You're given an array arr. Apply the following algorithm to it: find intervals of consecutive prime numbers and consecutive non-prime numbers; replace each such interval with the sum of numbers in it; if the resulting array is different from the initial one, return to step 1, otherwise return the result. Input A non-empty integer array such that: -10000 ≤ arr[i] ≤ 10000 1 ≤ arr.length ≤ 1000 Output An integer array. Examples For arr = [1, 2, 3, 5, 6, 4, 2, 3] the result should be [21, 5]: [1, 2, 3, 5, 6, 4, 2, 3] --> [(1), (2 + 3 + 5), (6 + 4), (2 + 3)] --> [1, 10, 10, 5] [1, 10, 10, 5] --> [(1 + 10 + 10), (5)] --> [21, 5] For arr = [-3, 4, 5, 2, 0, -10] the result should be [1, 7, -10]: [-3, 4, 5, 2, 0, -10] --> [(-3 + 4), (5 + 2), (0 + -10)] --> [1, 7, -10] Solve it in C# please
You're given an array arr. Apply the following
find intervals of consecutive prime numbers and consecutive non-prime numbers;
replace each such interval with the sum of numbers in it;
if the resulting array is different from the initial one, return to step 1, otherwise return the result.
Input
A non-empty integer array such that:
-10000 ≤ arr[i] ≤ 10000
1 ≤ arr.length ≤ 1000
Output
An integer array.
Examples
For arr = [1, 2, 3, 5, 6, 4, 2, 3] the result should be [21, 5]:
[1, 2, 3, 5, 6, 4, 2, 3] --> [(1), (2 + 3 + 5), (6 + 4), (2 + 3)] --> [1, 10, 10, 5]
[1, 10, 10, 5] --> [(1 + 10 + 10), (5)] --> [21, 5]
For arr = [-3, 4, 5, 2, 0, -10] the result should be [1, 7, -10]:
[-3, 4, 5, 2, 0, -10] --> [(-3 + 4), (5 + 2), (0 + -10)] --> [1, 7, -10]
Solve it in C# please

Step by step
Solved in 5 steps with 3 images

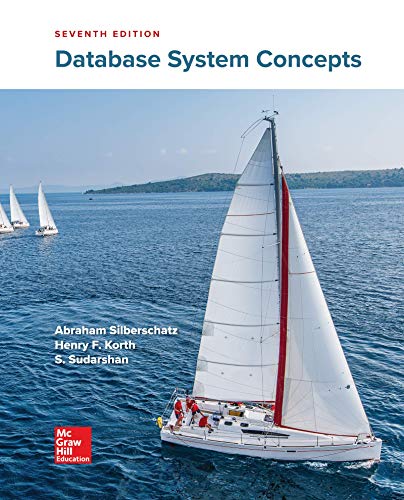
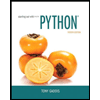
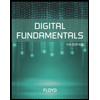
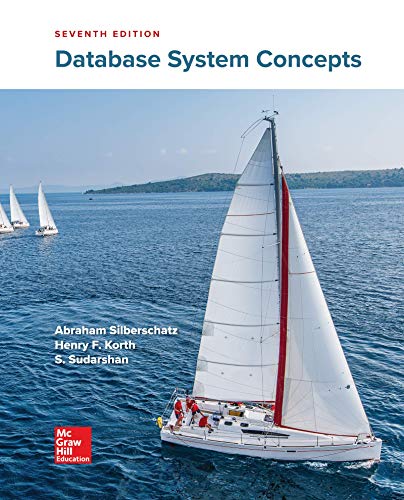
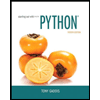
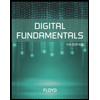
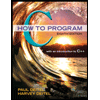
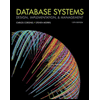
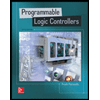