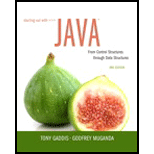
Starting Out with Java: From Control Structures through Data Structures (3rd Edition)
3rd Edition
ISBN: 9780134038179
Author: Tony Gaddis, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 19, Problem 8PC
Program Plan Intro
groupingBy Collector
- Import the required packages.
- Create a class “ReduceToMapDemo1”.
- Define the “main” method.
- Create a stream “names” for reducing to map.
- Create a key “KeyMapper” for a string. Key for string is the length of the string.
- Reduce the stream and get the map using “groupingBy()” method and the key “keymapper”.
- Print the map.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
OCaml Code: The goal of this project is to understand and build an interpreter for a small, OCaml-like, stackbased bytecode language. Make sure that the code compiles correctly and provide the code with the screenshot of the output. The code must have a function that takes a pair of strings (tuple) and returns a unit. You must avoid this error that is attached as an image. Make sure to have the following methods below:
-Push integers, strings, and names on the stack
-Push booleans
-Pushing an error literal or unit literal will push :error: or :unit:onto the stack, respectively
-Command pop removes the top value from the stack
-The command add refers to integer addition. Since this is a binary operator, it consumes the toptwo values in the stack, calculates the sum and pushes the result back to the stack
- Command sub refers to integer subtraction
-Command mul refers to integer multiplication
-Command div refers to integer division
-Command rem refers to the remainder of integer…
RUBY
Implement the Plates class buildMap function so that it populates the HashMap with the state abbreviations as keys and the counts of how many each appear in the file as values.
Sometimes, the parking attendant will add special notation to help her remember something about a specific entry. There are just non alphabetic characters that she adds to the state - your program should ignore these characters so that an entry like NY* still counts toward the NY plate count.
She is also very inconsistent with how she enters the plates. Sometimes she uses upper case, sometimes lowercase, and sometimes she even uses a mix. Be sure to account for this in your program.
Only add information for plates in New England (Maine, New Hampshire, Vermont, Massachusetts, Rhode Island, and Connecticut).
Plates.java
import java.util.Arrays;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Map;
import java.util.Set;
public class Plates {
private Map<String, Integer> plateMap;…
Chapter 19 Solutions
Starting Out with Java: From Control Structures through Data Structures (3rd Edition)
Ch. 19.1 - Prob. 19.1CPCh. 19.1 - What are the three general types of collections?Ch. 19.1 - Prob. 19.3CPCh. 19.1 - Prob. 19.4CPCh. 19.1 - Prob. 19.5CPCh. 19.1 - Prob. 19.6CPCh. 19.1 - Prob. 19.7CPCh. 19.2 - Prob. 19.8CPCh. 19.2 - Prob. 19.9CPCh. 19.2 - Prob. 19.10CP
Ch. 19.2 - Prob. 19.11CPCh. 19.2 - Prob. 19.12CPCh. 19.2 - Prob. 19.13CPCh. 19.2 - Prob. 19.14CPCh. 19.2 - Prob. 19.16CPCh. 19.2 - Prob. 19.17CPCh. 19.2 - Prob. 19.18CPCh. 19.2 - Prob. 19.20CPCh. 19.3 - Prob. 19.21CPCh. 19.3 - Prob. 19.22CPCh. 19.3 - Prob. 19.23CPCh. 19.3 - Prob. 19.24CPCh. 19.3 - Any time you override the Object classs equals...Ch. 19.3 - Prob. 19.26CPCh. 19.3 - Prob. 19.27CPCh. 19.3 - Prob. 19.28CPCh. 19.4 - Prob. 19.29CPCh. 19.4 - Prob. 19.31CPCh. 19.4 - Prob. 19.32CPCh. 19.6 - How do you define a stream of elements?Ch. 19.6 - How does a stream intermediate operation differ...Ch. 19.6 - Prob. 19.35CPCh. 19.6 - Prob. 19.36CPCh. 19.6 - Prob. 19.37CPCh. 19.6 - Prob. 19.38CPCh. 19.6 - Prob. 19.39CPCh. 19 - Prob. 1MCCh. 19 - Prob. 2MCCh. 19 - This type of collection is optimized for...Ch. 19 - Prob. 4MCCh. 19 - A terminal operation in a stream pipeline is also...Ch. 19 - Prob. 6MCCh. 19 - Prob. 7MCCh. 19 - This List Iterator method replaces an existing...Ch. 19 - Prob. 9MCCh. 19 - Prob. 10MCCh. 19 - This is an object that can compare two other...Ch. 19 - This class provides numerous static methods that...Ch. 19 - Prob. 13MCCh. 19 - Prob. 14MCCh. 19 - Prob. 15TFCh. 19 - Prob. 16TFCh. 19 - Prob. 17TFCh. 19 - Prob. 18TFCh. 19 - Prob. 19TFCh. 19 - Prob. 20TFCh. 19 - Prob. 21TFCh. 19 - Prob. 22TFCh. 19 - Prob. 1FTECh. 19 - Prob. 2FTECh. 19 - Prob. 3FTECh. 19 - Prob. 4FTECh. 19 - Write a statement that declares a List reference...Ch. 19 - Prob. 2AWCh. 19 - Assume that it references a newly created iterator...Ch. 19 - Prob. 4AWCh. 19 - Prob. 2SACh. 19 - Prob. 4SACh. 19 - Prob. 5SACh. 19 - Prob. 6SACh. 19 - How does the Java compiler process an enhanced for...Ch. 19 - Prob. 8SACh. 19 - Prob. 9SACh. 19 - Prob. 10SACh. 19 - Prob. 11SACh. 19 - Prob. 12SACh. 19 - Prob. 13SACh. 19 - Prob. 14SACh. 19 - Word Set Write an application that reads a line of...Ch. 19 - Prob. 3PCCh. 19 - Prob. 5PCCh. 19 - Prob. 8PC
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- A programmer is designing a program to catalog all books in a library. He plans to have a Book class that stores features of each book: author, title, isOnShelf, and so on, with operations like getAuthor, getTitle, getShelf Info, and set Shelf Info. Another class, LibraryList, will store an array of Book objects. The LibraryList class will include operations such as listAll Books, addBook, removeBook, and searchForBook. The programmer plans to implement and test the Book class first, before implementing the LibraryList class. The program- mer's plan to write the Book class first is an example of (A) top-down development. (B) bottom-up development. (C) procedural abstraction. (D) information hiding. (E) a driver program.arrow_forwardIn C++, Write the implementation for the methods of the Node class including the constructor. The constructor should set value to the passed in parameter, and set the value of the next pointer to NULL. getNext() should return the value of the next pointer. setNext() should set the value of the next pointer to the parameter that is passed in. In the main() function below (where it says // Step 2 code here), write the code to make a list of 10 Node objects. To do this, make a root pointer which is a pointer to a Node object, and then use a loop to create new Nodes, hooking up the list using setNext() and getNext() for each node. Set the value of each new Node to be the even numbers 2 through 18 (in addition to the root node which has value 0). Make sure that your nodes are linked together! Write code to step through your linked list (where it says // Step 3 code here), printing the value of each node. Write code to clean up the list (where it says // Step 4 code here). Print a…arrow_forwardThis is for python For this assignment, you will write a program to simulate a payroll application. To that effect, you will also create an Employee class, according to the specifications below. Since an Employee list might be large, and individual Employee objects may contain significant information themselves, we store the Employee list as a linked list. Employee class: Attributes • Employee ID: you can use a string – the ID will contain digits and/or hyphens. The ID must be provided during object construction and there should be no mechanism to change it later. • Number of hours worked in a week: a floating-point number. • Hourly pay rate: a floating-point number that represents how much the employee is paid for one hour of work. • Gross wages: a floating-point number that stores the number of hours times the hourly rate. Methods • A constructor (__init__) • Setter methods as needed. • Getter methods as needed. • This class should overload the __str__ or __repr__ methods so that…arrow_forward
- Written in Python with docstring please if applicable Thank youarrow_forwardThe play method in the Player class of the craps game plays an entire game without interaction with the user. Revise the Player class so that its user can make individual rolls of the dice and view the results after each roll. The Player class no longer accumulates a list of rolls, but saves the string representation of each roll after it is made. Add new methods rollDice, getNumberOfRolls, isWinner, and isLoser to the Player class. The last three methods allow the user to obtain the number of rolls and to determine whether there is a winner or a loser. The last two methods are associated with new Boolean instance variables (winner and loser respectively). Two other instance variables track the number of rolls and the string representation of the most recent roll (rollsCount and roll). Another instance variable (atStartup) tracks whether or not the first roll has occurred. At instantiation, the roll, rollsCount, atStartup, winner, and loser variables are set to their appropriate…arrow_forwardOCaml Code: The goal of this project is to understand and build an interpreter for a small, OCaml-like, stackbased bytecode language. Make sure that the code compiles correctly without any errors and provide the code with the screenshots of the outputs. The code must have a function that takes a pair of strings (tuple) and returns a unit. Make sure to have the following methods below: -Push integers, strings, and names on the stack -Push booleans -Pushing an error literal or unit literal will push :error: or :unit: onto the stack, respectively -Command pop removes the top value from the stack -The command add refers to integer addition. Since this is a binary operator, it consumes the top two values in the stack, calculates the sum and pushes the result back to the stack - Command sub refers to integer subtraction where if top two elements in the stack are integer numbers, pop the top element(y) and the next element(x), subtract y from x, and push the result x-y back onto the stack…arrow_forward
- I need to create a java program without using any java tools, just the java scanner.arrow_forwardImport the ArrayList and List classes from the java.util package to create a list of phone numbers and also import the HashSet and Set classes from the java.util package to create a set of unique prefixes. Create a class called PhoneNumberPrefix with a main method that will contain the code to find the unique prefixes. Create a List called phoneNumbers and use the add method to add several phone numbers to the list. List<String> phoneNumbers = new ArrayList<>(); phoneNumbers.add("555-555-1234"); phoneNumbers.add("555-555-2345"); phoneNumbers.add("555-555-3456"); phoneNumbers.add("444-444-1234"); phoneNumbers.add("333-333-1234"); Create a Set called prefixes and use a for-each loop to iterate over the phoneNumbers list. For each phone number, we use the substring method to extract the first 7 characters, which represent the prefix, and add it to the prefixes set using the add method. Finally, use the println method to print the prefixes set, which will contain all of…arrow_forwardJava programming homework Please help me with question 13.30arrow_forward
- python: def typehelper(poke_name): """ Question 5 - API Now that you've acquired a new helper, you want to take care of them! Use the provided API to find the type(s) of the Pokemon whose name is given. Then, for each type of the Pokemon, map the name of the type to a list of all types that do double damage to that type. Note: Each type should be considered individually. Base URL: https://pokeapi.co/ Endpoint: api/v2/pokemon/{poke_name} You will also need to use a link provided in the API response. Args: Pokemon name (str) Returns: Dictionary of types Hint: You will have to run requests.get() multiple times! >>> typehelper("bulbasaur") {'grass': ['flying', 'poison', 'bug', 'fire', 'ice'], 'poison': ['ground', 'psychic']} >>> typehelper("corviknight") {'flying': ['rock', 'electric', 'ice'], 'steel': ['fighting', 'ground', 'fire']} """ # pprint(typehelper("bulbasaur"))#…arrow_forwardContainers that support random-access iterators can be used with most but not all Standard Library algorithms. What is the exception?arrow_forwardProvide me complete and correct solution thanks 3arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
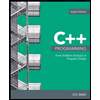
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning