You are to write programming logic to convert temperature from Celsius to Fahrenheit for some specific values of temperatures. In performing the conversion for these values, you are to use a loop. Within the loop you will covert the specific values from Celsius to Fahrenheit. The loop may be either a while loop or a for loop. Write a program called temperatureLoop Convert.cpp to convert 3 temperature values from Celsius to Fahrenheit. Requirements. 1. Use these 3 Celsius temperature values: -40° C,0° C, 100° C in a loop. Each time the loop is performed (that is, each time the loop iterates) one of the Celsius temperature values is converted to Fahrenheit. That is: 1st time loop is performed/iterates: -40° C is converted to Fahrenheit. 2nd time loop is performed/iterates: 0° C is converted to Fahrenheit. 3rd time loop is performed/iterates: 100° C is converted to Fahrenheit. 2. From Programming Exercise 5.4: The loop must use this formula inside it. Each time the loop is performed (that is, each time the loop iterates) one of the Celsius temperature values is converted to Fahrenheit. That is: 1st time loop is performed/iterates the formula is used to convert -40° C to Fahrenheit. 2nd time loop is performed/iterates the formula is used to convert 0° C to Fahrenheit. 3rd time loop is performed/iterates the formula is used to convert 100° C to Fahrenheit. Note -40° C is -40° F, 0° C is 32° F, 100° C is 212° F. Feel free to use these values to make sure your program is displaying the correct Fahrenheit values Program I/O. Input: None. Output should be in a table like format that looks similar to this: Temperatures From Celsius To Fahrenheit -40 C 0 C 100 C F = (9/5) C + 32 -40 F 32 F 212 F ======
Types of Loop
Loops are the elements of programming in which a part of code is repeated a particular number of times. Loop executes the series of statements many times till the conditional statement becomes false.
Loops
Any task which is repeated more than one time is called a loop. Basically, loops can be divided into three types as while, do-while and for loop. There are so many programming languages like C, C++, JAVA, PYTHON, and many more where looping statements can be used for repetitive execution.
While Loop
Loop is a feature in the programming language. It helps us to execute a set of instructions regularly. The block of code executes until some conditions provided within that Loop are true.


Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

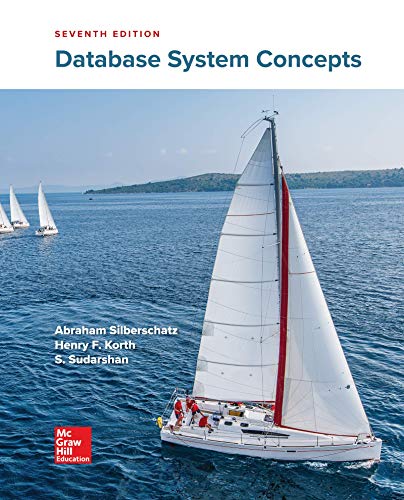
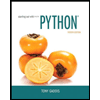
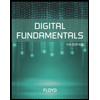
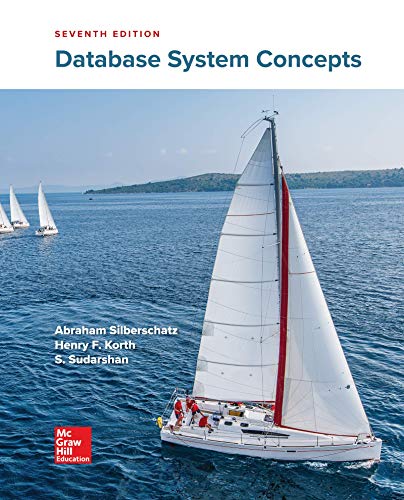
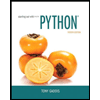
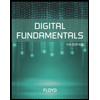
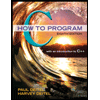
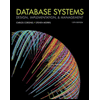
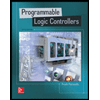