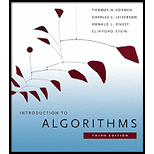
Introduction to Algorithms
3rd Edition
ISBN: 9780262033848
Author: Thomas H. Cormen, Ronald L. Rivest, Charles E. Leiserson, Clifford Stein
Publisher: MIT Press
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 11.1, Problem 2E
Program Plan Intro
To describe the application of bit
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
1.) Consider the problem of determining whether a DFA and a regular expression are
equivalent. Express this problem as a language and show that it is decidable.
ii) Let ALLDFA = {(A)| A is a DFA and L(A) = "}. Show that ALLDFA is decidable.
iii) Let AECFG = {(G)| G is a CFG that generates &}. Show that AECFG is decidable.
iv) Let ETM {(M)| M is a TM and L(M) = 0}. Show that ETM, the complement of
Erm, is Turing-recognizable.
Let X be the set {1, 2, 3, 4, 5} and Y be the set {6, 7, 8, 9, 10). We describe the
functions f: XY and g: XY in the following tables. Answer each part
and give a reason for each negative answer.
n
f(n)
n
g(n)
1
6
1
10
2
7
2
9
3
6
3
8
4
7
4
7
5
6
5
6
Aa. Is f one-to-one?
b. Is fonto?
c. Is fa correspondence?
Ad. Is g one-to-one?
e. Is g onto?
f.
Is g a correspondence?
vi) Let B be the set of all infinite sequences over {0,1}. Show that B is uncountable
using a proof by diagonalization.
Can you find the least amount of different numbers to pick from positive numbers (integers) that are at most 100 to confirm two numbers that add up to 101 when each number can be picked at most two times?
Can you find the formula for an that satisfies the provided recursive definition? Please show all steps and justification
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- What is the number of injective functions f from set {1,2,....,2n} to set {1,2,....,2n} so that f(x) >= x for all the 1<= x <= n?arrow_forwardIdeal MOSFET Current–Voltage Characteristics—NMOS Device and draw the circuitarrow_forward1. Create a Person.java file. Implement the public Person and Student classes in Person.java, including all the variables and methods in the UMLS. Person -name: String -street: String -city: String +Person(String name, String, street, String, city) +getName(): String +setName(String name): void +getStreet(): String +setStreet(String street): void +getCity(): String +setCity(String City): void +toString(): String Student -Id: int +Person(String name, String, street, String, city, int Id) +getId(): int +setId(int Id): void +toString(): String 2. Create a StudentTest.java file. Implement a public StudentTest class with a main method. In the main method, create one student object and print the object using System.out.println(). Your printing result must follow the example output: name: Mike, street: Morris Ave, city: Union, Id: 1000 Hint: You need to modify the toString methods in the Student class and Person class!arrow_forward
- 1) Apply the Paint Blue algorithm discussed in class to the following Finite Automata. a a a b b a COIS-3050H-R-W01-2025WI-COMB Formal Languages & Automata a b Show the status of the Finite Automata at the conclusion of the Paint Blue Algorithm (mark the visited states with an X and only include edges that have not been followed). 2) Use the pumping lemma to prove the following language is nonregular: L= {ab} = {abbb, aabbbbbb, aaabbbbbbbbb, ...}arrow_forward3) Find CFGs that for these regular languages over the alphabet Σ= {a, b}. Draw a Finite Automata e CFG. 1 COIS-3050H-R-W01-2025WI-COMB Formal anguages & Automata Is that contain the substring aba. (b) The language of all words that have an odd number letters and contains the string bb. (c) The language of all words that begin with the substring ba and contains an odd number of letters. 4) Convert the following FA into a PDA. a a S± b a a Ν Ꮓarrow_forwardCOIS-3050H-R-W01-2025WI-COMB Formal ministic PDA. Are the following words accepted by this Languages & Automata UI MIUSɩ that aTU I ed, indicate which state the PDA is in when the crash occurs. (a) aabbaa (b) aaabab (c) bababa Start (d) aaaabb A Accept Read₁ Push a (e) aaaaaa a b Read, Popi a a,b A Read₂ Accept A Pop₂arrow_forward
- 5) Eliminate the A-productions from the following CFG: Abc COIS-3050H-R-W01-2025WI-COMB Formal Languages & Automata BAabC C CaA | Bc | A 6) Convert the following CFG into CNF. S→ XYZ XaXbS | a |A YSbS | X | bb Z→ barrow_forwardNeed help answering these questions!1. Design a While loop that lets the user enter a number. The number should be multiplied by 10, and the result stored in a variable named product. The loop should iterate as long as the product contains a value less than 100. 2. Design a For loop that displays the following set of numbers: 0, 10, 20, 30, 40, 50 . . . 1000 3. Convert the While loop in the following code to a Do-While loop: Declare Integer x = 1 While x > 0 Display "Enter a number." Input x End Whilearrow_forwardNeed help with these:Design a While loop that lets the user enter a number. The number should be multiplied by 10, and the result stored in a variable named product. The loop should iterate as long as the product contains a value less than 100. 2. Design a For loop that displays the following set of numbers: 0, 10, 20, 30, 40, 50 . . . 1000 3. Convert the While loop in the following code to a Do-While loop: Declare Integer x = 1 While x > 0 Display "Enter a number." Input x End Whilearrow_forward
- Convert the While loop in the following code to a Do-While loop: Declare Integer x = 1 While x > 0 Display "Enter a number." Input x End Whilearrow_forwardPython - need help creating a python program that will sum the digits of a number entered by the user. For example if the user inputs the value 243 the program will output 9 because 2 + 4 + 3 = 9. The program should ask for a single integer from the user, it should then calculate the sum of all the digits of that number and output the result.arrow_forwardI need help with this in Python (with flowchart): Im creating a reverse guessing game. Then to choose a random number from 1 to 100 and the computer program will attempt to guess it, displaying the directions calculated or not. The guess will be displayed and the user will answer if it was correct or not. If correct, the game ends, if not then the computer asks if the guess was too high or too low. Finally inputting an answer and the computer generates a new guess within the proper range. Oh and to make sure the program doesnt guess outside of the ranges produced by the inputs of “too high” and “too low”. The program ending when the user guesses correctly or after the program takes 6 guesses. HELP ASAP!arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- New Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage LearningSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage LearningC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage LearningProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
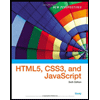
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:9781305503922
Author:Patrick M. Carey
Publisher:Cengage Learning
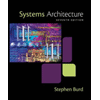
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning
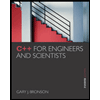
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
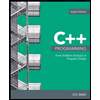
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
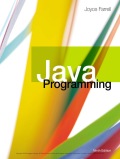
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT