem.style.setProperty("width", newVal); [arrow_forwardSolve in MATLABarrow_forwardHello please look at the attached picture. I need an detailed explanation of the architecturearrow_forward
- Information Security Risk and Vulnerability Assessment 1- Which TCP/IP protocol is used to convert the IP address to the Mac address? Explain 2-What popular switch feature allows you to create communication boundaries between systems connected to the switch3- what types of vulnerability directly related to the programmer of the software?4- Who ensures the entity implements appropriate security controls to protect an asset? Please do not use AI and add refrencearrow_forwardFind the voltage V0 across the 4K resistor using the mesh method or nodal analysis. Note: I have already simulated it and the value it should give is -1.714Varrow_forwardResolver por superposicionarrow_forward
- Describe three (3) Multiplexing techniques common for fiber optic linksarrow_forwardCould you help me to know features of the following concepts: - commercial CA - memory integrity - WMI filterarrow_forwardBriefly describe the issues involved in using ATM technology in Local Area Networksarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningNew Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
- Systems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
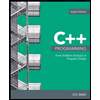
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
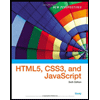
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:9781305503922
Author:Patrick M. Carey
Publisher:Cengage Learning
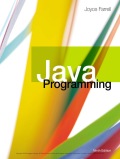
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
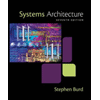
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning
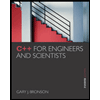
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage