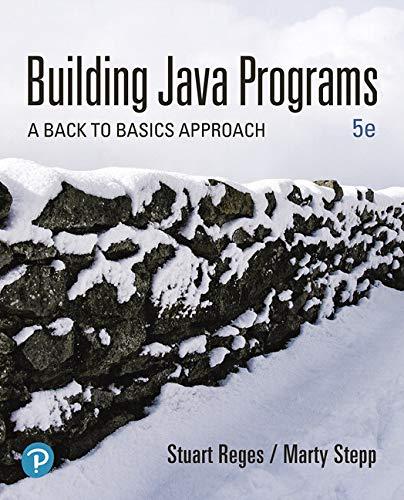
Rearrange stack of integers
Program plan:
- Import necessary packages.
- Create a class “StackSplit”,
- Define the method “splitstack ()” that accepts stack of integers,
- Construct “LinkedList” object.
- Calculate the stack size.
- Execute till stack is empty,
- Transfer all the stack elements from stack to queue.
- Execute loop till the stack size,
- Assign the element removed from the queue to the variable.
- Check whether the element is negative,
- Insert the element into the stack.
-
- Otherwise,
- Insert the element into the queue.
- Otherwise,
- Execute till queue is empty,
- Transfer non-negative integers from queue to stack.
- Print stack of integers after rearranged.
- Define the method “main()”,
- Create object for “Stack”.
- Add integers into the stack.
- Print the stack of integers.
- Call the method “splitStack()” with stack as parameter.
- Define the method “splitstack ()” that accepts stack of integers,

This program demonstrates the method that accepts a stack of integers and rearranges its elements.
Explanation of Solution
Program:
File name: “StackSplit.java”
//Import necessary packages
import java.util.LinkedList;
import java.util.Queue;
import java.util.Stack;
//Create a class
class StackSplit
{
//Define the method
public static void splitStack(Stack<Integer> s)
{
//Construct LinkedList object
Queue<Integer> q1 = new LinkedList<Integer>();
//Calculate the stack size
int old_Length = s.size();
//Execute till stack is empty
while (!s.isEmpty())
{
//Transfer all elements from stack to queue
q1.add(s.pop());
}
//Execute till the stack size
for (int i = 1; i <= old_Length; i++)
{
//Assign the element removed from the queue
int n1 = q1.remove();
//check whether the element is negative
if (n1 < 0)
{
//Insert the element into the stack
s.push(n1);
}
else
{
//Insert the element into the queue
q1.add(n1);
}
}
//Execute till queue is empty
while (!q1.isEmpty())
{
/*Transfer non-negative integers from queue to stack*/
s.push(q1.remove());
}
//Print stack of integers
System.out.println("The elements of a stack of integers after rearranged: "+s);
}
//Define the main() method
public static void main(String[] args)
{
//Create object for Stack
Stack<Integer> s1=new Stack<Integer>();
//Add the integers into the stack
s1.push(3);
s1.push(-5);
s1.push(1);
s1.push(2);
s1.push(-4);
//Print the stack of integers
System.out.println("The elements of a stack of integers: "+s1);
//Call the method
splitStack(s1);
}
}
Output:
The elements of a stack of integers: [3, -5, 1, 2, -4]
The elements of a stack of integers after rearranged: [-4, -5, 2, 1, 3]
Want to see more full solutions like this?
Chapter 14 Solutions
Building Java Programs: A Back To Basics Approach (5th Edition)
- 1. Complete the routing table for R2 as per the table shown below when implementing RIP routing Protocol? (14 marks) 195.2.4.0 130.10.0.0 195.2.4.1 m1 130.10.0.2 mo R2 R3 130.10.0.1 195.2.5.1 195.2.5.0 195.2.5.2 195.2.6.1 195.2.6.0 m2 130.11.0.0 130.11.0.2 205.5.5.0 205.5.5.1 R4 130.11.0.1 205.5.6.1 205.5.6.0arrow_forwardAnalyze the charts and introduce each charts by describing each. Identify the patterns in the given data. And determine how are the data points are related. Refer to the raw data (table):arrow_forward3A) Generate a hash table for the following values: 11, 9, 6, 28, 19, 46, 34, 14. Assume the table size is 9 and the primary hash function is h(k) = k % 9. i) Hash table using quadratic probing ii) Hash table with a secondary hash function of h2(k) = 7- (k%7) 3B) Demonstrate with a suitable example, any three possible ways to remove the keys and yet maintaining the properties of a B-Tree. 3C) Differentiate between Greedy and Dynamic Programming.arrow_forward
- What are the charts (with their title name) that could be use to illustrate the data? Please give picture examples.arrow_forwardA design for a synchronous divide-by-six Gray counter isrequired which meets the following specification.The system has 2 inputs, PAUSE and SKIP:• While PAUSE and SKIP are not asserted (logic 0), thecounter continually loops through the Gray coded binarysequence {0002, 0012, 0112, 0102, 1102, 1112}.• If PAUSE is asserted (logic 1) when the counter is onnumber 0102, it stays here until it becomes unasserted (atwhich point it continues counting as before).• While SKIP is asserted (logic 1), the counter misses outodd numbers, i.e. it loops through the sequence {0002,0112, 1102}.The system has 4 outputs, BIT3, BIT2, BIT1, and WAITING:• BIT3, BIT2, and BIT1 are unconditional outputsrepresenting the current number, where BIT3 is the mostsignificant-bit and BIT1 is the least-significant-bit.• An active-high conditional output WAITING should beasserted (logic 1) whenever the counter is paused at 0102.(a) Draw an ASM chart for a synchronous system to providethe functionality described above.(b)…arrow_forwardS A B D FL I C J E G H T K L Figure 1: Search tree 1. Uninformed search algorithms (6 points) Based on the search tree in Figure 1, provide the trace to find a path from the start node S to a goal node T for the following three uninformed search algorithms. When a node has multiple successors, use the left-to-right convention. a. Depth first search (2 points) b. Breadth first search (2 points) c. Iterative deepening search (2 points)arrow_forward
- We want to get an idea of how many tickets we have and what our issues are. Print the ticket ID number, ticket description, ticket priority, ticket status, and, if the information is available, employee first name assigned to it for our records. Include all tickets regardless of whether they have been assigned to an employee or not. Sort it alphabetically by ticket status, and then numerically by ticket ID, with the lower ticket IDs on top.arrow_forwardFigure 1 shows an ASM chart representing the operation of a controller. Stateassignments for each state are indicated in square brackets for [Q1, Q0].Using the ASM design technique:(a) Produce a State Transition Table from the ASM Chart in Figure 1.(b) Extract minimised Boolean expressions from your state transition tablefor Q1, Q0, DISPATCH and REJECT. Show all your working.(c) Implement your design using AND/OR/NOT logic gates and risingedgetriggered D-type Flip Flops. Your answer should include a circuitschematic.arrow_forwardA controller is required for a home security alarm, providing the followingfunctionality. The alarm does nothing while it is disarmed (‘switched off’). It canbe armed (‘switched on’) by entering a PIN on the keypad. Whenever thealarm is armed, it can be disarmed by entering the PIN on the keypad.If motion is detected while the alarm is armed, the siren should sound AND asingle SMS message sent to the police to notify them. Further motion shouldnot result in more messages being sent. If the siren is sounding, it can only bedisarmed by entering the PIN on the keypad. Once the alarm is disarmed, asingle SMS should be sent to the police to notify them.Two (active-high) input signals are provided to the controller:MOTION: Asserted while motion is detected inside the home.PIN: Asserted for a single clock cycle whenever the PIN has beencorrectly entered on the keypad.The controller must provide two (active-high) outputs:SIREN: The siren sounds while this output is asserted.POLICE: One SMS…arrow_forward
- 4G+ Vo) % 1.1. LTE1 : Q B NIS شوز طبي ۱:۱۷ کا A X حاز هذا على إعجاب Mohamed Bashar. MEDICAL SHOE شوز طبي ممول . اقوى عرض بالعراق بلاش سعر القطعة ١٥ الف سعر القطعتين ٢٥ الف سعر 3 قطع ٣٥ الف القياسات : 40-41-42-43-44- افحص وكدر ثم ادفع خدمة التوصيل 5 الف لكافة محافظات العراق ופרסם BNI SH ופרסם DON JU WORLD DON JU MORISO DON JU إرسال رسالة III Messenger التواصل مع شوز طبي تعليق باسم اواب حمیدarrow_forwardA manipulator is identified by the following table of parameters and variables:a. Obtain the transformation matrices between adjacent coordinate frames and calculate the global transformation matrix.arrow_forwardWhich tool takes the 2 provided input datasets and produces the following output dataset? Input 1: Record First Last Output: 1 Enzo Cordova Record 2 Maggie Freelund Input 2: Record Frist Last MI ? First 1 Enzo Last MI Cordova [Null] 2 Maggie Freelund [Null] 3 Jason Wayans T. 4 Ruby Landry [Null] 1 Jason Wayans T. 5 Devonn Unger [Null] 2 Ruby Landry [Null] 6 Bradley Freelund [Null] 3 Devonn Unger [Null] 4 Bradley Freelund [Null] OA. Append Fields O B. Union OC. Join OD. Find Replace Clear selectionarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
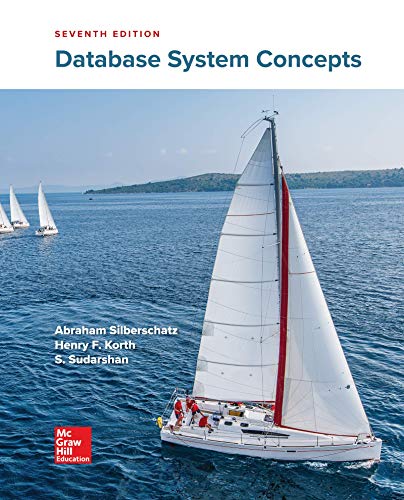
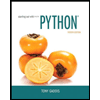
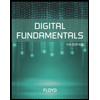
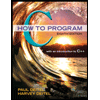
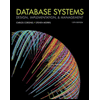
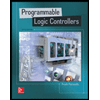