String Splitter
Write a function
Every good boy does fine,
results in the output
Every
good
boy
does
fine.

Want to see the full answer?
Check out a sample textbook solution
Chapter 12 Solutions
STARTING OUT WITH C++ MPL
Additional Engineering Textbook Solutions
Starting Out with Python (4th Edition)
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
Starting Out with Java: From Control Structures through Data Structures (3rd Edition)
Starting Out with C++ from Control Structures to Objects (9th Edition)
Starting Out with Programming Logic and Design (5th Edition) (What's New in Computer Science)
Problem Solving with C++ (10th Edition)
- Write in c++ pleasearrow_forwardWhat are values of vector "vec", if QUANTILE function returns following values? > quantile (vec) 0% 25% 50% 75% 100% 0.0 12.5 25.0 37.5 50.0arrow_forwardt. Given a string as a parameter to the function, return a square grid filled with all the characters of the string from left to right. Fill empty spaces in the grid with '_'. Sample Input: stringtogrid Output: {{s, t, r, i}, {n, g, t, o}, {g, r, i, d}, {_, _, _, _}} Complete the function: vector<vector<int> formGrid(string s){}arrow_forward
- Write c++arrow_forwardC++ languagearrow_forwardC++ Vectors Help: write a program using parallel vectors and a function which fills each of them with 500 random numbers between 1 and 100. The program should then pass both vectors to a function which will return an integer indicating a count of how many times both vectors had even numbers in the same location. So if vector01[0] contained 4 and vector02[0] contained 12, you would add one to count.If vector01[1] contained 3 and vector02[1] contained 4, you would not add one to count. main would display something like : The Vectors contain 128 cells where both values are even. Above was the question: I already have the code written: #include<iostream> #include<vector> #include<cstdlib> #include<ctime> using namespace std; int main() { int even = 0; srand(time(0)); vector <int> vector01; vector <int> vector02; for (int i = 0; i < 500; i++) { vector01.push_back(rand() % 100 + 1); vector02.push_back(rand() % 100 + 1); if (vector01[i] % 2 == 0…arrow_forward
- Please complete the following functions: 1. int loadDictionary(istream& dictfile, vector<string>& dict) 2. int permute(string word, vector<string>& dict, vector<string>& results) 3. void display(vector<string>& results)arrow_forwardCreat a class reversit and Write a function called reversit () that reverses a C-string (an array of char). Use a forloop that swaps the first and last characters, then the second and next-to-last characters, and so on. The string should be passed to reversit () as an argument. Write a program to exercise reversit (). The program should get a string from the user, call reversit (), and print out the result.by using c++arrow_forwardIn C++: Define a function that reads input 5 integers from the user, stores them in a vector, and then returns the vector from the function.arrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
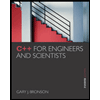