Your program should be in a new project named "Program 2: Credit Card Validation" and should be in a package named "creditCard". Your program must be in a file called "Program2CCValidation". Write a Java program that will: issue a 4 digit random PIN (1111 to 9999) for a credit card. The program that will verify that the credit card number is valid using the Luhn Algorithm. User must be prompted to enter their card number until a valid number is entered. A PIN will be issued only if the card is valid. (note, that we are checking for valid numbers only, NOT checking for specific card types, such as Visa, Mastercard, Diner's Club, etc), so there is no need to deal with different lengths of credit card numbers. Most credit cards numbers are 16 digits in length, so the number MUST be read in as String, rather than an int. MAKE NO ASSUMPTIONS ABOUT THE LENGTH OF THE STRING! Information on Luhn Algorithm https://www.freeformatter.com/credit-card-number-generator-validator.html (Links to an external site.) Print appropriate messages to let the user know if the card is valid or not valid. Use of the Random class is required, and you may not use arrays. You will find the following comments helpful to guide your work (It is a requirement that you must include them in the main method and follow them step-by-step!) : //set up a Scanner //set up a boolean named validCreditCard and set it to false //loop while not a valid credit card //prompt the user for a potential credit card number //Get the credit card number as a String - store in potentialCCN (use scanner's nextLine) //use Luhn to validate credit card using the following steps: //store the last digit AS AN INT for later use in lastDigit (probably requires Integer.parseInt(String) //TEST then comment out! - check the last digit - System.out.println(lastDigit); //remove the last digit from potentialCCN and store in potentialCCN using String's substring //TEST then comment out! - check potentialCCN - System.out.println(potentialCCN); //create reversedCCN as a empty String by: //reverse the digits using a for loop by adding characters to reversedCCN //end the reverse the string loop //TEST then comment out! - check reversedCCN - System.out.println(reversedCCN); //set boolean isOddDigit to false //set up an integer called current digit and set it to 0 //create an integer named sum and set it to 0 //multiply the digits in odd positions by 2, then subtract 9 from any number higher than 9 using: //(for loop running 0 to less than length of reversed CCN) //set currentDigit equal to the integer version of the current character //Test then comment out! - currentDigit -System.out.print(currentDigit); //toggle isOddDigit //if isOddDigit //multiply currentDigit by 2 and store in currentDigit //if currentDigit > 9, subtract 9 from currentDigit and store in currentDigit //TEST then comment out - Check currentDigit - System.out.println(currentDigit); }//end if isOddD //Comment in this segment for a useful test output string //System.out.print(currentDigit); //if (i>=reversedCCN.length()-1) //System.out.println(); //else //System.out.print(" + "); //end of segment // add currentDigit to sum and store in sum }//end the for loop that runs from 0 to less than the length of reversed CCN //TEST and comment out - System.out.println("sum:"+sum) //if the last digit of sum + the last Digit ends in a zero, set validCreditCard to true //end if //if validCreditCard is false, output an appropriate message //end if }//end while that checks for valid credit card //issue a RANDOM PIN and print it out - no more help :) Additional Restrictions: You may not use Math.random or the two-argument form of Random.nextInt (use the one-argument form ONLY) Your program should work despite the length of the credit card number (it should work for credit card lengths of 10 as well as 16). Be sure to comment out all the debugging output before submission!
Your program should be in a new project named "Program 2: Credit Card Validation" and should be in a package named "creditCard". Your program must be in a file called "Program2CCValidation". Write a Java program that will: issue a 4 digit random PIN (1111 to 9999) for a credit card. The program that will verify that the credit card number is valid using the Luhn
Information on Luhn Algorithm https://www.freeformatter.com/credit-card-number-generator-validator.html (Links to an external site.) Print appropriate messages to let the user know if the card is valid or not valid. Use of the Random class is required, and you may not use arrays. You will find the following comments helpful to guide your work (It is a requirement that you must include them in the main method and follow them step-by-step!) :
//set up a Scanner
//set up a boolean named validCreditCard and set it to false
//loop while not a valid credit card
//prompt the user for a potential credit card number
//Get the credit card number as a String - store in potentialCCN (use scanner's nextLine)
//use Luhn to validate credit card using the following steps:
//store the last digit AS AN INT for later use in lastDigit (probably requires Integer.parseInt(String)
//TEST then comment out! - check the last digit - System.out.println(lastDigit);
//remove the last digit from potentialCCN and store in potentialCCN using String's substring
//TEST then comment out! - check potentialCCN - System.out.println(potentialCCN);
//create reversedCCN as a empty String by:
//reverse the digits using a for loop by adding characters to reversedCCN
//end the reverse the string loop
//TEST then comment out! - check reversedCCN - System.out.println(reversedCCN);
//set boolean isOddDigit to false
//set up an integer called current digit and set it to 0
//create an integer named sum and set it to 0
//multiply the digits in odd positions by 2, then subtract 9 from any number higher than 9 using:
//(for loop running 0 to less than length of reversed CCN)
//set currentDigit equal to the integer version of the current character
//Test then comment out! - currentDigit -System.out.print(currentDigit);
//toggle isOddDigit
//if isOddDigit
//multiply currentDigit by 2 and store in currentDigit
//if currentDigit > 9, subtract 9 from currentDigit and store in currentDigit
//TEST then comment out - Check currentDigit - System.out.println(currentDigit);
}//end if isOddD
//Comment in this segment for a useful test output string
//System.out.print(currentDigit);
//if (i>=reversedCCN.length()-1)
//System.out.println();
//else
//System.out.print(" + ");
//end of segment
// add currentDigit to sum and store in sum
}//end the for loop that runs from 0 to less than the length of reversed CCN
//TEST and comment out - System.out.println("sum:"+sum)
//if the last digit of sum + the last Digit ends in a zero, set validCreditCard to true
//end if
//if validCreditCard is false, output an appropriate message
//end if
}//end while that checks for valid credit card
//issue a RANDOM PIN and print it out - no more help :)
Additional Restrictions:
You may not use Math.random or the two-argument form of Random.nextInt (use the one-argument form ONLY)
Your program should work despite the length of the credit card number (it should work for credit card lengths of 10 as well as 16).
Be sure to comment out all the debugging output before submission!

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

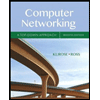
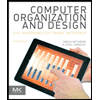
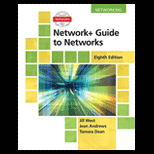
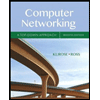
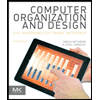
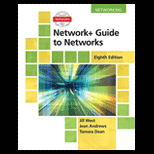
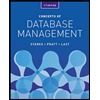
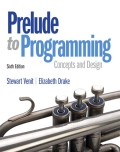
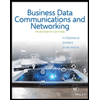