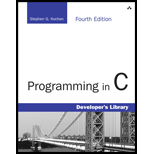
Programming in C
4th Edition
ISBN: 9780321776419
Author: Stephen G. Kochan
Publisher: Addison-Wesley
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Chapter 11, Problem 8E
Program Plan Intro
Program Plan:
- Include the necessary header files into program.
- Define the function named “bitSize()” which is used to fetch the size of the value.
- Declare the variables in type of unsigned int and int.
- Using “while” loop, check the value of “size”.
- If the value greater than “0”, right shift operation is performed and increments the “temp” value.
- Return the value of “temp-1”.
- Define the function named “no_of_bits()” which is used to calculate the minimum number of bits needed to represent the value.
- Declare and initialize the variables.
- Using “while” loop, check the value and left shift the value of “temp” and increment the “counter” value.
- Return the size which is reduced from counter.
- Define the function named “bitpat_get()” which is used to extract the “n” bits from source starting at “start” argument.
- Declare the variables.
- Call the “bitSize()” method and store the size into variable “size”.
- Initialize the value of “c” in type of integer.
- Using “if…else” condition, calculate the offset and temporary value.
- Return the temporary value to function.
- Define the function named “bitpat_set()” which is used to set the bit size within the source to value which is starting at “*start”.
- Declare the variables.
- Call the “no_of_bits()” method which passes “*start” as argument.
- Call the other appropriate methods and store in the variable.
- Using left shift operator to calculate the value of “val”.
- Print the appropriate result on screen.
- Define the main method.
- Declare the variables in type of unsigned integer.
- Prompt and get the values.
- Call the “bitpat_set()” method and print the result on screen.
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
2:21 m
Ο
21%
AlmaNet
WE ARE
HIRING
Experienced Freshers
Salesforce
Platform
Developer
APPLY NOW
SEND YOUR CV:
Email: hr.almanet@gmail.com
Contact: +91 6264643660
Visit: www.almanet.in
Locations: India, USA, UK, Vietnam
(Remote & Hybrid Options Available)
Provide a detailed explanation of the architecture on the diagram
hello please explain the architecture in the diagram below. thanks you
Chapter 11 Solutions
Programming in C
Ch. 11 - Type in and run the four programs presented in...Ch. 11 - Write a program that determines whether your...Ch. 11 - Prob. 3ECh. 11 - Using the result obtained in exercise 3, modify...Ch. 11 - Write a function called b that takes two...Ch. 11 - Write a function called b that looks for the...Ch. 11 - Write a function called b to extract a specified...Ch. 11 - Prob. 8E
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Complete the JavaScript function addPixels () to calculate the sum of pixelAmount and the given element's cssProperty value, and return the new "px" value. Ex: If helloElem's width is 150px, then calling addPixels (hello Elem, "width", 50) should return 150px + 50px = "200px". SHOW EXPECTED HTML JavaScript 1 function addPixels (element, cssProperty, pixelAmount) { 2 3 /* Your solution goes here *1 4 } 5 6 const helloElem = document.querySelector("# helloMessage"); 7 const newVal = addPixels (helloElem, "width", 50); 8 helloElem.style.setProperty("width", newVal); [arrow_forwardSolve in MATLABarrow_forwardHello please look at the attached picture. I need an detailed explanation of the architecturearrow_forward
- Information Security Risk and Vulnerability Assessment 1- Which TCP/IP protocol is used to convert the IP address to the Mac address? Explain 2-What popular switch feature allows you to create communication boundaries between systems connected to the switch3- what types of vulnerability directly related to the programmer of the software?4- Who ensures the entity implements appropriate security controls to protect an asset? Please do not use AI and add refrencearrow_forwardFind the voltage V0 across the 4K resistor using the mesh method or nodal analysis. Note: I have already simulated it and the value it should give is -1.714Varrow_forwardResolver por superposicionarrow_forward
- Describe three (3) Multiplexing techniques common for fiber optic linksarrow_forwardCould you help me to know features of the following concepts: - commercial CA - memory integrity - WMI filterarrow_forwardBriefly describe the issues involved in using ATM technology in Local Area Networksarrow_forward
- For this question you will perform two levels of quicksort on an array containing these numbers: 59 41 61 73 43 57 50 13 96 88 42 77 27 95 32 89 In the first blank, enter the array contents after the top level partition. In the second blank, enter the array contents after one more partition of the left-hand subarray resulting from the first partition. In the third blank, enter the array contents after one more partition of the right-hand subarray resulting from the first partition. Print the numbers with a single space between them. Use the algorithm we covered in class, in which the first element of the subarray is the partition value. Question 1 options: Blank # 1 Blank # 2 Blank # 3arrow_forward1. Transform the E-R diagram into a set of relations. Country_of Agent ID Agent H Holds Is_Reponsible_for Consignment Number $ Value May Contain Consignment Transports Container Destination Ф R Goes Off Container Number Size Vessel Voyage Registry Vessel ID Voyage_ID Tonnagearrow_forwardI want to solve 13.2 using matlab please helparrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrSystems ArchitectureComputer ScienceISBN:9781305080195Author:Stephen D. BurdPublisher:Cengage LearningC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
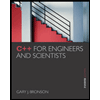
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
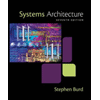
Systems Architecture
Computer Science
ISBN:9781305080195
Author:Stephen D. Burd
Publisher:Cengage Learning
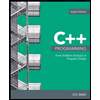
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
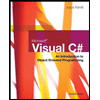
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
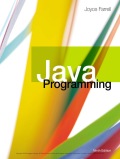
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT