You've been hired by Encoding Egrets to write a C++ console application that encodes and decodes text. Use a validation loop to prompt for and get from the user a string that is up to ten characters in length. Create value function encodedString that takes the string as input and returns the equivalent encoded string. Start with an empty encoded string. Use a for loop to encode each character of the input string. Use the following
Control structures
Control structures are block of statements that analyze the value of variables and determine the flow of execution based on those values. When a program is running, the CPU executes the code line by line. After sometime, the program reaches the point where it has to make a decision on whether it has to go to another part of the code or repeat execution of certain part of the code. These results affect the flow of the program's code and these are called control structures.
Switch Statement
The switch statement is a key feature that is used by the programmers a lot in the world of programming and coding, as well as in information technology in general. The switch statement is a selection control mechanism that allows the variable value to change the order of the individual statements in the software execution via search.
You've been hired by Encoding Egrets to write a C++ console application that encodes and decodes text. Use a validation loop to prompt for and get from the user a string that is up to ten characters in length. Create value function encodedString that takes the string as input and returns the equivalent encoded string. Start with an empty encoded string. Use a for loop to encode each character of the input string. Use the following
- Convert the character to an integer (cast to int).
- Add 17 to the integer value.
- Mod the integer value by 256 to insure its stays within the character range 0-255.
- Convert the integer back to a character (cast back to char).
Create value function decodedString that takes the encoded string as input and returns the equivalent decoded string. It does the reverse of function encodedString. The original string and decoded string should be identical. Use formatted output manipulators (setw, left/right) to print the following rows:
- Input string.
- The encoded string (some characters may be unprintable).
- The decoded string.
And columns:
- A left-justified label.
- A left-justified string.
Declare global constants for the maximum string length (14), the shift value (17), the character boundary (256), and the column widths. The output should look like this:
Welcome to Encoding Egrets
--------------------------
Enter a string up to 14 characters in length to encode: Snow in November!
Error: the string is longer than 14 characters.
Enter a string up to 14 characters in length to encode: Hello World!
String: Hello World!
Encoded string: Yv}}Ç1hÇâ}u2
Decoded string: Hello World!
End of Encoding Egrets

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

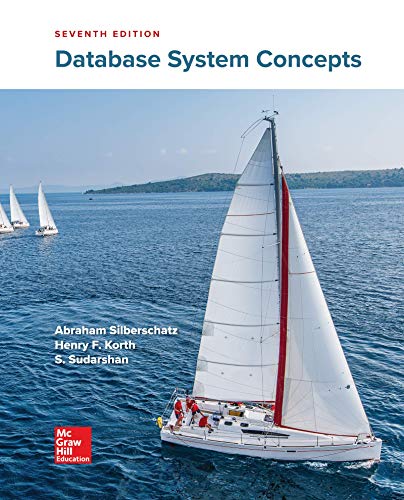
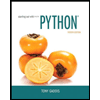
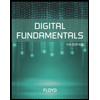
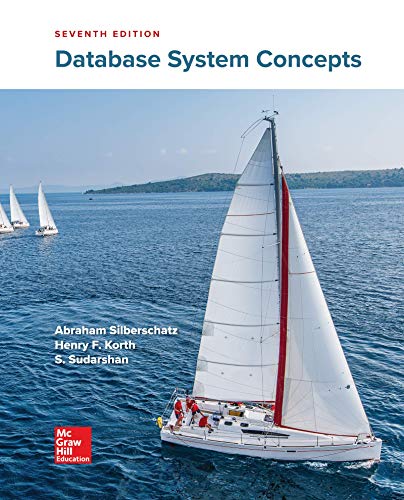
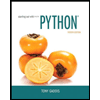
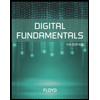
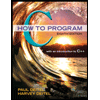
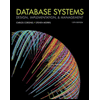
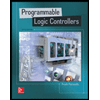