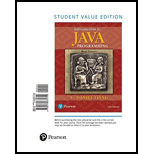
(Mersenne prime)A prime number is called a Mersenne prime if it can be written in the form 2p – 1 for some positive integer p. Write a
(Hint: You have to use BigInteger to store the number because it is too big to be stored in long. Your program may take several hours to run.)
p | 2^p – 1 |
2 | 3 |
3 | 7 |
5 | 31 |

Want to see the full answer?
Check out a sample textbook solution
Chapter 10 Solutions
Introduction to Java Programming and Data Structures: Brief Version (11th Global Edition)
Additional Engineering Textbook Solutions
Starting Out with C++ from Control Structures to Objects (9th Edition)
Starting Out with C++: Early Objects (9th Edition)
Introduction To Programming Using Visual Basic (11th Edition)
Java: An Introduction to Problem Solving and Programming (8th Edition)
Concepts Of Programming Languages
INTERNATIONAL EDITION---Engineering Mechanics: Statics, 14th edition (SI unit)
- A prime number is called a Mersenne prime if it can be writtenin the form 2p - 1 for some positive integer p. Write a program that findsall Mersenne primes with p ≤100 and displays the output as shown below.(Hint: You have to use BigInteger to store the number because it is too big tobe stored in long. Your program may take several hours to run.) p 2^p – 1---------------------2 33 75 31...arrow_forwardTask: A shop sells a range of mobile devices, SIM cards and accessories as shown in the table (see screenshot): Write a program algorithm for this shop. - Your program or programs must include appropriate prompts for the entry of data; data must be validated on entry. - Error messages and other output need to be set out clearly and understandably. - All arrays, variables, constants and other identifiers must have meaningful names. You will need to complete these three tasks. Task 1 – Setting up the system. Write a program to: - use appropriate data structures to store the item code, description and price information for the mobile devices, SIM cards and accessories; - allow the customer to choose a specific phone or tablet; - allow phone customers to choose whether the phone will be SIM Free or Pay As You Go; - allow the customer to choose a standard or luxury case; - allow the customer to choose the chargers required (none,…arrow_forwardQ1: Write a program that reads a set of N integers. Then, the program finds and outputs the sum of the even values and the sum of the odd values of them. For example: if the value entered for N=5, and the five integers are 3, 6, 2, 9, 7. Then the sum of even values (6+2 = 8), and the sum of odd values (3+9+7 = 19) Q2: Write a program that reads some numbers until it reaches a specific value (assume -1 is a stop value in this case). The program should find and outputs the average of all of the numbers (between 10...40) except the stop value (-1). For example: If the numbers look like: 12 5 30 48 -1. Then, the average of the numbers: (12+30)/2 = 21.arrow_forward
- /*code Kth Largest Factor A positive integer d is said to be a factor of another positive integer N if when N is divided by d, the remainder obtained is zero. For example, for number 12, there are 6 factors 1, 2, 3, 4, 6, 12. Every positive integer k has at least two factors, 1 and the number k itself.Given two positive integers N and k, write a program to print the kth largest factor of N. Input Format: The input is a comma-separated list of positive integer pairs (N, k). Output Format: The kth highest factor of N. If N does not have k factors, the output should be 1. Constraints: 1<N<10000000000 1<k<600. You can assume that N will have no prime factors which are larger than 13..arrow_forwardQUESTION 1 Write a program that computes and displays the five set of products of each three random numbers between 1 and 20 using only for loops (Don't use Arrays). Here is a sample run: The Product 1: of 2, 10, 8 is 160 The Product 2: of 11, 3, 20 is 660 The Product 3: of 5, 12, 8 is 480 The Product 4: of 3, 5, 7 is 105 The Product 5: of 12, 11, 18 is 2376arrow_forwardComputer Engineering labarrow_forward
- Programming language: JAVA Write a piece of code to generate 100 random characters (10 rows of 10 characters) and display them on the screen. -In a loop, generate a random number between 1 to 255 by using Math.random(). Use this number as an ASCII code for a character char c and then print c.arrow_forwardA Fibonacci Number sequence is the series of numbers: 0, 1, 1, 2, 3, 5, 8, 13, 21, 34, ... The next number is calculated by adding up the two numbers before it. Write a program called fib.cpp. The program will print out the n" Fibonacci Numbers using a function called: void FibonacciNumber (int n, int& fib_no) This function will calculate the n term in the Fibonacci Numbers and return in fib_no. By definition, the function returns 0 for n=1, and returns 1 for n = 2. For the subsequence number: nth = (n - 2)th + (n - 1)th You need to verify the input n > 0. Task 2 Sample Output Please enter the nth term Fibonacci number to compute: -1 Please enter a number greater than 0! Please enter the nth term Fibonacci number to compute: 12 The Fibonacci series is: 0, 1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89 The 12th. term is: 89arrow_forwardCODE IN JAVA design a program that grades arithmetic quizzes as follows: (Use the below startup code for Quizzes.java and provide code as indicated by the comments) 1. Ask the user how many questions are in the quiz. 2. Use a for loop to load the array. Ask the user to enter the key (that is, the correct answers). There should be one answer for each question in the quiz, and each answer should be an integer. They can be entered on a single line, e.g., 34, 7, 13, 100, 8 might be the key for a 5-question quiz. You will need to store the key in an array called "key". 3. Ask the user to enter the student's answers for the quiz to be graded. There needs to be one answer for each question. Note that each answer can simply be compared to the key as it is entered. If the answer is correct, add 1 to a correct answer counter. need this in a separate for loop from the loop used to load the array. 4. When the user has entered all of the answers to be graded, print the number correct…arrow_forward
- QuadraticAA.java Write a program that solves quadratic equations of the form , where The values A, B, and C will be real numbers (doubles) that you will request from the user. The equation below is what is used to solve for . Remember, there will be two solutions for x (not necessarily distinct). No loops are necessary, so don’t include any. To find the two solutions for this equation, you will use the quadratic formula: Remember that it must be solved for both the and : You will ask the user to input a value for A, B, and C (in that order, using those same letters), then you will use those values to find the two values of x as above. Your output MUST then display the original quadratic equation back to the user with their coefficients in place, and then output the two results. We will assume that the user will not put zero for A. *Format ALL OUTPUT VALUES (including the equation) to have only two decimal places. There is a test case below. Your program should run the…arrow_forwardCreate the following program using Python,you may use Replit for a free version of Python. Use loops to input a list of employee names and salaries and store them in parallel arrays. End the input with a sentinel value. The salaries should be floating point numbers and Salaries should be input in even hundreds. For example, a salary of 36,510 should be input as 36.5 and a salary of 69,030 should be entered as 69.0. Find the average of all the salaries of the employees. Find the highest and the lowest of all salaries. Display the following using proper labels: 1.Display the names and salaries of all the employees. 2. Display the average of all the salaries (for example, " The average of all salaries is $30000" ) 3. Display the highest of all the salaries 4. Display the lowest of all the salaries Please inculde header comments. If possible include step comments. Name of the submission - Employee Salaries Author of the submission - John Smith Summary/goal of the submission…arrow_forward(Python matplotlib or seaborn) CPU Usage We have the hourly average CPU usage for a worker's computer over the course of a week. Each row of data represents a day of the week starting with Monday. Each column of data is an hour in the day starting with 0 being midnight. Create a chart that shows the CPU usage over the week. You should be able to answer the following questions using the chart: When does the worker typically take lunch? Did the worker do work on the weekend? On which weekday did the worker start working on their computer at the latest hour? cpu_usage = [ [2, 2, 4, 2, 4, 1, 1, 4, 4, 12, 22, 23, 45, 9, 33, 56, 23, 40, 21, 6, 6, 2, 2, 3], # Monday [1, 2, 3, 2, 3, 2, 3, 2, 7, 22, 45, 44, 33, 9, 23, 19, 33, 56, 12, 2, 3, 1, 2, 2], # Tuesday [2, 3, 1, 2, 4, 4, 2, 2, 1, 2, 5, 31, 54, 7, 6, 34, 68, 34, 49, 6, 6, 2, 2, 3], # Wednesday [1, 2, 3, 2, 4, 1, 2, 4, 1, 17, 24, 18, 41, 3, 44, 42, 12, 36, 41, 2, 2, 4, 2, 4], # Thursday [4, 1, 2, 2, 3, 2, 5, 1, 2, 12, 33, 27, 43, 8,…arrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrC++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
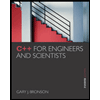
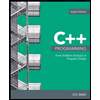