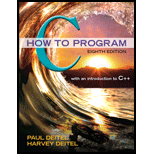
(Variable-Length Argument List: Calculating Products) Write a

Program Plan:
This header file <stdarg.h>is used for object ap of type va_list is used by macros va_startva_arg and va_end to process the variable-length argument list of function product.
product( int x, ) :This function definition product is using a variable length argument list and calculate the product of a series of integers.
Printf (): used to print the data onto output screen.
Variables i, j, l, m, and n are of integer type which are passed as argument to function.
Total variable of integer type is used to store product of integer value.
Program Description: Purpose of the program is to calculates the series of integers that are passed to function product with several calls, by using a variable length list of arguments list and display result.
Explanation of Solution
Program: Following is Cprogram that calculates the series of integers that are passed to function product with several calls, by using a variable length list of arguments list and display result.
#include<stdio.h>//header file #include<stdarg.h>///header-file for variable-length argument lists //Function prototype int product( int x, ... ); //Start of main intmain( void ) { //Initialize the integers inti = 5; int j = 4; int k = 3; int l = 2; int m = 1; //display user the values of integers printf( "%s%d\n%s%d\n%s%d\n%s%d\n%s%d\n","i = ", i, "j = ", j,"k = ", k,"l = ", l, "m = ", m ); //display the result of product using the function call to different series of integers printf( "%s%d\n%s%d\n%s%d\n%s%d\n", "The Product of i and j is: ", product( 2,i,j ), "The Product of i, j and k is: ",product(3,i,j,k ), "The Product of i, j, k and 1 is: ", product(4,i,j,k,l ), "The Product of i, j, k, 1, and m is: ",product(5,i,j,k,l,m) ); //terminate program succesfully return0; }//End of main //function product in which product of integers is passed as arguments int product( int x, ... ) { //Declare and initialize variable total int total = 1; //variable to counter loop intz; //Stores information needed by va_start and va_end. /*initialize variable Length argument List*/ va_listarg; //Stores information needed by va_start. /*invoke the macros to access the arguments*/ va_start( arg, x ); //Process is running for variable length argument list. /*evaluate the total using for loop*/ for( z = 1; z <= x ; z++ ) { total *= va_arg( arg, int); }/*end of for loop*/ //Clean up variable-length argument list. /*Perform the housekeeping termination*/ va_end( arg); /*return the arguments of product*/ returntotal; }/*end of function Product*/
Explanation:
- Use header file notation
for variable-length list of arguments. - Read different series of integers in function call.
- Assign the value of total equals to 1 and use the argument list including va_list of variable list, next initialize va_start ( ) to invoke the macros so as to access the arguments in function definition.
- Loop for to evaluate the value of product.
- Display the product of a series of integers.
Sample Output:
Want to see more full solutions like this?
Chapter 14 Solutions
C How to Program (8th Edition)
Additional Engineering Textbook Solutions
Modern Database Management
Management Information Systems: Managing The Digital Firm (16th Edition)
SURVEY OF OPERATING SYSTEMS
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
Java How to Program, Early Objects (11th Edition) (Deitel: How to Program)
Starting Out with C++ from Control Structures to Objects (9th Edition)
- 1. Complete the routing table for R2 as per the table shown below when implementing RIP routing Protocol? (14 marks) 195.2.4.0 130.10.0.0 195.2.4.1 m1 130.10.0.2 mo R2 R3 130.10.0.1 195.2.5.1 195.2.5.0 195.2.5.2 195.2.6.1 195.2.6.0 m2 130.11.0.0 130.11.0.2 205.5.5.0 205.5.5.1 R4 130.11.0.1 205.5.6.1 205.5.6.0arrow_forwardAnalyze the charts and introduce each charts by describing each. Identify the patterns in the given data. And determine how are the data points are related. Refer to the raw data (table):arrow_forward3A) Generate a hash table for the following values: 11, 9, 6, 28, 19, 46, 34, 14. Assume the table size is 9 and the primary hash function is h(k) = k % 9. i) Hash table using quadratic probing ii) Hash table with a secondary hash function of h2(k) = 7- (k%7) 3B) Demonstrate with a suitable example, any three possible ways to remove the keys and yet maintaining the properties of a B-Tree. 3C) Differentiate between Greedy and Dynamic Programming.arrow_forward
- What are the charts (with their title name) that could be use to illustrate the data? Please give picture examples.arrow_forwardA design for a synchronous divide-by-six Gray counter isrequired which meets the following specification.The system has 2 inputs, PAUSE and SKIP:• While PAUSE and SKIP are not asserted (logic 0), thecounter continually loops through the Gray coded binarysequence {0002, 0012, 0112, 0102, 1102, 1112}.• If PAUSE is asserted (logic 1) when the counter is onnumber 0102, it stays here until it becomes unasserted (atwhich point it continues counting as before).• While SKIP is asserted (logic 1), the counter misses outodd numbers, i.e. it loops through the sequence {0002,0112, 1102}.The system has 4 outputs, BIT3, BIT2, BIT1, and WAITING:• BIT3, BIT2, and BIT1 are unconditional outputsrepresenting the current number, where BIT3 is the mostsignificant-bit and BIT1 is the least-significant-bit.• An active-high conditional output WAITING should beasserted (logic 1) whenever the counter is paused at 0102.(a) Draw an ASM chart for a synchronous system to providethe functionality described above.(b)…arrow_forwardS A B D FL I C J E G H T K L Figure 1: Search tree 1. Uninformed search algorithms (6 points) Based on the search tree in Figure 1, provide the trace to find a path from the start node S to a goal node T for the following three uninformed search algorithms. When a node has multiple successors, use the left-to-right convention. a. Depth first search (2 points) b. Breadth first search (2 points) c. Iterative deepening search (2 points)arrow_forward
- We want to get an idea of how many tickets we have and what our issues are. Print the ticket ID number, ticket description, ticket priority, ticket status, and, if the information is available, employee first name assigned to it for our records. Include all tickets regardless of whether they have been assigned to an employee or not. Sort it alphabetically by ticket status, and then numerically by ticket ID, with the lower ticket IDs on top.arrow_forwardFigure 1 shows an ASM chart representing the operation of a controller. Stateassignments for each state are indicated in square brackets for [Q1, Q0].Using the ASM design technique:(a) Produce a State Transition Table from the ASM Chart in Figure 1.(b) Extract minimised Boolean expressions from your state transition tablefor Q1, Q0, DISPATCH and REJECT. Show all your working.(c) Implement your design using AND/OR/NOT logic gates and risingedgetriggered D-type Flip Flops. Your answer should include a circuitschematic.arrow_forwardA controller is required for a home security alarm, providing the followingfunctionality. The alarm does nothing while it is disarmed (‘switched off’). It canbe armed (‘switched on’) by entering a PIN on the keypad. Whenever thealarm is armed, it can be disarmed by entering the PIN on the keypad.If motion is detected while the alarm is armed, the siren should sound AND asingle SMS message sent to the police to notify them. Further motion shouldnot result in more messages being sent. If the siren is sounding, it can only bedisarmed by entering the PIN on the keypad. Once the alarm is disarmed, asingle SMS should be sent to the police to notify them.Two (active-high) input signals are provided to the controller:MOTION: Asserted while motion is detected inside the home.PIN: Asserted for a single clock cycle whenever the PIN has beencorrectly entered on the keypad.The controller must provide two (active-high) outputs:SIREN: The siren sounds while this output is asserted.POLICE: One SMS…arrow_forward
- 4G+ Vo) % 1.1. LTE1 : Q B NIS شوز طبي ۱:۱۷ کا A X حاز هذا على إعجاب Mohamed Bashar. MEDICAL SHOE شوز طبي ممول . اقوى عرض بالعراق بلاش سعر القطعة ١٥ الف سعر القطعتين ٢٥ الف سعر 3 قطع ٣٥ الف القياسات : 40-41-42-43-44- افحص وكدر ثم ادفع خدمة التوصيل 5 الف لكافة محافظات العراق ופרסם BNI SH ופרסם DON JU WORLD DON JU MORISO DON JU إرسال رسالة III Messenger التواصل مع شوز طبي تعليق باسم اواب حمیدarrow_forwardA manipulator is identified by the following table of parameters and variables:a. Obtain the transformation matrices between adjacent coordinate frames and calculate the global transformation matrix.arrow_forwardWhich tool takes the 2 provided input datasets and produces the following output dataset? Input 1: Record First Last Output: 1 Enzo Cordova Record 2 Maggie Freelund Input 2: Record Frist Last MI ? First 1 Enzo Last MI Cordova [Null] 2 Maggie Freelund [Null] 3 Jason Wayans T. 4 Ruby Landry [Null] 1 Jason Wayans T. 5 Devonn Unger [Null] 2 Ruby Landry [Null] 6 Bradley Freelund [Null] 3 Devonn Unger [Null] 4 Bradley Freelund [Null] OA. Append Fields O B. Union OC. Join OD. Find Replace Clear selectionarrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
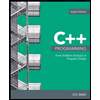
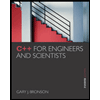
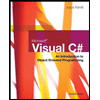