Your program must first ask the user for the board dimensions. Then, the program asks the user if the computer is to be the Black or White player. For this lab, we will assume that the Black player always gets the first move. So, if the computer is black, then the computer should make the first move; otherwise, the program prompts the human player to make the first move. The board is printed after every moe. Once the first move is out of the way, the turns proceed as described above, alternating between Black and White unless one of the players has no move to make, which case your program should print a message "W player has no valid move." (ie. for the case of the White player) and should prompt the Black player for another move. After each turn, your program must print the board, and must detect whether the game has been won, or whether there is a draw. If your program detects the game is over (ie. a win or a draw), a message is printed and the program terminates. The specific messages to print are: "W player wins.", "B player wins." or "Draw!". If the human player makes an illegal move, your program must detect this, print an error message, and end the game, declaring the winner (with the corresponding message above).
Your program must first ask the user for the board dimensions. Then, the program asks the user if the computer is to be the Black or White player. For this lab, we will assume that the Black player always gets the first move. So, if the computer is black, then the computer should make the first move; otherwise, the program prompts the human player to make the first move. The board is printed after every moe. Once the first move is out of the way, the turns proceed as described above, alternating between Black and White unless one of the players has no move to make, which case your program should print a message "W player has no valid move." (ie. for the case of the White player) and should prompt the Black player for another move. After each turn, your program must print the board, and must detect whether the game has been won, or whether there is a draw. If your program detects the game is over (ie. a win or a draw), a message is printed and the program terminates. The specific messages to print are: "W player wins.", "B player wins." or "Draw!". If the human player makes an illegal move, your program must detect this, print an error message, and end the game, declaring the winner (with the corresponding message above).
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question

Transcribed Image Text:A few important notes:
1. It is likely that two or more squares may end up having the same score. In that case your
program must choose the position with the lower row. If there are two positions with the
same score in the same row, your program must choose the solution with the lower column.
You can ensure this happens easily by the order in which you compute the score and test to
see if it is the best.
2. Your program must be able to work with the computer being either the Black or the White
player.
Here is a sample execution of the program (your program must conform to this output). Notice that,
close to the end, the computer (playing White) has no valid move, and the turn returns to the human
player (playing Black).
Enter the board dimension: 4
Computer plays (B/W): W
abcd
a UUUU
b UWBU
C UBWU
d UUUU
Enter move for colour B (Rowcol): ba
abcd
a UUUU
b BBBU
C UBWU
d UUUU
Computer places W at aa.
abcd
a WUUU
b BWBU
c UBWU
d UUUU
Enter move for colour B (RowCol): ab
abcd
a WBUU
b BBBU
C UBWU
d UUUU
Computer places W at ac.
abcd
a WWWU
b BBWU
C UBWU
d UUUU
Enter move for colour B (RowCol): bd
abcd
a WWWU
b BBBB
C UBWU

Transcribed Image Text:The Game Flow and Prescribed Algorithm
Your program must first ask the user for the board dimensions. Then, the program asks the user
if the computer is to be the Black or White player. For this lab, we will assume that the Black
player always gets the first move. So, if the computer is black, then the computer should make the
first move; otherwise, the program prompts the human player to make the first move. The board is
printed after every move.
Once the first move is out of the way, the turns proceed as described above, alternating between
Black and White unle ss one of the players has no move to make, which case your program should
print a message "W player has no valid move." (i.e. for the case of the White player) and should
prompt the Black player for another move. After each turn, your program must print the board, and
must detect whether the game has been won, or whether there is a draw. If your program detects the
game is over (ie. a win or a draw), a message is printed and the program terminates. The specific
messages to print are: "W player wins.", "B player wins." or "Draw!". If the human player
makes an illegal move, your program must detect this, print an error message, and end the game,
declaring the winner (with the corresponding message above).
Part 1: Implementing the Prescribed Algorithm
How Should the Computer Make Moves?
The method for the computer to make a move is as follows: for each possible place it could make
a move, it should compute a number, called a "score" that is larger when a square is a better place
for the computer to lay a tile. As these scores are computed for each square, it should keep track of
which one is the highe st, and use that square as the move. (See below on what to do in the event of
a tied score).
The score for each candidate position is defined as the total number of the human player's tiles that
would be flipped if the computer were to lay a tile at that position. The figure below shows the
scores if the computer were the White player.
2 1
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 1 images

Recommended textbooks for you
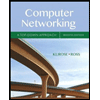
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
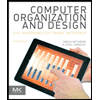
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
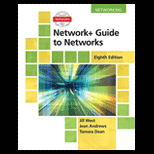
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
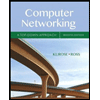
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
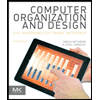
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
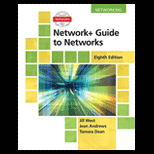
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
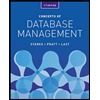
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
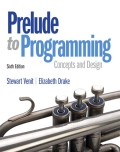
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
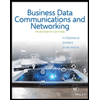
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY