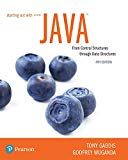
Explanation of Solution
Method definition for “reverse()”:
The method definition for “reverse()” is given below:
/* Method definition for "reverse()" */
public void reverse()
{
//Create a list "rev_list" and set it to "null"
Node rev_list = null;
/* This loop will perform up to the first node equals to "null" */
while (first != null)
{
/* Move a node to rev_list from what leftovers of the original list */
/* Set a reference "ref" to first node */
Node ref = first;
/* Set "first" to next first node */
first = first.next;
/* Set next node to "rev_list" */
ref.next = rev_list;
/* Set the "rev_list" to reference "ref" */
rev_list = ref;
}
/* Make the list "rev_list" as the new list */
first = rev_list;
}
Explanation:
From the above method definition,
- Create a list “rev_list” and then set it to “null”.
- Performs “while” loop. This loop will perform up to the first node becomes “null”.
- Set a reference “ref” to first node.
- Set “first” to next value of “first”.
- Assign next node to “rev_list”.
- Assign the “rev_list” to reference “ref”.
- Finally make the list “rev_list” as new list.
Complete code:
The complete executable code for “reverse()” method is given below:
//Define "LinkedList1" class
class LinkedList1
{
/* The code for this part is same as the textbook of "LinkedList1" class */
/* Method definition for "reverse()"*/
public void reverse()
{
//Create a list "rev_list" and set it to "null"
Node rev_list = null;
/* This loop will perform up to the first node equals to "null" */
while (first != null)
{
/* Move a node to rev_list from what remains of the original list */
/* Set a reference "ref" to first node */
Node ref = first;
/* Set "first" to next first node */
first = first...

Want to see the full answer?
Check out a sample textbook solution
Chapter 19 Solutions
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
- 4. def modify_data(x, my_list): X = X + 1 my_list.append(x) print(f"Inside the function: x = {x}, my_list = {my_list}") num = 5 numbers = [1, 2, 3] modify_data(num, numbers) print(f"Outside the function: num = {num}, my_list = {numbers}") Classe Classe that lin Thus, A pro is ref inter Ever dict The The output: Inside the function:? Outside the function:?arrow_forwardpython Tasks 5 • Task 1: Building a Library Management system. Write a Book class and a function to filter books by publication year. • Task 2: Create a Person class with name and age attributes, and calculate the average age of a list of people Task 3: Building a Movie Collection system. Each movie has a title, a genre, and a rating. Write a function to filter movies based on a minimum rating. ⚫ Task 4: Find Young Animals. Create an Animal class with name, species, and age attributes, and track the animals' ages to know which ones are still young. • Task 5(homework): In a store's inventory system, you want to apply discounts to products and filter those with prices above a specified amount. 27/04/1446arrow_forwardOf the five primary components of an information system (hardware, software, data, people, process), which do you think is the most important to the success of a business organization? Part A - Define each primary component of the information system. Part B - Include your perspective on why your selection is most important. Part C - Provide an example from your personal experience to support your answer.arrow_forward
- Management Information Systemsarrow_forwardQ2/find the transfer function C/R for the system shown in the figure Re དarrow_forwardPlease original work select a topic related to architectures or infrastructures (Data Lakehouse Architecture). Discussing how you would implement your chosen topic in a data warehouse project Please cite in text references and add weblinksarrow_forward
- Please original work What topic would be related to architectures or infrastructures. How you would implement your chosen topic in a data warehouse project. Please cite in text references and add weblinksarrow_forwardWhat is cloud computing and why do we use it? Give one of your friends with your answer.arrow_forwardWhat are triggers and how do you invoke them on demand? Give one reference with your answer.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
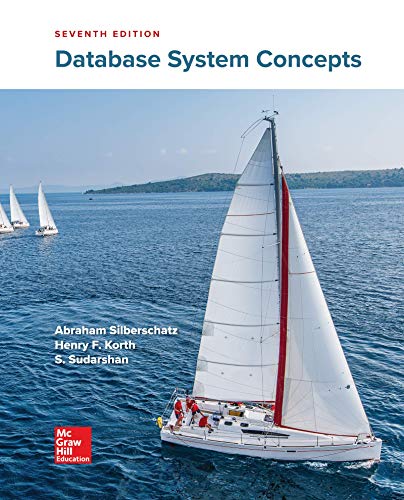
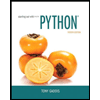
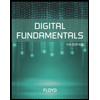
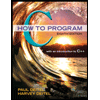
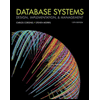
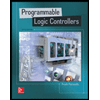