You may use the Java Libraries for solving this problem. We recommend using java.util.* The star “ * ” acts as a wildcard and allows you to use the entire java.util library. The heap presented in the screenshot is also known as a max-heap, in which each node is greater than or equal to any of it's children. A min-heap is a heap in which each node is less than or equal to any of its children. Min-heaps are often used to implement priority queues. Revise the Heap class in the screenshot to implement a min-heap. (The max heap example begins on the screenshot that says "Listings 23.9" at the top). Use the following logic:
PLEASE READ THIS FIRST! _____________________
Please write in java. Add comments but make the comments short. No need for really long comments. Please keep code very neat and simple , dont add anything unneccesary in the code if you weren't instructed to do so. If youve answered this before please dont copy and paste from a previous question! (Rewrite it in another way)..... Also be sure to read the instructions below carefully. The two files that are attached are screenshots of the textbook's MAX-HEAP Example. You will be creating a mini-heap from that example. But the Instructions below explains everything you'll be doing. NO THIS is not a homework assignment, it's practice work. Please type the code out so that I can copy and paste it into my IDE and see the output for my self but also still provide a screenshot of your output!
INSTRUCTIONS FOR QUESTION BELOW!!_____________________________
You may use the Java Libraries for solving this problem. We recommend using java.util.* The star “ * ” acts as a wildcard and allows you to use the entire java.util library.
The heap presented in the screenshot is also known as a max-heap, in which each node is greater than or equal to any of it's children. A min-heap is a heap in which each node is less than or equal to any of its children. Min-heaps are often used to implement priority queues. Revise the Heap class in the screenshot to implement a min-heap. (The max heap example begins on the screenshot that says "Listings 23.9" at the top).
Use the following logic:
- Write a main method that will accept 5 numbers, and put them in a min-heap
- Remove them from the min heap, printing one at a time as they are removed, to show that the list is sorted
Hint: The screenshotted textbook example is for a MAX heap; you must alter that example to reflect a MIN heap – think about it !

![internal heap representation
no-arg constructor
constructor
add a new object
append the object
swap with parent
heap now
remove the root
empty heap
root
new root
remove the last
adjust the tree
LISTING 23.9 Heap.java
1 public class Heap<E extends Comparable<E>> {
2 private java.util.ArrayList<E> list = new java.util.ArrayListo():
3
4
5
6
7
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
/** Create a default heap */
public Heap() {
/* Create a heap from an array of objects */
public Heap (E[] objects) {
for (int i = 0; i <objects.length; i++)
add(objects[1]);
}
/** Add a new object into the heap /
public void add(E newObject) {
list.add(newObject); // Append to the heap
int currentIndex-list.size()-1; // The index of the last node
while (currentIndex > 0) {
int parentIndex - (currentIndex - 1) / 2;
// Swap if the current object is greater than its parent
if (list.get(current Index) .compareTo(
list.get(parent Index)) > 0) {
E temp = list.get(currentIndex):
list.set(current Index, list.get (parentIndex));
list.set(parentIndex, temp):
}
else
break; // The tree is a heap now
currentIndex - parentIndex;
/** Remove the root from the heap */
public E remove() {
if (list.size() == 0) return null;
E renovedObject - list.get(0);
list.set(0, list.get(list.size()-1));
list.remove(list.size()-1):
int current Index - 0:
while (currentIndex < list.size()) {](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F0b9c1552-116f-4e2a-bbd1-47a03e858b24%2Fb74c9063-4656-45d5-9055-9d67b1354a65%2Fn23pzor_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 6 images

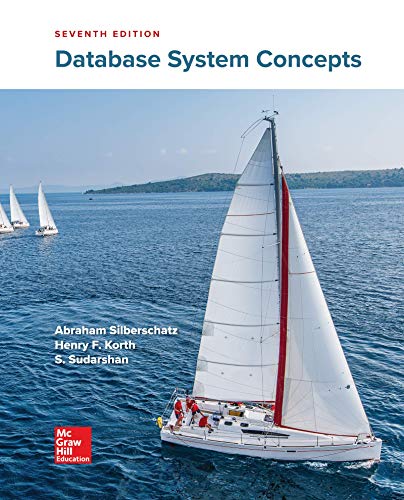
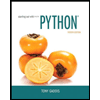
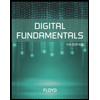
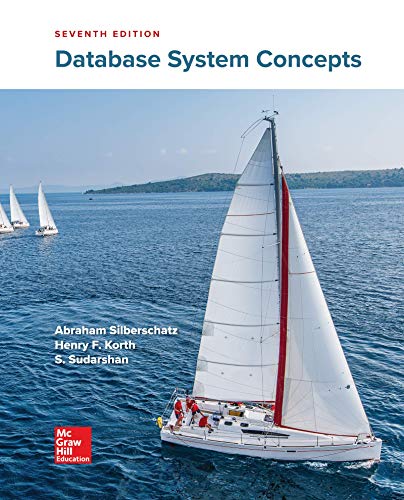
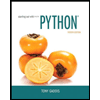
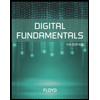
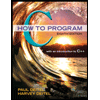
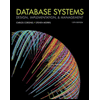
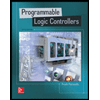