You'll have to use the Scanner class to get input from the user. Scanner allows you to specify if the entry should be treated as a String or as an Integer, etc. When the user is expected to enter an Integer (as is the case here), use the nextInt() method. For example: scanner.nextInt(). This is different than the nextLine() method. There's a wrinkle in this problem. You are to assume that the integer that is being entered is in Binary form. That means that if the user enters 10 they don't mean "ten". They mean "two". To do this, you have to modify the scanner object to use a different "radix". This is also known as a base. A regular number has a radix of 10. We often call these 'decimal numbers or "base 10" numbers because we count in groups of ten. A binary number has a radix of 2 because you count in groups of two: 0 then 1. How do you do this? Use the radix() method. What does a binary number look like? 0 is 0. 1 is 1. The number 2? It's 10. And three? It's 11. Seven is 111. Eight 1000
You'll have to use the Scanner class to get input from the user. Scanner allows you to specify if the entry should be treated as a String or as an Integer, etc. When the user is expected to enter an Integer (as is the case here), use the nextInt() method. For example: scanner.nextInt(). This is different than the nextLine() method. There's a wrinkle in this problem. You are to assume that the integer that is being entered is in Binary form. That means that if the user enters 10 they don't mean "ten". They mean "two". To do this, you have to modify the scanner object to use a different "radix". This is also known as a base. A regular number has a radix of 10. We often call these 'decimal numbers or "base 10" numbers because we count in groups of ten. A binary number has a radix of 2 because you count in groups of two: 0 then 1. How do you do this? Use the radix() method. What does a binary number look like? 0 is 0. 1 is 1. The number 2? It's 10. And three? It's 11. Seven is 111. Eight 1000
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question

Transcribed Image Text:1 import java.util.Scanner;
T
2
3
4
5
6-
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
4142789
25
26
Y
public class preLabC {
public static Integer myMethod(String repeat ThisValue) {
System.out.println("The computer wants you to enter the following binary number: + repeat ThisValue);
// Step 1. Add in a line here to accept a new Scanner object.
You're done this before... var
//
30
31
32
33
34 }
}
something something... Scanner(System.in);
// what goes here?
// Step 2. Tell Java that the thing you're going to scan in will be in Binary form (only 1's and 0's)
ObjectName DOT useRadix( SOME_NUMBER);
//
//
what number should you use in the parentheses? 2 for Binary.
// the scanned object should be in binary (Radix 2)
// Step 3. Scan the values from the user. It's going to return an Integer, not a String.
//
Integer binary Integer scannedObject.nextInt(); // capture the user entry as an Integer Object.
System.out.println("In this black VPL box, enter a binary number: ");
System.out.println("You entered " + binaryInteger +
which in binary is
return binary Integer;
"
+ Integer.toBinaryString(binaryInteger) );
A String showing a Binary number will be passed to your
method.
You should have the user (you, in this case) type out a Binary
number within the VPL window like this...
Description
→ Submission
✪A
8
9
10
preLabC.java
1- import java.util.Scanner;
2
3
4- public class preLabC (
5
6-
7
19
Submissions list
<> Edit
Similarity
Submission view
?
method.
A Test activity
Grade
public static Integer myMethod(String repeat ThisValue){
> Console: connected (Running: 9 seg)
JUnit version 4.11
.Test
computer wants you to enter the following binary number: 11
Previous s11
System.out.println("The computer wants you to enter the following
new Scanner(System.in);
cennedObject useRodix // the scanned object should be in binary (Radix 2)
Integer binaryInteger scannedobject.nextInt(); // capture the user entry as an integer.
Type your binary
number here.
Then hit enter or return.
System.out.println("In this black VPL box, enter a binary number: ");
System.out.println("You entered" + binaryInteger", which in binary is + Integer.toBinaryString(binary Integer));
You'll have to use the Scanner class to get input from the
user. Scanner allows you to specify if the entry should be
treated as a String or as an Integer, etc.
When the user is expected to enter an Integer (as is the case
here), use the nextInt() method. For example:
scanner.nextInt(). This is different than the nextLine()
There's a wrinkle in this problem. You are to assume that the
integer that is being entered is in Binary form. That means
that if the user enters 10 they don't mean "ten". They mean
"two". To do this, you have to modify the scanner object to
use a different "radix". This is also known as a base. A
regular number has a radix of 10. We often call these
'decimal" numbers or "base 10" numbers because we count in
groups of ten. A binary number has a radix of 2 because you
count in groups of two: 0 then 1.
How do you do this? Use the radix() method.
What does a binary number look like? 0 is 0. 1 is 1. The
number 2? It's 10. And three? It's 11. Seven is 111. Eight
is 1000.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
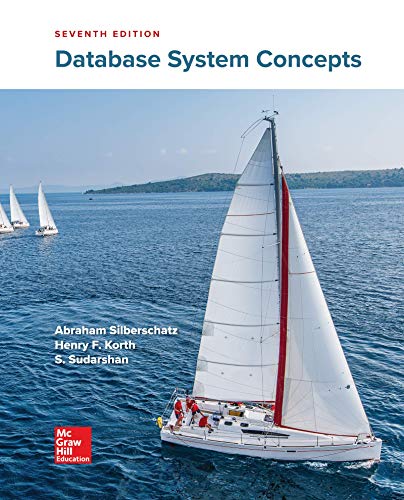
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
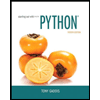
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
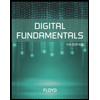
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
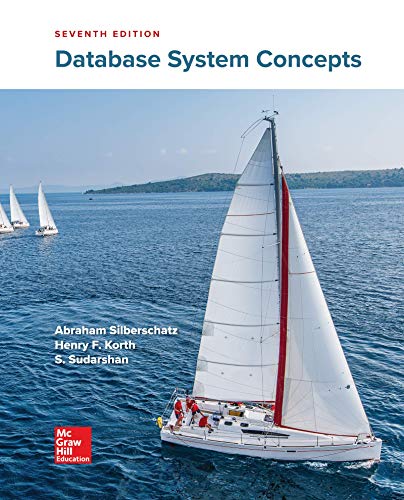
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
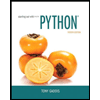
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
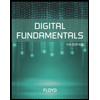
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
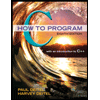
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
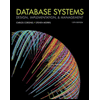
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
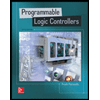
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education