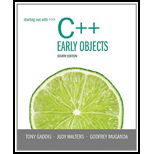
Concept explainers
File Filter
A file filter reads an input file, transforms it in some way, and writes the results to an output file. Write an abstract file filter class that defines a pure virtual function for transforming a character. Create one subclass of your file filter class that performs encryption, another that transforms a file to all uppercase, and another that creates an unchanged copy of the original file.
The class should have a member function
void doFilter(ifstream &in, ofstream &out)
that is called to perform the actual filtering. The member function for transforming a single character should have the prototype
char transform(char ch)
The encryption class should have a constructor that takes an integer as an argument and uses it as the encryption key.

Want to see the full answer?
Check out a sample textbook solution
Chapter 15 Solutions
Starting Out with C++: Early Objects
Additional Engineering Textbook Solutions
Java How to Program, Early Objects (11th Edition) (Deitel: How to Program)
Java: An Introduction to Problem Solving and Programming (8th Edition)
Web Development and Design Foundations with HTML5 (8th Edition)
Degarmo's Materials And Processes In Manufacturing
Mechanics of Materials (10th Edition)
Starting Out with C++ from Control Structures to Objects (9th Edition)
- The functionality of each file is described below:Class BankAccount This class has two instance variables:namebalanceCreate a constructor that takes two parameters to initialize the instance variables name and balance. The name must not be an empty string. The name also must not include any numbers or symbols. The balance must be zero or more to be used.If the name was an empty string a Value Error will be raised with the message “name cannot be empty”If the name contained numbers or symbols a Value Error will be raised with the message “name cannot include numbers or symbols”If the name was valid, it will be formatted properly and assigned to the instance variable. The valid name consists of alphabetical characters lowercase or upper case, may include space, underscore or hyphen.The name may consist of first, middle and last name.One way to validate the name is using Regular Expressions.Proper name format is the first character uppercase and the rest of the name lowercase character.…arrow_forwardAssignment Submission Instructions:This is an individual assignment – no group submissions are allowed. Submit a script file that contains the SELECT statements by assigned date. The outline of the script file lists as follows:/* ******************************************************************************** * Name: YourNameGoesHere * * Class: CST 235 * * Section: * * Date: * * I have not received or given help on this assignment: YourName * ***********************************************************************************/USE RetailDB;####### Tasks: Write SQL Queries ######### -- Task 1 (Customer Information):-- List your SELECT statement below. Make sure the SQL script file can be run successfully in MySQL and show the outcome of the code on MySQLarrow_forwardPROGRAMMING LANGUAGE: C++ Implement another class with name UniversityClass which is composed of one teacher and multiple students(use student array) as data members. Another data member of UniversityClass is CourseName that is just a string data member. Write a function in UniversityClass to take Teacher Name, Student names and course name from user. Insert these records in a text file and print screenshot of that text file. Print UniversityClass code here. Print main function here. Print screenshot of text file here.arrow_forward
- C++ Struct Pointers Help: I have a file called names.txt. Write a program that reads each line and then store the name and nickname under an individual pointer to the class Person. names.txt: Norman, Normie Justine, Jussy Richard, Dick Shelley, Shell class Person { public: string name; string nickname; Person(string name, nickname) { this->name=name; this->nickname = nickname; } }; Print out each pointer's name and nickname to verify that it has been stored.arrow_forwardWrite a program that contains topics Inheritance Operator Overloading Vector Template Exception Handling File Stream Friend function and Friend classarrow_forwardCLASSES, DYNAMIC ARRAYS AND POINTERS Define a class called textLines that will be used to store a list of lines of text (each line can be specified as a string). Use a dynamic array to store the list. In addition, you should have a private data member that specifies the length of the list. Create a constructor that takes a file name as parameter, and fills up the list with lines from the file. Make sure that you set the dynamic array to expand large enough to hold all the lines from the file. Also, create a constructor that takes an integer parameter that sets the size of an empty list. in C++ Write member functions to: remove and return the last line from the list add a new line onto the end of the list, if there is room for it, otherwise print a message and expand the array empty the entire list return the number of lines still on the list take two lists and return one combined list (with no duplicates) copy constructor to support deep copying remember the destructor!arrow_forward
- Program language: C++ A publishing company markets both hardcopy and eBook versions of its work. Create a class publication that stores the title and price of a publication. From this class derive two classes: book, which adds a page count, and digital, which adds a storage capacity in MG bytes. Each of these classes should have a getdata() function to get its data from the user at the keyboard and put a putdata() function to display its data. Add a base class sales that hold an array of three floats so that it can record the dollar sales of a particular publication for the last three months. Include a getData() function to get three sales amounts from the user and a putdata() function to display the sales figures. Modify the book and digital classes so they are derived from both publication and sales. An object of the class book or digital should input and output sales data along with its other data. Write the main function to create a book object and a digital object and test their…arrow_forwardPut the class definition in EV.h and the implementation of the constructors and functions in EV.cpp 1. Define a class called "EV", that represents the information of an electric vehicle. The EV is defined with these attributes: model (string), range(int), unit (string) and year (int). Functions of the EV class must perform the following operations: 1. A default constructor which sets all the numeric instance variables to zero. 2. A constructor with 4 parameters which sets the 4 instance variables to the corresponding values passed. 3. Implement a getter function for each of the 4 instance variables that will return the value of the instance variable. For example, the getX() function for the instance variable model must be called getModel(). 4. Implement a setter function for each instance variable that will assign to the instance variable to the value passed. For example, the setX() function for the instance variable model must be called setModel(). 5. An info function: this function…arrow_forwardC++arrow_forward
- C++ PROGRAM Create a class MyClass with a string data member ‘paragraph’. Provide a constructor, getParagraph() and display() method in the class. getParagraph() method must read a paragraph from a file. The display method should display that paragraph. Create another class, MyFriendClass and make it a friend of the class MyClass. Provide a function getWord(MyClass &obj1, int index) with return type string in the class MyFriendClass which accepts an object of class MyClass (by reference) and an integer. The getWord() function should return the word at location index passed as an argument to the data member ‘paragraph’ of the object received as input.arrow_forwardCurrent file: Encyclopedia.h Load default 1 #ifndef ENCYCLOPEDIAH 2 #define ENCYCLOPEDIAH 3 4 #include "Book.h" 6 class Encyclopedia : public Book { // TODO: Declare mutator functions - 7 8 // SetEdition(), SetNumVolumes() 9 10 // TODO: Declare accessor functions - GetEdition(), GetNumVolumes() 11 12 // 13 14 // TODO: Declare a PrintInfo() function that overrides the PrintInfo in Book class 15 16 // 17 18 19 // TODO: Declare private fields: edition, numVolumes 20 21 }; 22 23 #endi farrow_forwardAssignment 1 University Library SystemIn this assignment you are going to implement your own version of university library system,The system will have two different sides , the first one is to help the librarian to do his job and the other side for admin to manage every one permissions in the system , so You should provide the following features in your system: Admin viewo Add/remove Studentso Add/remove librariano Add/remove other admins Librarian viewo Add/Delete bookso Issue/Return bookso View bookso View Issued bookso Log in /log outo Search for the book(id/name) Simple backend for your system , You could use a file each row should represent item o Books File should be something like:Id , Book Name, Author Name, Available quantity, Issued Quantity 1,Oliver Twist, Charles Dickens,98,2In the previous the first row was the name of each column , you can do that orhandle it in your code , id =1 , Book Name =Oliver Twist ..Etc. Tables neededo Books Id Book Name Author Name…arrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
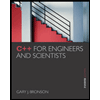
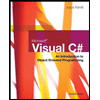