You are working as a software developer for a large insurance company. Your company is planning to migrate the existing systems from Visual Basic to Java and this will require new calculations. You will be creating a program that calculates the insurance payment category based on the BMI score.
Addition of Two Numbers
Adding two numbers in programming is essentially the same as adding two numbers in general arithmetic. A significant difference is that in programming, you need to pay attention to the data type of the variable that will hold the sum of two numbers.
C++
C++ is a general-purpose hybrid language, which supports both OOPs and procedural language designed and developed by Bjarne Stroustrup. It began in 1979 as “C with Classes” at Bell Labs and first appeared in the year 1985 as C++. It is the superset of C programming language, because it uses most of the C code syntax. Due to its hybrid functionality, it used to develop embedded systems, operating systems, web browser, GUI and video games.
I have this so far on the project, now I need to add exception handling...please help me complete my ongoing project...thanks!


Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

please fix code to match "enter patients name in lbs"
thank you
import java.util.LinkedList;
import java.util.Queue;
import java.util.Scanner;
interface Patient {
public String displayBMICategory(double bmi);
public String displayInsuranceCategory(double bmi);
}
public class BMI implements Patient {
public static void main(String[] args) {
/*
* local variable for saving the patient information
*/
double weight = 0;
String birthDate = "", name = "";
int height = 0;
Scanner scan = new Scanner(System.in);
String cont = "";
Queue<String> patients = new LinkedList<>();
// do while loop for keep running program till user not entered q
do {
System.out.print("Press Y for continue (Press q for exit!) ");
cont = scan.nextLine();
if (cont.equalsIgnoreCase("q")) {
System.out.println("Thank you for using BMI calculator");
break;
}
System.out.print("Please enter patient name: ");
/*
* try catch block for valid data for weight catch block for null value catch
* block for divide by zero exception if height is zero finally block
*/
try {
name = scan.nextLine();
System.out.print("Please enter the weight of the patient in kg: ");
weight = scan.nextDouble();
scan.nextLine();
System.out.print("Please entet the birth date of patient: ");
birthDate = scan.nextLine();
System.out.print("Please enter the height of patient in cm: ");
height = scan.nextInt();
scan.nextLine();
} catch (NumberFormatException e) {
System.out.println("Please enter valid data!");
} catch (ArithmeticException e) {
System.out.println("Height can not be zero!");
} catch (NullPointerException e) {
System.out.println("Name can not be blank!");
} finally {
System.out.println("Welcome to BMI calculator!!");
}
// calculate height in meter
double heightInMeter = (double) height / 100;
// calculate BMI
double bmi = weight / (heightInMeter * heightInMeter);
/*
* Print the patient name and date of birth print the patient BMI category print
* the patient insurance payment category
*/
Patient patient = new BMI();
patients.add(name);
System.out.println("Patient " + name + " and Date of birth is - " + birthDate
+ "\nPatient BMI category is - " + patient.displayBMICategory(bmi));
System.out.println("And Patient insurance payment category is - " + patient.displayInsuranceCategory(bmi));
} while (!cont.equalsIgnoreCase("q"));
System.out.println("WaitingQueue : " + patients);
String patientName = patients.remove();
System.out.println("Removed from WaitingQueue : " + patientName + " | New WaitingQueue : " + patients);
scan.close();
}
@Override
public String displayBMICategory(double bmi) {
String bmiCategory = "";
if (bmi <= 18.5) {
bmiCategory = "underweight";
} else if (bmi > 18.5 && bmi <= 24.9) {
bmiCategory = "Normal";
} else if (bmi > 25 && bmi <= 29.9) {
bmiCategory = "Overweight";
} else if (bmi > 29.9) {
bmiCategory = "Obesity";
}
return bmiCategory;
}
@Override
public String displayInsuranceCategory(double bmi) {
/*
* set Insurance Payment category as BMI category
*/
String insuranceCategory = "";
if (bmi <= 18.5) {
insuranceCategory = "Low";
} else if (bmi > 18.5 && bmi <= 24.9) {
insuranceCategory = "Low";
} else if (bmi > 25 && bmi <= 29.9) {
insuranceCategory = "High";
} else if (bmi > 29.9) {
insuranceCategory = "Highest";
}
return insuranceCategory;
}
}
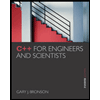
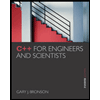