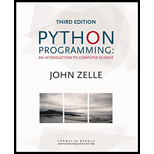
Concept explainers
Maximum of list
Program plan:
- Import the package.
- Define the “Max()” function,
- Make simultaneous assignment to initialize the variables.
- Assign the values return from “len()”.
- Execute while loop when both “lst_1” and “lst_2” have more items,
- Check whether top of “lst_1” is larger,
- If it is “True”, copy that top value into current location in “lst_3”.
- Increment “s1” by “1”.
- Otherwise,
- Copy the top of “lst_2” into the current location in “lst_3”.
- Increment “s2” by “1”.
- Increment “s3” by “1” when element is added to “lst_3”.
- Check whether top of “lst_1” is larger,
- Execute the “while” loop to copy remaining elements from “lst_1”,
- Copy the element.
- Increment “s1” by “1”.
- Increment “s3” by “1”.
- Execute the "while" loop to copy remaining elements from “lst_2”,
- Copy the element.
- Increment “s2” by “1”.
- Increment “s3” by “1”.
- Define the function “merge_Sort()”,
- Assign the initial values for the variable.
- Check whether the number element is greater than 1,
- Split the list into two sub lists.
- Make simultaneous assignment to assign two sub lists using slicing.
- Make recursive calls to sort each sub list.
- Merge the sorted sub list into original list.
- Create empty list.
- Create for loop to iterate “100” times.
- Append the random value to the end of the list.
- Call the “merge_Sort()” function.
- Print the list.

This Python program is to demonstrate a recursive function “Max()” to find the largest number in a list where the largest number is the larger of the first item and the maximum of all the other items.
Explanation of Solution
Program:
File name: “conference.py”
#Import the package
from random import randrange
#Define the function
def Max(lst_1, lst_2, lst_3):
#Make simultaneous assignment
s1, s2, s3 = 0, 0 , 0
#Assign the values return from len()
n1, n2 = len(lst_1), len(lst_2)
'''Execute while loop when both lst_1 and lst_2 have more items'''
while s1 < n1 and s2 < n2:
#Check whether top of lst_1 is larger
if lst_1[s1] > lst_2[s2]:
'''Copy that top value into current location in lst_3'''
lst_3[s3] = lst_1[s1]
#Increment by "1"
s1 = s1 + 1
#Otherwise
else:
'''Copy the top of lst_2 into the current location in lst_3'''
lst_3[s3] = lst_2[s2]
#Increment by "1"
s2 = s2 + 1
#Increment by "1" when element added into lst_3
s3 = s3 + 1
'''Execute the "while" loop to copy remaining elements from lst_1'''
while s1 < n1:
#Copy the element
lst_3[s3] = lst_1[s1]
#Increment by "1"
s1 = s1 + 1
#Increment by "1"
s3 = s3 + 1
'''Execute the "while" loop to copy remaining elements from lst1_2'''
while s2 < n2:
#Copy the element
lst_3[s3] = lst_2[s2]
#Increment by "1"
s2 = s2 + 1
#Increment by "1"
s3 = s3 + 1
#Define the function
def merge_Sort(num):
#Assign the value
n = len(num)
'''Check whether the number element is greater than 1'''
if n > 1:
#Split the list into two sub lists
v = n // 2
'''Make simultaneous assignment to assign two sub lists using slicing'''
nums_1, nums_2 = num[:v], num[v:]
#Make recursive calls to sort each sub list
merge_Sort(nums_1)
merge_Sort(nums_2)
#Merge the sorted sub list into original list
Max(nums_1, nums_2, num)
#Create empty list
lst = []
#Create for loop
for n in range(100):
#Append the random value to the end of the list
lst.append(randrange(1, 1000))
#Call the function
merge_Sort(lst)
#Print the list
print(lst)
Output:
[996, 992, 975, 974, 956, 952, 947, 937, 921, 915, 902, 899, 891, 889, 886, 863, 863, 862, 856, 841, 837, 819, 808, 791, 760, 755, 754, 739, 736, 732, 685, 663, 638, 628, 623, 622, 612, 594, 569, 562, 552, 532, 531, 528, 527, 523, 492, 475, 468, 461, 452, 451, 444, 438, 431, 413, 405, 401, 375, 370, 358, 354, 350, 350, 347, 339, 325, 290, 286, 246, 227, 220, 215, 188, 185, 182, 168, 161, 149, 142, 141, 141, 139, 135, 115, 114, 112, 101, 96, 94, 94, 85, 84, 84, 80, 78, 70, 18, 13, 11]
>>>
Want to see more full solutions like this?
Chapter 13 Solutions
Python Programming: An Introduction to Computer Science, 3rd Ed.
- .NET Interactive Solving Sudoku using Grover's Algorithm We will now solve a simple problem using Grover's algorithm, for which we do not necessarily know the solution beforehand. Our problem is a 2x2 binary sudoku, which in our case has two simple rules: •No column may contain the same value twice •No row may contain the same value twice If we assign each square in our sudoku to a variable like so: 1 V V₁ V3 V2 we want our circuit to output a solution to this sudoku. Note that, while this approach of using Grover's algorithm to solve this problem is not practical (you can probably find the solution in your head!), the purpose of this example is to demonstrate the conversion of classical decision problems into oracles for Grover's algorithm. Turning the Problem into a Circuit We want to create an oracle that will help us solve this problem, and we will start by creating a circuit that identifies a correct solution, we simply need to create a classical function on a quantum circuit that…arrow_forwardusing r languagearrow_forward8. Cash RegisterThis exercise assumes you have created the RetailItem class for Programming Exercise 5. Create a CashRegister class that can be used with the RetailItem class. The CashRegister class should be able to internally keep a list of RetailItem objects. The class should have the following methods: A method named purchase_item that accepts a RetailItem object as an argument. Each time the purchase_item method is called, the RetailItem object that is passed as an argument should be added to the list. A method named get_total that returns the total price of all the RetailItem objects stored in the CashRegister object’s internal list. A method named show_items that displays data about the RetailItem objects stored in the CashRegister object’s internal list. A method named clear that should clear the CashRegister object’s internal list. Demonstrate the CashRegister class in a program that allows the user to select several items for purchase. When the user is ready to check out, the…arrow_forward
- 5. RetailItem ClassWrite a class named RetailItem that holds data about an item in a retail store. The class should store the following data in attributes: item description, units in inventory, and price. Once you have written the class, write a program that creates three RetailItem objects and stores the following data in them: Description Units in Inventory PriceItem #1 Jacket 12 59.95Item #2 Designer Jeans 40 34.95Item #3 Shirt 20 24.95arrow_forwardFind the Error: class Information: def __init__(self, name, address, age, phone_number): self.__name = name self.__address = address self.__age = age self.__phone_number = phone_number def main(): my_info = Information('John Doe','111 My Street', \ '555-555-1281')arrow_forwardFind the Error: class Pet def __init__(self, name, animal_type, age) self.__name = name; self.__animal_type = animal_type self.__age = age def set_name(self, name) self.__name = name def set_animal_type(self, animal_type) self.__animal_type = animal_typearrow_forward
- Task 2: Comparable Interface and Record (10 Points) 1. You are tasked with creating a Java record of Dog (UML is shown below). The dog record should include the dog's name, breed, age, and weight. You are required to implement the Comparable interface for the Dog record so that you can sort the records based on the dogs' ages. Create a Java record named Dog.java. name: String breed: String age: int weight: double + toString(): String > Dog + compareTo(otherDog: Dog): int > Comparable 2. In the Dog record, establish a main method and proceed to generate an array named dogList containing three Dog objects, each with the following attributes: Dog1: name: "Buddy", breed: "Labrador Retriever", age: 5, weight: 25.5 Dog2: name: "Max", breed: "Golden Retriever", age: 3, weight: 30 Dog3: name: "Charlie", breed: "German Shepherd", age: 2, weight: 22 3. Print the dogs in dogList before sorting the dogList by age. (Please check the example output for the format). • 4. Sort the dogList using…arrow_forwardThe OSI (Open Systems Interconnection) model is a conceptual framework that standardises the functions of a telecommunication or computing system into seven distinct layers, facilitating communication and interoperability between diverse network protocols and technologies. Discuss the OSI model's physical layer specifications when designing the physical network infrastructure for a new office.arrow_forwardIn a network, information about how to reach other IP networks or hosts is stored in a device's routing table. Each entry in the routing table provides a specific path to a destination, enabling the router to forward data efficiently across the network. The routing table contains key parameters determining the available routes and how traffic is directed toward its destination. Briefly explain the main parameters that define a routing entry.arrow_forward
- You are troubleshooting a network issue where an employee's computer cannot connect to the corporate network. The computer is connected to the network via an Ethernet cable that runs to a switch. Suspecting a possible layer 1 or layer 2 problem, you decide to check the LED status indicators on both the computer's NIC and the corresponding port on the switch. Describe five LED link states and discuss what each indicates to you as a network technician.arrow_forwardYou are a network expert tasked with upgrading the network infrastructure for a growing company expanding its operations across multiple floors. The new network setup needs to support increased traffic and future scalability and provide flexibility for network management. The company is looking to implement Ethernet switches to connect various devices, including workstations, printers, and IP cameras. As part of your task, you must select the appropriate types of Ethernet switches to meet the company's needs. Evaluate the general Ethernet switch categories you would consider for this project, including their features and how they differ.arrow_forwardYou are managing a Small Office Home Office (SOHO) network connected to the Internet via a fibre link provided by your Internet Service Provider (ISP). Recently, you have noticed a significant decrease in Internet speed, and after contacting your ISP, they confirmed that no issues exist on their end. Considering that the problem may lie within your local setup, identify three potential causes of the slow Internet connection, focusing on physical factors affecting the fibre equipment.arrow_forward
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:Cengage
- Systems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage LearningNew Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage LearningCOMPREHENSIVE MICROSOFT OFFICE 365 EXCEComputer ScienceISBN:9780357392676Author:FREUND, StevenPublisher:CENGAGE L
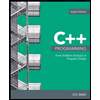
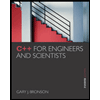
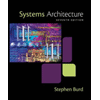
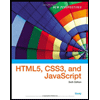