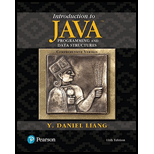
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
11th Edition
ISBN: 9780134670942
Author: Y. Daniel Liang
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Concept explainers
Textbook Question
Chapter 11.14, Problem 11.14.1CP
What modifier should you use on a class so a class in the same package can access it, but a class in a different package cannot access it?
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
Describe how you can use an interface as an alternative to a class to specify the type for a parameter.
package assignment;
public class Circle2D2 {
//data fields specifying the center of the circle
private double x, y;
//data field radius
private double radius;
//default circle with (0, 0) for (x, y) and 1 for radius
Circle2D2() {
this(0, 0, 1);
}
//circle with the specified x, y, and radius
Circle2D2(double x, double y, double radius) {
this.x = x;
this.y = y;
this.radius = radius;
}
//return x
public double getX() {
return x;
}
//return y
public double getY() {
return y;
}
//return radius
public double getRadius() {
return radius;
}
//return the area of the circle
public double getArea() {
return Math.PI * Math.pow(radius, 2);
}
//return the perimeter of the circle
public double getPerimeter() {
return 2 * Math.PI * radius;
}
//return true if the specified point (x, y) is inside this circle
public boolean contains(double x, double y) {
return Math.sqrt(Math.pow(x - this.x, 2) +
Math.pow(y - this.y, 2))
< radius;
}
//return true if…
Why is it vital to implement a destructor in a class?
Chapter 11 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Ch. 11.2 - True or false? A subclass is subset of a...Ch. 11.2 - What keyword do you use to define a subclass?Ch. 11.2 - What is single inheritance? What is multiple...Ch. 11.3 - What is the output of running the class C in (a)?...Ch. 11.3 - How does a subclass invoke its superclasss...Ch. 11.3 - True or false? When invoking a constructor from a...Ch. 11.4 - True or false? You can override a private method...Ch. 11.4 - True or false? You can override a static method...Ch. 11.4 - How do you explicitly invoke a superclasss...Ch. 11.4 - How do you invoke an overridden superclass method...
Ch. 11.5 - Identify the problems in the following code:...Ch. 11.5 - Prob. 11.5.2CPCh. 11.5 - If a method in a subclass has the same signature...Ch. 11.5 - If a method in a subclass has the same signature...Ch. 11.5 - If a method in a subclass has the same name as a...Ch. 11.5 - Prob. 11.5.6CPCh. 11.7 - Prob. 11.7.1CPCh. 11.8 - Prob. 11.8.1CPCh. 11.8 - Prob. 11.8.2CPCh. 11.8 - Can you assign new int[50], new Integer [50], new...Ch. 11.8 - Prob. 11.8.4CPCh. 11.8 - Show the output of the following code:Ch. 11.8 - Show the output of following program: 1public...Ch. 11.8 - Show the output of following program: public class...Ch. 11.9 - Indicate true or false for the following...Ch. 11.9 - For the GeometricObject and Circle classes in...Ch. 11.9 - Suppose Fruit, Apple, Orange, GoldenDelicious, and...Ch. 11.9 - What is wrong in the following code? 1public class...Ch. 11.10 - Prob. 11.10.1CPCh. 11.11 - Prob. 11.11.1CPCh. 11.11 - Prob. 11.11.2CPCh. 11.11 - Prob. 11.11.3CPCh. 11.11 - Prob. 11.11.4CPCh. 11.11 - Prob. 11.11.5CPCh. 11.12 - Correct errors in the following statements: int[]...Ch. 11.12 - Correct errors in the following statements: int[]...Ch. 11.13 - Prob. 11.13.1CPCh. 11.14 - What modifier should you use on a class so a class...Ch. 11.14 - Prob. 11.14.2CPCh. 11.14 - In the following code, the classes A and B are in...Ch. 11.14 - In the following code, the classes A and B are in...Ch. 11.15 - Prob. 11.15.1CPCh. 11.15 - Indicate true or false for the following...Ch. 11 - Sections 11.211.4 11.1(The Triangle class) Design...Ch. 11 - (Subclasses of Account) In Programming Exercise...Ch. 11 - (Maximum element in ArrayList) Write the following...Ch. 11 - Prob. 11.5PECh. 11 - (Use ArrayList) Write a program that creates an...Ch. 11 - (Shuffle ArrayList) Write the following method...Ch. 11 - (New Account class) An Account class was specified...Ch. 11 - (Largest rows and columns) Write a program that...Ch. 11 - Prob. 11.10PECh. 11 - (Sort ArrayList) Write the following method that...Ch. 11 - (Sum ArrayList) Write the following method that...Ch. 11 - (Remove duplicates) Write a method that removes...Ch. 11 - (Combine two lists) Write a method that returns...Ch. 11 - (Area of a convex polygon) A polygon is convex if...Ch. 11 - Prob. 11.16PECh. 11 - (Algebra: perfect square) Write a program that...Ch. 11 - (ArrayList of Character) Write a method that...Ch. 11 - (Bin packing using first fit) The bin packing...
Additional Engineering Textbook Solutions
Find more solutions based on key concepts
This optional Google account security feature sends you a message with a code that you must enter, in addition ...
SURVEY OF OPERATING SYSTEMS
What is a compiler?
Starting Out with Java: From Control Structures through Data Structures (4th Edition) (What's New in Computer Science)
Assume a telephone signal travels through a cable at two-thirds the speed of light. How long does it take the s...
Electric Circuits. (11th Edition)
(Displaying a Square of Asterisks) Write a method squareOfAsterisks that displays a solid square (the same numb...
Java How to Program, Early Objects (11th Edition) (Deitel: How to Program)
True or False: The superclass constructor always executes before the subclass constructor.
Starting Out with Java: From Control Structures through Objects (7th Edition) (What's New in Computer Science)
F46. Determine the moment of the force about point O.
INTERNATIONAL EDITION---Engineering Mechanics: Statics, 14th edition (SI unit)
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Java A class always has a constructor that does not take any parameters even if there are other constructors in the class that take parameters. Choose one of the options:TrueFalsearrow_forwardT/F: Instance variables are shared by all the instances of the class. T/F: The scope of instance and static variables is the entire class. They can be declared anywhere inside a class. T/F: To declare static variables, constants, and methods, use the static modifier.arrow_forwardQ:-Write a statement in ClassA to check out the accessibility of your variable. package package_02; public class ClassB { public int x = 1; int y = 2; private int z =3; } package package_01; import package_02.ClassB; public class ClassA { public static void main(String[] args) { ClassB cb = new ClassB(); // System.out.print(cb.x); /* 1 */ // System.out.print(cb.y); /* 2 */ // System.out.print(cb.z); /* 3 */ } }arrow_forward
- java Assignment Description: Write a class named Car that has the following fields: The yearModel field is an int that holds the car’s year model. The make field references a String object that holds the make of the car. The speed field is an int that holds the car’s current speed. In addition, the class should have the following constructor and other methods. The constructor should accept the car’s year and make as arguments. These values should be assigned to the object’s yearModel and make fields. The constructor should also assign 0 to the speed field. Constructor: The constructor should accept no arguments. Constructor: The constructor should accept the car’s year as argument.Assign the value to the object’s yearModel. No need to assign anything to the make and assign 0 to speed. Appropriate mutator methods should set the values in an object’s yearModel, make, and speed fields. Appropriate accessor methods should get the values stored in an object’s yearModel, make, and…arrow_forwardYou cannot use the isinstance function to determine whether an object is an instance of a subclass of a class.True or Falsearrow_forwardWhat do you call the technique that inserts an object to a function that can replicate the behavior of the object instead of merging objects with different classes? A. Polymorphism with an Object B. Abstraction C. Inheritance D. Encapsulationarrow_forward
- PLZ help with the following IN JAVA When defining an inner class to be a helper class for an outer class, the inner classes access should be marked as: Public Private Protected Package accessarrow_forwardTrue or false: A class may have a constructor with no parameter list, and an overloaded constructor whose parameters all take default arguments.arrow_forwardjava programming language You are part of a team writing classes for the different game objects in a video game. You need to write classes for the two human objects warrior and politician. A warrior has the attributes name (of type String) and speed (of type int). Speed is a measure of how fast the warrior can run and fight. A politician has the attributes name (of type String) and diplomacy (of type int). Diplomacy is the ability to outwit an adversary without using force. From this description identify a superclass as well as two subclasses. Each of these three classes need to have a default constructor, a constructor with parameters for all the instance variables in that class (as well as any instance variables inherited from a superclass) accessor (get) and mutator (set) methods for all instance variables and a toString method. The toString method needs to return a string representation of the object. Also write a main method for each class in which that class is tested – create…arrow_forward
- What method is automatically executed whenever a new instance of the class, which the method belongs to, has been instantiated? a. encapsulation b. abstraction c. polymorphismarrow_forwardC# Program Create program that computes student average Requirements:1. Use inheritance2. Use exception handling if applicable3. Create a class student4. Use the constructor method to collect the student information: name, math, science and english grade (User should be able to input student info)5. Create a method that computes the average6. Create another method that outputs (similarly to) the ff. when calledName: AnnaMath: 92Science: 92English: 92Average: 927. Instantiate a Student object and utilize the defined methods.arrow_forwardJAVA ENCAPSULATIONarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
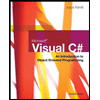
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
Introduction to Classes and Objects - Part 1 (Data Structures & Algorithms #3); Author: CS Dojo;https://www.youtube.com/watch?v=8yjkWGRlUmY;License: Standard YouTube License, CC-BY