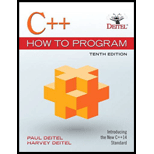
C++ How to Program (Early Objects Version)
10th Edition
ISBN: 9780134448824
Author: Paul Deitel; Harvey M. Deitel
Publisher: Pearson Education (US)
expand_more
expand_more
format_list_bulleted
Concept explainers
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Which tool takes the 2 provided input datasets and produces the following output dataset?
Input 1:
Record
First
Last
Output:
1 Enzo
Cordova
Record
2 Maggie
Freelund
Input 2:
Record
Frist
Last
MI
?
First
1 Enzo
Last
MI
Cordova
[Null]
2 Maggie
Freelund
[Null]
3 Jason
Wayans
T.
4 Ruby
Landry
[Null]
1 Jason
Wayans
T.
5 Devonn
Unger
[Null]
2 Ruby
Landry
[Null]
6 Bradley
Freelund
[Null]
3 Devonn
Unger
[Null]
4 Bradley
Freelund
[Null]
OA. Append Fields
O B. Union
OC. Join
OD. Find Replace
Clear selection
What are the similarities and differences between massively parallel processing systems and grid computing. with references
Modular Program Structure. Analysis of Structured Programming Examples. Ways to Reduce Coupling.
Based on the given problem, create an algorithm and a block diagram, and write the program code:
Function: y=xsinx
Interval: [0,π]
Requirements:
Create a graph of the function.
Show the coordinates (x and y).
Choose your own scale and show it in the block diagram.
Create a block diagram based on the algorithm.
Write the program code in Python.
Requirements:
Each step in the block diagram must be clearly shown.
The graph of the function must be drawn and saved (in PNG format).
Write the code in a modular way (functions and the main part should be separate).
Please explain and describe the results in detail.
Chapter 12 Solutions
C++ How to Program (Early Objects Version)
Ch. 12 - (Programming in the General) How is it that...Ch. 12 - (Polymorphism vs. switch logic) Discuss the...Ch. 12 - (Inheriting Interface vs. Implementation)...Ch. 12 - Prob. 12.6ECh. 12 - Prob. 12.7ECh. 12 - Prob. 12.8ECh. 12 - Prob. 12.9ECh. 12 - (Polymorphic Application) You've been asked to...Ch. 12 - (Payroll-System Modification) Modify the payroll...Ch. 12 - Prob. 12.12E
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Based on the given problem, create an algorithm and a block diagram, and write the program code: Function: y=xsinx Interval: [0,π] Requirements: Create a graph of the function. Show the coordinates (x and y). Choose your own scale and show it in the block diagram. Create a block diagram based on the algorithm. Write the program code in Python. Requirements: Each step in the block diagram must be clearly shown. The graph of the function must be drawn and saved (in PNG format). Write the code in a modular way (functions and the main part should be separate). Please explain and describe the results in detail.arrow_forwardBased on the given problem, create an algorithm and a block diagram, and write the program code: Function: y=xsinx Interval: [0,π] Requirements: Create a graph of the function. Show the coordinates (x and y). Choose your own scale and show it in the block diagram. Create a block diagram based on the algorithm. Write the program code in Python. Requirements: Each step in the block diagram must be clearly shown. The graph of the function must be drawn and saved (in PNG format). Write the code in a modular way (functions and the main part should be separate). Please explain and describe the results in detail.arrow_forwardQuestion: Based on the given problem, create an algorithm and a block diagram, and write the program code: Function: y=xsinx Interval: [0,π] Requirements: Create a graph of the function. Show the coordinates (x and y). Choose your own scale and show it in the block diagram. Create a block diagram based on the algorithm. Write the program code in Python. Requirements: Each step in the block diagram must be clearly shown. The graph of the function must be drawn and saved (in PNG format). Write the code in a modular way (functions and the main part should be separate). Please explain and describe the results in detail.arrow_forward
- 23:12 Chegg content://org.teleg + 5G 5G 80% New question A feed of 60 mol% methanol in water at 1 atm is to be separated by dislation into a liquid distilate containing 98 mol% methanol and a bottom containing 96 mol% water. Enthalpy and equilibrium data for the mixture at 1 atm are given in Table Q2 below. Ask an expert (a) Devise a procedure, using the enthalpy-concentration diagram, to determine the minimum number of equilibrium trays for the condition of total reflux and the required separation. Show individual equilibrium trays using the the lines. Comment on why the value is Independent of the food condition. Recent My stuff Mol% MeOH, Saturated vapour Table Q2 Methanol-water vapour liquid equilibrium and enthalpy data for 1 atm Enthalpy above C˚C Equilibrium dala Mol% MeOH in Saturated liquid TC kJ mol T. "Chk kot) Liquid T, "C 0.0 100.0 48.195 100.0 7.536 0.0 0.0 100.0 5.0 90.9 47,730 928 7,141 2.0 13.4 96.4 Perks 10.0 97.7 47,311 87.7 8,862 4.0 23.0 93.5 16.0 96.2 46,892 84.4…arrow_forwardYou are working with a database table that contains customer data. The table includes columns about customer location such as city, state, and country. You want to retrieve the first 3 letters of each country name. You decide to use the SUBSTR function to retrieve the first 3 letters of each country name, and use the AS command to store the result in a new column called new_country. You write the SQL query below. Add a statement to your SQL query that will retrieve the first 3 letters of each country name and store the result in a new column as new_country.arrow_forwardWe are considering the RSA encryption scheme. The involved numbers are small, so the communication is insecure. Alice's public key (n,public_key) is (247,7). A code breaker manages to factories 247 = 13 x 19 Determine Alice's secret key. To solve the problem, you need not use the extended Euclid algorithm, but you may assume that her private key is one of the following numbers 31,35,55,59,77,89.arrow_forward
- Consider the following Turing Machine (TM). Does the TM halt if it begins on the empty tape? If it halts, after how many steps? Does the TM halt if it begins on a tape that contains a single letter A followed by blanks? Justify your answer.arrow_forwardPllleasassseee ssiiirrrr soolveee thissssss questionnnnnnnarrow_forwardPllleasassseee ssiiirrrr soolveee thissssss questionnnnnnnarrow_forward
- Pllleasassseee ssiiirrrr soolveee thissssss questionnnnnnnarrow_forwardPllleasassseee ssiiirrrr soolveee thissssss questionnnnnnnarrow_forward4. def modify_data(x, my_list): X = X + 1 my_list.append(x) print(f"Inside the function: x = {x}, my_list = {my_list}") num = 5 numbers = [1, 2, 3] modify_data(num, numbers) print(f"Outside the function: num = {num}, my_list = {numbers}") Classe Classe that lin Thus, A pro is ref inter Ever dict The The output: Inside the function:? Outside the function:?arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage Learning
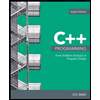
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
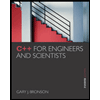
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
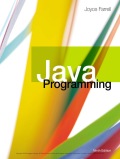
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
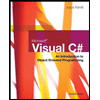
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
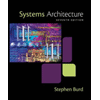
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning
What is Abstract Data Types(ADT) in Data Structures ? | with Example; Author: Simple Snippets;https://www.youtube.com/watch?v=n0e27Cpc88E;License: Standard YouTube License, CC-BY