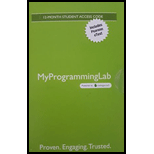
(Supermarket Simulation) Write a program that simulates a check-out line at a supermarket. The line is a queue. Customers arrive in random integer intervals of 1 to 4 minutes. Also, each customer is serviced in random integer intervals of 1 to 4 minutes. Obviously, the rates need to be balanced. If the average arrival rate is larger than the average service rate, the queue will grow infinitely. Even with balanced rates, randomness can still cause long lines. Run the supermarket simulation for a 12-hour day (720 minutes) using the following
A. Choose a random integer between 1 and 4 to determine the minute at which the first customer arrives.
B. At the first customer’s arrival time:
Determine customer’s service time (random integer from 1 to 4);
Begin servicing the customer;
Schedule arrival time of next customer (random integer 1 to 4 added to the current time).
C. For each minute of the day:
If the next customer arrives,
Say so;
Enqueue the customer;
Schedule the arrival time of the next customer.
If service was completed for the last customer,
Say so;
Dequeue next customer to be serviced;
Determine customer’s service completion time (random integer from 1 to 4 added to the current time).
Now run your simulation for 720 minutes and answer each of the following:
- What’s the maximum number of customers in the queue at any time?
- What’s the longest wait any one customer experienced?
- What happens if the arrival interval is changed from 1 to 4 minutes to 1 to 3 minutes?

Want to see the full answer?
Check out a sample textbook solution
Chapter 12 Solutions
MYPROGRAMMINGLAB WITH PEARSON ETEXT
Additional Engineering Textbook Solutions
Absolute Java (6th Edition)
Introduction To Programming Using Visual Basic (11th Edition)
Java: An Introduction to Problem Solving and Programming (8th Edition)
Starting Out With Visual Basic (7th Edition)
Computer Science: An Overview (12th Edition)
Starting out with Visual C# (4th Edition)
- [Python Language] Using loops of any kind, lists, or is not allowed. Angela loves reading books. She recently started reading an AI generated series called “Harry Trotter”. Angela is collecting books from the series at her nearest bookstore. Since the series is AI generated, the publishers have produced an infinite collection of the books where each book is identified by a unique integer. The bookstore has exactly one copy of each book. Angela wants to buy the books in the range [l,r], where l ≤ r. As an example, the range [−3,3] means that Angela wants to buy the books − 3, − 2, − 1, 0, 1, 2, and 3. Dan also loves the series (or maybe annoying Angela – who knows, really), and he manages to sneak into the bookstore very early to buy all of the books in the range [d,u], where d ≤ u. When Angela later visits, sadly she will not find those books there anymore. For example, if Angela tries to buy books [−2,3] and Dan has bought books [0,2], Angela would only receive books − 2, − 1,…arrow_forwardNeed help in python. Problem: 2D random walk. A two dimensional random walk simulates the behavior of a particle moving in a grid of points. At each step, the random walker moves north, south, east, or west with probability 1/4, independently of previous moves. Compose a program that takes a command-line argument n and estimates how long it will take a random walker to hit the boundary of a 2n+1-by-2n+1 square centered at the starting point. //Given codeImport stdioImport randomImport sysn = int(sys.argv[1])//write code herestdio.write('The walker took ')stdio.write(c)stdio.writeln(' steps')arrow_forwardIn C Programming Language solve the following programarrow_forward
- Provide full C++ Code This assignment is a review of loops. Do not use anything more advanced than a loop, such as functions or arrays or classes. In the card game named 'blackjack' players get two cards to start with, and then they are asked whether or not they want more cards. Players can continue to take as many cards as they like. Their goal is to get as close as possible to a total of 21 without going over. Face cards have a value of 10. Write a command line game that plays a simple version of blackjack. The program should generate a random number between 1 and 10 each time the player gets a card. Each of the values (1 through 10) must be equally likely. (In other words, this won't be like real black jack where getting a 10 is more likely than getting some other value, because in real black jack all face cards count as 10.) It should keep a running total of the players cards, and ask the player whether or not it should deal another card. Sample output for the game is written…arrow_forwardPython answer only. Correct answer will upvoted else downvoted. It is the ideal opportunity for your very first race in the game against Ronnie. To make the race intriguing, you have wagered a dollars and Ronnie has wagered b dollars. Yet, the fans appear to be frustrated. The fervor of the fans is given by gcd(a,b), where gcd(x,y) means the best normal divisor (GCD) of integers x and y. To make the race seriously invigorating, you can perform two kinds of activities: Increment both an and b by 1. Diminishing both an and b by 1. This activity must be performed if both an and b are more noteworthy than 0. In one action, you can play out any of these activities. You can perform self-assertive (potentially zero) number of moves. Decide the greatest energy the fans can get and the base number of moves needed to accomplish it. Note that gcd(x,0)=x for any x≥0. Input The principal line of input contains a solitary integer t (1≤t≤5⋅103) — the number of experiments.…arrow_forwardWrite a MATLAB code to create a matrix of random numbers with 4 rows and three columns. Then use nested loops and IF to put zero instead of the numbers that are greater than 0.5.arrow_forward
- Computer Science Engineering:arrow_forwardWATERSORT PROGRAM - JAVA •The aim of Part 2 is to set up the puzzle for the player to solve in Part 3. IMPORTANT NOTE: We are not going to start with 2 empty bottles, but rather with 8 empty slots spread over the 5 bottles as explained in the intro video. Start with 5 empty bottles. Use a random number generator to fill bottles one slot at a time with the colours while keeping 8 slots empty – there is a total of 5*4 = 20 slots – so if we will 12 slots, 8 are free. •Advantage: Easy to create a puzzle with a good mix •Disadvantage: With more colours and bottles and only 2 open bottles for more advanced versions of the game, the result might not be solvable. Start with three sorted bottles. In the strategy the idea is to load three bottles with uniform colour and then move ink around for a number of moves until the bottles are mixed up. •Advantage: Result is always solvable since the bottles are created in a reversed-game strategy. •Disadvantage: It is hard to develop an algorithm which…arrow_forwardThis needs to be done in Java! (Sales Commission Calculator) A large company pays its salespeople on a commission basis. The salespeople receive $200 per week plus 9% of their gross sales for that week. For example, a salesperson who sells $5,000 worth of merchandise in a week receives $200 plus 9% of $5,000, or a total of $650. You’ve been supplied with a list of the items sold by each salesperson. The values of these items are shown below. Develop a Java application that inputs one salesperson’s items sold for last week and calculates and displays that salesperson’s earnings. There’s no limit to the number of items that can be sold. Item Value 1 239.99 2 129.75 3 99.95 4 350.89 Example Output: Enter number sold of product #1: 10Enter number sold of product #2: 20Enter number sold of product #3: 30Enter number sold of product #4: 40Earnings this week: $2182.61 NOTES: Do not create a separate class for this program. Create the…arrow_forward
- Solve and complete.arrow_forwardCourse:Artificial Intelligence Topic:Sample Neural Network to calculate total error using Forward pass backpropagation. Problem:Implement forward pass backpropagation for 100(for two input 200)random numbers.(Set range for random numbers 0.01-1.00) using C++/Python with short explanation with key points.arrow_forwardParallel Lines python 3 Write a program to draw a set of linear lines with equation y = mx + b where m is the slope of the line and b is the y-intercept. Prompt user to enter the slope and y-intercept. Draw the line from x=-10 to x=10. Use numpy arange method to generate x values from -10 to 10 with step size of 0.1. For example., x = np.arange(-10, 10, 0.1) Use the plt.axis() function to set the range of x and y values from -10 to 10. Add xlabel with x-axis, ylabel with y-axis, and title Parallel Lines. After you have drawn the line, draw two lines that are parallel to the first line. Hint: Two lines are parallel if they have the same slope but different y-intercept. Draw two lines where the y-intercept value is one unit below the first line, and one unit above the first line. or example, if the first line y-intercept is 4, then draw lines with y-intercept of 3 and y-intercept of 5.arrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
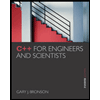