You will implement the following functions: /** * Reads from standard input a list of Short Tandem Repeat (STRS) * and their known counts for several individuals * @param name STRS @param name Individuals. * @param STRcounts * * the DNA sequence for each individual * @pre * @post **/ void readData (vector & name STRS, vector& name Individuals, vector>& STRcounts) For example, consider the input: 3 AGAT AATG TATC Alice 5 28 Bob 3 7 4 Charlie 6 1 5 nameSTRS, name Individuals, and name STRS are empty nameSTRS, name Individuals and STRcounts are populated with data read from stdin It shows, in the first line, the number of STRs followed by the names of those STRS, which will be populated into the vector nameSTRs. The remaining lines contain data for a number of individuals. Their names will be populated into the vector name Individuals and the longest consecutive counts of STRS will be stored in the 2D vector STRcounts (which is a vector of vector of ints). Elements in a 2D vector are vector themselves. Check this resource for learning more about 2D Vectors in C++. /** the STRS (eg. AGAT, AATG, TATC) the names of individuals (eg. Alice, Bob, Charlie) the count of the longest consecutive occurrences of each STR in Note, that an empty line at the end of the input denotes the end of data. In other words, the code should stop reading names and STR counts as soon as an empty line is encountered. * Prints a list of Short Tandem Repeat (STRS) and their * known counts for several individuals * * @param * @param * @param nameSTRS name Individuals the STRS (eg. AGAT, AATG, TATC) the names of individuals (eg. Alice, Bob, Charlie) the STR counts. STRcounts * @pre nameSTRS, name Individuals, and STRcounts hold the data intended to be printed * @post the name of individuals and their STR counts in a column-major format are printed to stdout **/ void printData (vector& nameSTRs, vector & nameIndividuals, vector>&
You will implement the following functions: /** * Reads from standard input a list of Short Tandem Repeat (STRS) * and their known counts for several individuals * @param name STRS @param name Individuals. * @param STRcounts * * the DNA sequence for each individual * @pre * @post **/ void readData (vector & name STRS, vector& name Individuals, vector>& STRcounts) For example, consider the input: 3 AGAT AATG TATC Alice 5 28 Bob 3 7 4 Charlie 6 1 5 nameSTRS, name Individuals, and name STRS are empty nameSTRS, name Individuals and STRcounts are populated with data read from stdin It shows, in the first line, the number of STRs followed by the names of those STRS, which will be populated into the vector nameSTRs. The remaining lines contain data for a number of individuals. Their names will be populated into the vector name Individuals and the longest consecutive counts of STRS will be stored in the 2D vector STRcounts (which is a vector of vector of ints). Elements in a 2D vector are vector themselves. Check this resource for learning more about 2D Vectors in C++. /** the STRS (eg. AGAT, AATG, TATC) the names of individuals (eg. Alice, Bob, Charlie) the count of the longest consecutive occurrences of each STR in Note, that an empty line at the end of the input denotes the end of data. In other words, the code should stop reading names and STR counts as soon as an empty line is encountered. * Prints a list of Short Tandem Repeat (STRS) and their * known counts for several individuals * * @param * @param * @param nameSTRS name Individuals the STRS (eg. AGAT, AATG, TATC) the names of individuals (eg. Alice, Bob, Charlie) the STR counts. STRcounts * @pre nameSTRS, name Individuals, and STRcounts hold the data intended to be printed * @post the name of individuals and their STR counts in a column-major format are printed to stdout **/ void printData (vector& nameSTRs, vector & nameIndividuals, vector>&
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
C++ Code

Transcribed Image Text:You will implement the following functions:
/**
* Reads from standard input a list of Short Tandem Repeat (STRS)
and their known counts for several individuals
*
* @param
the STRS (eg. AGAT, AATG, TATC)
@param
the names of individuals (eg. Alice, Bob, Charlie)
* @param STRcounts
the count of the longest consecutive occurrences of each STR in
the DNA sequence for each individual
* @pre
nameSTRS, name Individuals, and name STRS are empty
@post nameSTRS, name Individuals and STRcounts are populated with data read from stdin
*
name
For example, consider the input:
AGAT
AATG
TATC
**/
void readData(vector<string> & nameSTRS, vector<string>& name Individuals, vector<vector<int>>&
STRcounts)
3 AGAT AATG TATC
Alice 5 28
Bob 3 7 4
Charlie 6 15
It shows, in the first line, the number of STRs followed by the names of those STRS, which will be populated into the vector nameSTRs. The
remaining lines contain data for a number of individuals. Their names will be populated into the vector name Individuals and the longest
consecutive counts of STRS will be stored in the 2D vector STRcounts (which is a vector of vector of ints). Elements in a 2D vector are
vector themselves. Check this resource for learning more about 2D Vectors in C++.
Note, that an empty line at the end of the input denotes the end of data. In other words, the code should stop reading names and STR
counts as soon as an empty line is encountered.
nameSTRS
name Individuals
/**
Prints a list of Short Tandem Repeat (STRS) and their
*known counts for several individuals
* @param
@param
* @param
This function will print out the information that has been previously read (using the function readData) in a format that aligns an
individual's STR counts along a column. For example, the output for the above input will be:
STRcounts
@pre
nameSTRS, nameIndividuals, and STRcounts hold the data intended to be printed
@post the name of individuals and their STR counts in a column-major format are printed to
stdout
**/
void printData(vector<string> & nameSTRS, vector<string> & name Individuals, vector<vector<int>>&
STRcounts)
Alice
nameSTRS
the STRS (eg. AGAT, AATG, TATC)
name Individuals. the names of individuals (eg. Alice, Bob, Charlie)
the STR counts.
5
2
8
Bob
3
7
4
Charlie
6
1
5

Transcribed Image Text:This output uses text manipulators to left-align each name and counts within 10 characters. The row of dashes is set to 40 characters.
/**
*
*
*
@param
sequence a DNA sequence of an individual
@param nameSTRS the STRS (eg. AGAT, AATG, TATC)
* @returns the count of the longest consecutive occurrences of each STR in nameSTRS
*
Computes the longest consecutive occurrences of several STRS in a DNA sequence
**/
vector<int> getSTRcounts (string& sequence, vector<string>& name STRs)
For example, if the sequence is
AACCCTGCGCGCGCGCGATCTATCTATCTATCTATCCAGCATTAGCTAGCATCAAGATAGATAGATGAATTTCGAAATGAATGAATGAATGAATGAATGA
and the vector namesSTRS is {"AGAT", "AATG", "TATC"}, then the output is the vector {3, 7, 4}
/**
* Compares if two vectors of STR counts are identical or not
*
*
@param countQuery STR counts that is being queried (such as that computed from an input
DNA sequence)
*
@param count DB STR counts that are known for an individual (such as that stored in a
database)
@returns a boolean indicating whether they are the same or not
**/
bool compare STRcounts (vector<int>& countQuery, vector<int>& countDB)
For example, if countQuery is the vector {3, 7, 4), and countDB is the vector {3, 3, 4), the function returns false.
Expert Solution

Step 1: Overview
The question asks for a C++ program that can read, process, and display Short Tandem Repeat (STR) data. It specifies the functions required to read input, print data in a tabular format, calculate STR counts from a DNA sequence, and compare STR counts. The program should also include user prompts for input.
Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 4 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
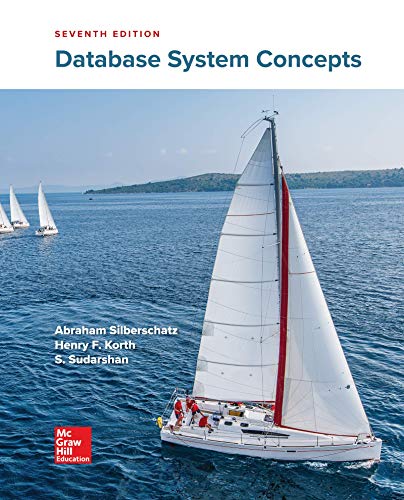
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
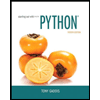
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
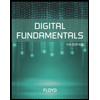
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
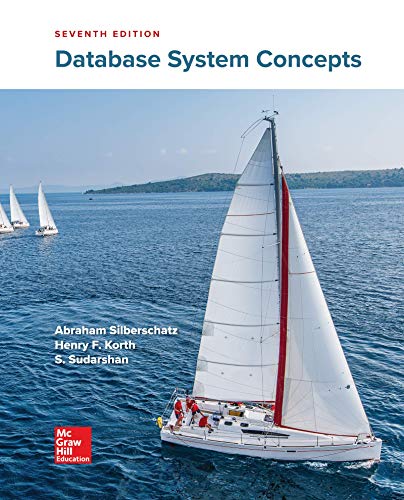
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
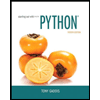
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
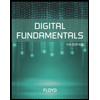
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
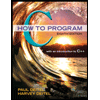
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
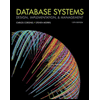
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
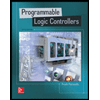
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education