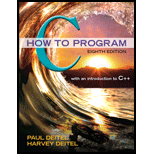
C How to Program (8th Edition)
8th Edition
ISBN: 9780133976892
Author: Paul J. Deitel, Harvey Deitel
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
Chapter 21, Problem 21.15E
a.
Program Plan Intro
- Declare variable xCoordinate and yCoordinate of type int as private member of class.
- Provide declaration of ostream and istream operator as friend function.
Program Description: To create point class having two integer data membersxcoordinate and ycoordinate and provide declaration for stream insertion and stream extraction overloaded operator functions.
b.
Program Plan Intro
Program Plan:
- Define overloaded insertion operator <<. Inside the definition use an if-else statement to check if the failbit was set or not.
- If not then display the value of xCoordinate and yCoordinate data members of point class else display the message that wrong input was entered.
- Also inside the else part use call clear function of clear the failbit.
- Define overloaded extraction operator>>. Inside the definition use ignore function to ignore the first bracket, then read the first interger value , then again use ignore to ignore the comma and extra space, next read the ycoordinatevalue and finally use ignore to skip the last closing bracket.
Program Description: To implement the overloaded insertion and extraction functions of class point in file point.cpp.
c.
Program Plan Intro
Program Plan:
- Include header file point.h
- Declare a variable A of type Point.
- Use cin statement to read the value of point in variable A.
- Use cout statement to display the value of variable A if correct value was entered.
Program Description: To write a driver program to test the Point class.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Program language: C++
A publishing company markets both hardcopy and eBook versions of its work. Create a class publication that stores the title and price of a publication. From this class derive two classes: book, which adds a page count, and digital, which adds a storage capacity in MG bytes. Each of these classes should have a getdata() function to get its data from the user at the keyboard and put a putdata() function to display its data.
Add a base class sales that hold an array of three floats so that it can record the dollar sales of a particular publication for the last three months. Include a getData() function to get three sales amounts from the user and a putdata() function to display the sales figures. Modify the book and digital classes so they are derived from both publication and sales. An object of the class book or digital should input and output sales data along with its other data. Write the main function to create a book object and a digital object and test their…
- Create a struct called Complex for performing arithmetic with complex numbers. Write a driver program to test your struct. Complex numbers have the form: realPart + imaginaryPart * iwhere i is the square root of -1Use double variables to represent data of the struct. Provide a function that enables an object of this struct to be initialized when it is declared. The function should contain default values in case no initializers are provided. Also provide functions for each of the following:a) Addition of two Complex numbers: The real parts are added together and the imaginary parts are added together.b) Subtraction of two Complex numbers: The real part of the right operand is subtracted from the real part of the left operand and the imaginary part of the right operand is subtracted from the imaginary part of the left operand.c) Printing Complex numbers in the form (a, b) where a is the real part and b is the imaginary partSubmit one file which contains all code above: the structure…
Write in C++ Language.
(Employee Record): Create a class named 'Staff' having the following members: Data members - Id – Name - Phone number – Address - AgeIt also has a function named 'printSalary' which prints the salary of the staff.Two classes 'Employee' and 'Officer' inherits the 'Staff' class. The 'Employee' and 'Officer' classes have data members 'Top Skill' and 'department' respectively. Now, assign name, age, phone number, address and salary to an employee and a officer by making an object of both of these classes and print the same.
Chapter 21 Solutions
C How to Program (8th Edition)
Ch. 21 - (Write C++ Statements) Write a statement for each...Ch. 21 - (Inputting Decimal, Octal and Hexadecimal Values)...Ch. 21 - Prob. 21.8ECh. 21 - Prob. 21.9ECh. 21 - Prob. 21.10ECh. 21 - Prob. 21.11ECh. 21 - (Converting Fahrenheit to Celsius) Write a program...Ch. 21 - In some programming languages, strings are entered...Ch. 21 - Prob. 21.14ECh. 21 - Prob. 21.15E
Knowledge Booster
Similar questions
- C++ programming Language Write a program that converts a number entered in Roman numerals todecimal form. Program should consist of a class, say romanType. Anobject of romanType should do the following:a. Store the number as a Roman numeral.b. Convert and store the number into decimal form.c. Print the number as a Roman numeral or decimal number as requested by the user. (Write two separate functions—one to print the number as aRoman numeral and the other to print the number as a decimal number.)The decimal values of the Roman numerals are:M 1000D 500C 100L 50X 10V 5I 1Remember, a larger numeral preceding a smaller numeral means addition,so LX is 60. A smaller numeral preceding a larger numeral means subtraction, so XL is 40. Any place in a decimal number, such as the 1s place, the10s place, and so on, requires from zero to four Roman numerals. (The program must include implementation files, .cpp and .h )arrow_forward#include <iostream> #include <fstream> using namespace std; /* This program declares a class called Inventory that has itemnNumber (which contains the id number of a product) and numOfItem (which contains the quantity on hand of the corresponding product)as private data members.The program will read these values from a file and store them in an array of objects (of type Inventory). It will then print these values to the screen. Example: Given the following data file: 986 8 432 24 This program reads these values into an array of objects and prints the following: Item number 986 has 8 items in stock Item number 432 has 24 items in stock */ const NUMOFPROD = 10; // This holds the number of products a store sells class Inventory {public: void getId(int item); // This puts item in the private data member // itemNumber of the object that calls it. void getAmount(int num); // This puts num in the private data member // numOfItem of the object that calls it.…arrow_forwardPROGRAMMING LANGUAGE: C++ You need to store hiring date and date of birth for teachers, and for students store their admission date and date of birth. You need to create a Date class for this purpose. Create objects of Date class in Teacher and Student class to store respective dates. You need to write print function in Teacher and Student classes as well to print all information of Teachers and Students. You need to perform composition to implement this task. Create objects of Teacher and Student classes in main function and call print function for both objects. Print Date class here. Print updated Teacher class here. Print updated Student class here. Print main function here. Print Outputshere.arrow_forward
- Select the statement that is false regarding file guarding. Group of answer choices: In your .h files all code should be contained within the guards. It restricts the scope of a namespace, which prevents namespace pollution. If used properly it prevents redefinitions of classes/functions. It is an instruction to the preprocessor. It must make use of a unique identifier.arrow_forwardhelp with c++ paste indented code plzz Have to use given main function to test your answer Q2: Continue with House class:a) Copy the previous program to a new file.b) Write Constructor with two parameter, and assign to location, and price.c) Write default constructor, initialize location to “TBD”, price to 0, Implement constructor delegationd) implement a non-member function names output that will print all information of House object. Use following main() to test your class. int main(){House a("1234 qcc st, Bayside, NY",1000000); output(a); House b; output(b); } Output from given main: Location: 1234 qcc st, Bayside, NY Price: 1000000 Location: TBD Price: 0\ ANSWER:arrow_forward1- When making a class instance, the default constructor of its fields is invoked. (True or False) 2- An uninitialized pointer is 3- If the following lines of code have errors, correct them; otherwise, write "no C++ errors." int *x = new int; *x = 5; cout<arrow_forwardHELP WITH C++ PASTE INDNETED CODE PLZZ YOU HAVE TO USE THE GIVEN MAIN FUNCTION TO TEST YOUR ANSWER Q4: Continue with House class:a) Copy the previous program to a new file. Implement comparison operator overload for comparing the price of House object. b) Overload the operator > to output boolean, which support auto type conversion. c) Overload the operator <= to output boolean, which support auto type conversion. d) Overload the operator == to output boolean, which support auto type conversion. e) Overload the operator != to output boolean, which support auto type conversion. (Hint: need one argument constructor) Use following main() to test your class. int main(){House a("1234 qcc st, Bayside, NY",1000000); House b("5678 cuny st, Bayside, NY",900000); cout<<(a>b)<<endl; // print 1 cout<<(a<=b)<<endl; // print 0 cout<<(a==b)<<endl; // print 0 cout<<(b!=a)<<endl; // print 1…arrow_forwardX15: inOrder Write a function in Java that implements the following logic: Given three ints, a, b , and c,return true if b is greater than a, and c is greater than b.However, with the exception that if bok is true, b does not need to be greater than a.arrow_forwardResearch assistants provide support to professionals who are conducting experiments or gathering andanalyzing information and data. Suppose you are working as RA (research assistant) with a Professor who isworking on a project and evaluating complex mathematical equations. Your duty is to assist him, so in thisregard he has assigned a task to you. Your task is to write a oop c++ program to create a class named equation which will have the data members a, b and c which are the coefficients of the quadratic equation. The class will have two more data members namely proot and nroot which stand for the positive root and negative root of the equation. Suppose that variables a, b and c are integers. Where proot and nroot are floats. Input Function to get values of a, b and c Then design a friend function which will determine the proot and nroot of the equation. Create another friend function which will display the values of proot and nroot.arrow_forward: You have to write a program to store information of university students. There can be many students in university, so you can make a Student class and objects of class Student to store information. The university is interested in storing information about student’s name, enrollment, department, class, CGPA and gender. You can store this information as data member values for each object. But as you already know you cannot directly access data members of a class, so you need to make member functions to access data members. You can make as many member functions as you want. There are no restrictions. The University is interested in knowing the student’s name with highest CGPA in a specific class. For example if you entered information of 10 students from class BSIT 2 and information of 5 students from class BSIT 3, and it is asked to find name of student with highest CGPA in BSIT 2 then your program must be able to find details of highest CGPA student from only BSIT 2. The university is…arrow_forward50- What does it mean for an object to be "immutable"? a. The object can't be used in calculations. b. The object can't have its value changed. c. The object can't be passed as an argument to a function. d. The value of the object can't be accessed.arrow_forwardDownload the file Ackermann.cpp. Inside the file the recursive Ackermann function is implemented (described in Chapter 14 Programming Challenge 9). Do the following and answer the three questions: a) Run the program. What happens?b) Now uncomment the code that is commented out and run the program again. What happens now?c) What do you think is going on?arrow_forwardarrow_back_iosSEE MORE QUESTIONSarrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
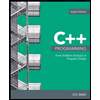
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
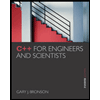
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr