You need to write three functions in util.py. When they are done correctly, linreg_mi.py will un unchanged. The three functions are: • read_excel_data which reads in the excel file and returns X, Y, and labels. Y is a 1- dimentional numpy array containing the last column of the spreadsheet. X is a 2-dimensional numpy array that contains the data in the other columns and the first column is filled with 1s. labels is a list of strings from the header in the spreadsheet. format_prediction which takes B (the vector of coefficients) and labels that you created in read_excel_data. Then it returns a string like this: predicted price = $32,362.85+ ($85.61 x sqft_hvac) + ($2.73 x sqft_yard) + ($59,195.07 x bedrooms) + ($9,599.24 x bathro ($-17,421.84 x miles_to_school)} • score that takes B, X, and Y and returns the R² score.
SQL
SQL stands for Structured Query Language, is a form of communication that uses queries structured in a specific format to store, manage & retrieve data from a relational database.
Queries
A query is a type of computer programming language that is used to retrieve data from a database. Databases are useful in a variety of ways. They enable the retrieval of records or parts of records, as well as the performance of various calculations prior to displaying the results. A search query is one type of query that many people perform several times per day. A search query is executed every time you use a search engine to find something. When you press the Enter key, the keywords are sent to the search engine, where they are processed by an algorithm that retrieves related results from the search index. Your query's results are displayed on a search engine results page, or SER.

![12:21
Share
Business card Sep 11, 2022
Done
linreg_mi (3 of 6)
import numpy as np
import pandas as pd
import sys
import util
# Check command line
if len(sys.argv) != 2:
print (f"{sys.argv[0]} <xlsx>")
exit (1)
#Read in the argument
infilename = sys.argv[1]
# Read the spreadsheet
X, Y, labels util.read_excel_data(infilename)
n, d= X. shape
print (f"Read {n} rows, {d-1} features from
{infilename)",")
# Find the coefficients for the linear
regression
B = np.linalg.inv(X.T @ X) @ X.T @ Y
# Pretty print them
print (util.format_prediction (B, labels))
R2 = util.score (B, X, Y)
print (f"R2 (R2: f}")
Done
import pandas as pd
import numpy as np.
util (6 of 6)
#Read in the excel file
# Returns:
#X: first column is 1s, the rest are from the
spreadsheet
#Y: The last column from the spreadsheet
#
labels: The list of headers for the columns
of X from the spreadsheet
def read_excel_data(infilename):
## Your code here
return X, Y, labels
Make it pretty
def format prediction (B, labels):
## Your code here
return pred_string
Modify
# Return the R2 score for coefficients B
#Given inputs X and outputs Y
def score (B, X, Y):
## Your code here
return R2
EK
Text actions
20
Save contact
More](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F3b8f61c3-b57f-4943-8538-4e0823d8c6e6%2Fce2eb1a2-8dd4-4ac0-b088-1a47fafd656a%2Fuvv8gin_processed.jpeg&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

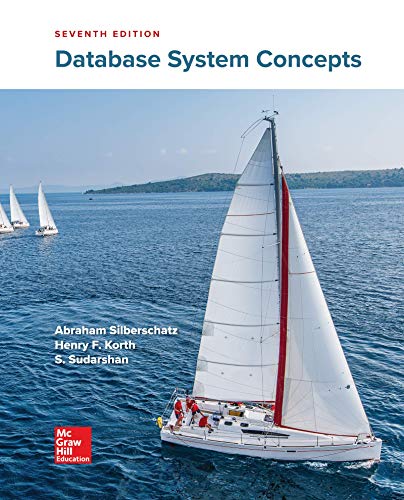
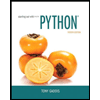
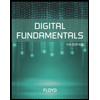
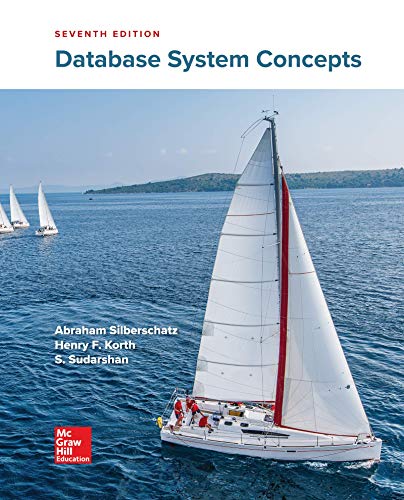
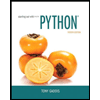
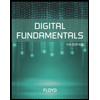
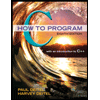
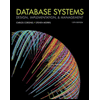
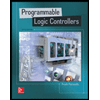