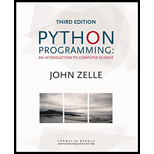
Python Programming: An Introduction to Computer Science, 3rd Ed.
3rd Edition
ISBN: 9781590282755
Author: John Zelle
Publisher: Franklin, Beedle & Associates
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 2, Problem 10PE
Program Plan Intro
Convert kilometers to miles
- Declare a main function. Inside the main function,
- Print the statement
- Get the kilometers from the user
- Calculate the distance in miles.
- Print the distance in miles.
- Call the main function.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
The images I have uploaded are the part 1 to 4 and questions below are continue on the questions uploaded
5. C++ Class Template with Method Stubs
#pragma once
#include <iostream>
#include <string>
#include <stdexcept>
#include <vector>
template <typename T>
class HashTable {
private:
struct Entry {
std::string key;
T value;
bool isOccupied;
bool isDeleted;
Entry() : key(""), value(), isOccupied(false), isDeleted(false) {}
};
Entry* table;
size_t capacity;
size_t size;
double loadFactorThreshold;
size_t customHash(const std::string& key) const {
size_t hash = 5381;
for (char c : key) {
hash = ((hash << 5) + hash) + c;
}
return hash;
}
size_t probe(const std::string& key, bool forInsert = false) const;
void resize();
public:
// Constructor
HashTable(size_t initialCapacity = 101);
// Big…
this project is NOT for graded(marks) purposes, please help me with the introduction. give me answers for the project. i will include an image explaining everything about the project.
Java Graphics
(Bonus
In this lab, we'll be practicing what we learned about GUIs, and Mouse events. You will need to
implement the following:
A GUI with a drawing panel. We can click in this panel, and you will capture those clicks as a
Point (see java.awt.Point) in a PointCollection class (you need to build this).
The points need to be represented by circles.
Below the drawing panel, you will need 5 buttons:
O
о
о
○
An input button to register your mouse to the drawing panel.
A show button to paint the points in your collection on the drawing panel.
A button to shift all the points to the left by 50 pixels.
The x position of the points is not allowed to go below zero.
Another button to shift all the points to the right 50 pixels.
"
The x position of the points cannot go further than the
You can implement this GUI in any way you choose. I suggest using the BorderLayout for a panel
containing the buttons, and a GridLayout to hold the drawing panel and button panels.
Regardless of how…
Chapter 2 Solutions
Python Programming: An Introduction to Computer Science, 3rd Ed.
Ch. 2 - Prob. 1TFCh. 2 - Prob. 2TFCh. 2 - Prob. 3TFCh. 2 - Prob. 4TFCh. 2 - Prob. 5TFCh. 2 - Prob. 6TFCh. 2 - Prob. 7TFCh. 2 - Prob. 8TFCh. 2 - Prob. 9TFCh. 2 - Prob. 10TF
Ch. 2 - Prob. 1MCCh. 2 - Prob. 2MCCh. 2 - Prob. 3MCCh. 2 - Prob. 4MCCh. 2 - Prob. 5MCCh. 2 - Prob. 6MCCh. 2 - Prob. 7MCCh. 2 - Prob. 8MCCh. 2 - Prob. 9MCCh. 2 - Prob. 10MCCh. 2 - Prob. 1DCh. 2 - Prob. 3DCh. 2 - Prob. 4DCh. 2 - Prob. 5DCh. 2 - Prob. 6DCh. 2 - Prob. 7DCh. 2 - Prob. 1PECh. 2 - Prob. 2PECh. 2 - Prob. 3PECh. 2 - Prob. 4PECh. 2 - Prob. 5PECh. 2 - Prob. 6PECh. 2 - Prob. 7PECh. 2 - Prob. 8PECh. 2 - Prob. 9PECh. 2 - Prob. 10PECh. 2 - Prob. 11PECh. 2 - Prob. 12PE
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- also provide the number of moves(actions) made at state A and moves(actions) made state B. INCLUDE Java program required(this question is not graded)arrow_forwardYou are given a class that processes purchases for an online store. The class receives calls to: • Retrieve the prices for items from a database • Record the sold items • Update the database • Refresh the webpage a. What architectural pattern is suitable for this scenario? Illustrate your answer by drawing a model for the solution, showing the method calls/events. b. Comment on how applying this pattern will impact the modifiability of the system. c. Draw a sequence diagram for the update operation.arrow_forward2. The memory management has contiguous memory allocation, dynamic partitions, and paging. Compare the internal fragmentation and external fragmentation for these three approaches. [2 marks] 3. Suppose we have Logical address space = 24 = 16 (m = 4), Page size=2² =4 (n = 2), Physical address space = 26 = 64 (r = 6). Answer the following questions: [4 marks] 1) Total # of pages ? 2) Total # of frames ? 3) Number of bits to represent logical address? 4) Number of bits to represent offset ? 5) Number of bits to represent physical address? 6) Number of bits to represent a page number? 7) Number of bits to represent a frame number / 4. What is translation look-aside buffers (TLBS)? Why we need them to implement the page table? [2 marks] 5. Why we need shared pages for multiple processes? Give one example to show the benefits. [2 marks] 6. How to implement the virtual memory by using page out and page in? Explain with an example. [2 marks] 7. We have a reference string of referenced page…arrow_forward
- Good morning, please solve this trying to follow this criteria. (use Keil) Abstract describing the requirements and goals of the assignment. List file with no errors or warnings. Brief description of your implementation design and code. Debugging screen shots for different scenarios with your reference and comments. Conclusion (and please give me the code without errors, make sure it is working)arrow_forwardGood mrng, please solve this trying to follow this criteria. (use Keil) Abstract describing the requirements and goals of the assignment. List file with no errors or warnings. Brief description of your implementation design and code. Debugging screen shots for different scenarios with your reference and comments. Conclusion (and please give me the code without errors, make sure it is working)arrow_forward#include <stdio.h> #include <stdlib.h> #include <unistd.h> int global_var = 42; // int* function(int *a) { int local_var = 10; // *a = *a + local_var; int *local_pointer = (int *)malloc (size of (int) * 64); // Allocated array with 64 integers return local_pinter; } int main() { int local_main[1024*1024*1024*1024*1024] = {0}; // initialize an array and set all items as 0 int *heap_var = (int *)malloc(size of(int) * 16); // Allocated array with 16 integers *heap_var = 100; function(heap_var); printf(“the value is %d\n”, *heap_var); free(heap_var); // release the memory return 0; } 1) draw the memory layout of the created process, which should include text, data, heap and stack [2 marks]. 2) Indicate which section the variables are allocated [2 marks]: global_var local_var, local_pointer local_main heap_var, *heap_var (the data that it points to) 3) Does this code have memory leaking (heap memory is not released)? [2 marks] 4) The…arrow_forward
- 8. List three HDD scheduling algorithms. [2 marks] 9. True or False? The NVM has the same scheduling algorithms with HDD. Explain why? [2 marks] 10. Why the modern mouses use polling to detect movements instead of interrupts? [2 marks] 11. What is thrashing? How does it happen? [2 marks] 12. Given a reference string of page numbers 7, 0, 1, 2, 0, 3, 0, 4, 2, 3, 0, 3, 0, 3, 2, 1, 2, 0, 1, 7, 0, 1 and 4 frames show how the page replacement algorithms work, and how many page faults? [6 marks], 1) FIFO algorithm? [2 marks] 2) Optimal algorithm? [2 marks] 3) LRU algorithm? [2 marks] 13. List at least three file systems that you know. [2 marks] 14. In C programming, how the seek to a specific position in the file by offset? [2 marks]arrow_forwardA Personal Address Book application program allows the user to add, delete, search, save, and load contact information. The program separates the user interface (command-line) and the internal processing subsystem. The internal processing system consists of the following classes: • • Contact Manager (responsible for add and delete operations) Contact Finder (responsible for the search operation) pataManager (responsible for save and load operations) a. What design pattern can be used to implement the user interface? Explain your answer using a class diagram for the entire system. b. Draw a UML sequence diagram to show the behavioral view of the Personal Address Book program, demonstrating what happens when a user enters new contact information.arrow_forwardA system comprises three components: A, B, and C. Calling A requires calling B, and calling B requires calling A. Component C is responsible for dissimilar tasks T#1, #2, and T#3. a. Comment on the modifiability of this system. What problems do you see in this system, and how would you solve them? b. Suppose that T#1 is performed by both component A and C. What does this indicate about A and C? How would you solve this problem?arrow_forward
- Please answer questions from number 1 to 3 if these questions in the image provided below(NOTE: THESE QUESTIONS ARE NOT GRADED!)arrow_forwardIn a client-server system for a hypothetical banking application, the clients are ATM machines, and the server is the bank server. Suppose the client has two operations: withdraw and deposit. Use the broker architectural pattern to document an initial architecture design for this system: a. Draw a class diagram for this system. Use a client-side proxy to encrypt the data using an encrypt operation and a server-side proxy to decrypt the data. b. Discuss how you plan to apply redundancy tactics on the server. Additionally, identify the quality attribute that this tactic will achieve and discuss any potential side effects of applying it.arrow_forwarda. Comment on how you would achieve higher performance for a hypothetical Trent Course Registration System, assuming it utilizes a client-server architecture. b. Suppose we want greater availability of the server. Discuss what kind of tactics should be used to achieve that.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
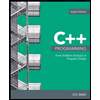
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
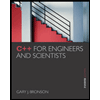
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
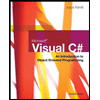
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
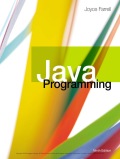
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
Constants, Variables, Data types, Keywords in C Programming Language Tutorial; Author: LearningLad;https://www.youtube.com/watch?v=d7tdL-ZEWdE;License: Standard YouTube License, CC-BY