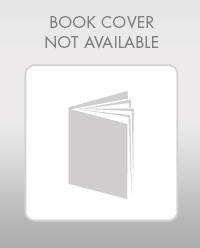
Starting Out With C++: Early Objects (10th Edition)
10th Edition
ISBN: 9780135235003
Author: Tony Gaddis, Judy Walters, Godfrey Muganda
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 14, Problem 8PC
Program Plan Intro
Palindrome Testing
Program Plan:
- Include the required header files to the program.
- Declare function prototype.
- Define the “main()” function. Inside this function,
- Do until the user enters “ENTER” key using “while” condition.
- Get a word from the user and store it in a variable “word”.
- Check if the entered word is empty.
- If the condition is true then, return 1.
- Call the function “isPalindrome()” and check if the function returns “true”.
- If the function returns true then, the entered word is a palindrome.
- Else, the entered word is not a palindrome.
- Return the statement.
- Do until the user enters “ENTER” key using “while” condition.
- Give function definition for “isPalindrome()”.
- Check if the value of “lower” is greater than “upper”.
- Return “true”.
- Check if the first and the last characters are not equal.
- Return “false”.
- Return and call the function “isPalindrome()” recursively.
- Check if the value of “lower” is greater than “upper”.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
I need help to solve a simple problem using Grover’s algorithm, where the solution is not necessarily known beforehand. The problem is a 2×2 binary sudoku with two rules:
• No column may contain the same value twice.
• No row may contain the same value twice.
Each square in the sudoku is assigned to a variable as follows:
We want to design a quantum circuit that outputs a valid solution to this sudoku. While using Grover’s algorithm for this task is not necessarily practical, the goal is to demonstrate how classical decision problems can be converted into oracles for Grover’s algorithm.
Turning the Problem into a Circuit
To solve this, an oracle needs to be created that helps identify valid solutions. The first step is to construct a classical function within a quantum circuit that checks whether a given state satisfies the sudoku rules.
Since we need to check both columns and rows, there are four conditions to verify:
v0 ≠ v1 # Check top row
v2 ≠ v3 # Check bottom row…
I need help to solve a simple problem using Grover’s algorithm, where the solution is not necessarily known beforehand. The problem is a 2×2 binary sudoku with two rules:
• No column may contain the same value twice.
• No row may contain the same value twice.
Each square in the sudoku is assigned to a variable as follows:
We want to design a quantum circuit that outputs a valid solution to this sudoku. While using Grover’s algorithm for this task is not necessarily practical, the goal is to demonstrate how classical decision problems can be converted into oracles for Grover’s algorithm.
Turning the Problem into a Circuit
To solve this, an oracle needs to be created that helps identify valid solutions. The first step is to construct a classical function within a quantum circuit that checks whether a given state satisfies the sudoku rules.
Since we need to check both columns and rows, there are four conditions to verify:
v0 ≠ v1 # Check top row
v2 ≠ v3 # Check bottom row…
I need help to solve a simple problem using Grover’s algorithm, where the solution is not necessarily known beforehand. The problem is a 2×2 binary sudoku with two rules:
• No column may contain the same value twice.
• No row may contain the same value twice.
Each square in the sudoku is assigned to a variable as follows:
We want to design a quantum circuit that outputs a valid solution to this sudoku. While using Grover’s algorithm for this task is not necessarily practical, the goal is to demonstrate how classical decision problems can be converted into oracles for Grover’s algorithm.
Turning the Problem into a Circuit
To solve this, an oracle needs to be created that helps identify valid solutions. The first step is to construct a classical function within a quantum circuit that checks whether a given state satisfies the sudoku rules.
Since we need to check both columns and rows, there are four conditions to verify:
v0 ≠ v1 # Check top row
v2 ≠ v3 # Check bottom row…
Chapter 14 Solutions
Starting Out With C++: Early Objects (10th Edition)
Ch. 14.1 - What is a recursive functions base case?Ch. 14.1 - What happens if a recursive function does not...Ch. 14.1 - Prob. 14.3CPCh. 14.1 - What is the difference between direct and indirect...Ch. 14 - What type of recursive function do you think would...Ch. 14 - Which repetition approach is less efficient; a...Ch. 14 - When should you choose a recursive algorithm over...Ch. 14 - Prob. 4RQECh. 14 - Prob. 5RQECh. 14 - Prob. 6RQE
Ch. 14 - Predict the Output 7. What is the output of the...Ch. 14 - Soft Skills 8. Programming is communication; the...Ch. 14 - Prob. 1PCCh. 14 - Recursive Conversion Convert the following...Ch. 14 - Prob. 3PCCh. 14 - Recursive Array Sum Write a function that accepts...Ch. 14 - Prob. 5PCCh. 14 - Recursive Member Test Write a recursive Boolean...Ch. 14 - Prob. 7PCCh. 14 - Prob. 8PCCh. 14 - Ackermanns Function Ackermanns function is a...Ch. 14 - Prefix to Postfix Write a program that reads...Ch. 14 - Prob. 11PCCh. 14 - Prob. 12PC
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Don't use ai to answer I will report you answerarrow_forwardYou can use Eclipse later for program verification after submission. 1. Create an abstract Animal class. Then, create a Cat class. Please implement all the methods and inheritance relations in the UML correctly: Animal name: String # Animal (name: String) + getName(): String + setName(name: String): void + toString(): String + makeSound(): void Cat breed : String age: int + Cat(name: String, breed: String, age: int) + getBreed(): String + getAge (): int + toString(): String + makeSound(): void 2. Create a public CatTest class with a main method. In the main method, create one Cat object and print the object using System.out.println(). Then, test makeSound() method. Your printing result must follow the example output: name: Coco, breed: Domestic short-haired, age: 3 Meow Meowarrow_forwardautomata theory can please wright the exact language it know for example say it knows strings start 0 and end with 1 this is as example also as regular expressionarrow_forward
- I would like help to resolve the following case, thank youarrow_forwardI need help with the following casearrow_forwardQ2) by using SHI-Tomasi detector method under the constraints shown in fig. 1 below find the corner that is usful to use in video-steganography? 10.8 ...... V...... 0.7 286 720 ke Fig.1 Threshold graph. The plain test is :Hello Ahmed the key is: 3a 2x5 5b 7c 1J 55 44 2X3 [ ] 2x3arrow_forward
- What significant justification is there for the -> operator in C and C++?arrow_forwardMultidimensional arrays can be stored in row major order, as in C++, or in column major order, as in Fortran. Develop the access functions for both of these arrangements for three-dimensional arrays.arrow_forwardWhat are the arguments for and against Java’s implicit heap storage recovery, when compared with the explicit heap storage recovery required in C++? Consider real-time systems.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage Learning
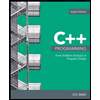
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
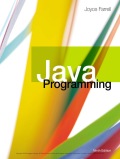
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
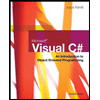
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
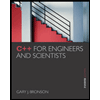
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
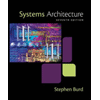
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning