You are given a C++ program (Test2Q1.cpp) with 10 errors (syntax errors and/ or logical errors, if any). The program is developed to determine the optimization level of apartment design built-up area. It has five (5) user-defined functions as listed below: Study how all of the above functions were used/called inside the main function of the program. You are required to debug the errors, compile, and run the program. You are NOT ALLOWED to remove any statements in the program. You are only allowed to update the statements provided in the program and add a new statement(s) if absolutely necessary. Figure 1 is the source code of the program and Table 1 is the 3 (three) test cases that you can use to test the program to know if you have completely and correctly solved all the bugs. 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 #include #include #include // Max and min of built-up area (square feet) #define MAX_AREA 900 #define MIN_AREA 600 using namespace std; // function prototypes int getInput(string, int, int); void setBuiltArea(int, int); int setRoom(string); char getLevel(int); string getStatus(); // start main function int main() { // built area dimension / size (feet / square feet) int builtWidth, builtLength, builtArea; // total occupied area for rooms (square feet) int roomArea = 0; setBuiltArea(builtWidth, builtLength); builtArea = builtWidth * builtLength; cout << endl; // set and get the total room built size/area roomArea += setRoom("living"); roomArea += setRoom("kitchen"); roomArea += setRoom("bed"); roomArea = setRoom("bath"); // percentage of built-area occupied for room float percentOccupied = static_cast(roomArea) / builtArea * 100; cout << endl; 4 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 07 98 99 100 101 102 103 104 105 106 107 108 109 cout << "Built-up area is " << builtArea << " (square feet)" << endl; cout << "Total room area is " << roomArea << " (square feet)" << endl; cout << "Free space is "<< builtArea - roomArea << " (square feet)" << endl; cout << "Percentage of occupied area for rooms: " << percentOccupied << "%" << endl; cout << "Area optimization status is " << getStatus(percentOccupied) << endl; return 0; } // implement new user-defined functions // function to get integer number input (feet) for // built area and room size int getInput(string prompt, int min, int max) { int input; do { cout << prompt; cin << input; } while(input > min && input < max); } // Function to set built-up area. Then width must be in the // range of 14 to 20 feet while the length must be in the // range of 30 to 50 feet void setBuiltArea(int width, int length) { int area; // built-up area must be in the range of // 600 to 900 square feet while (area < MIN_AREA || area > MAX_AREA) { cout << "Set the built-up area (width and length in feet)\n"; width = getInput("Width: ", 14, 20); length = getInput("Length: ", 30, 50); area = width * length; if (area < MIN_AREA) cout << "Built-up area can't less than " << MIN_AREA << " square feet\n\n"; else if (area > MAX_AREA) cout << "Built-up area can't exceed " << MAX_AREA << " square feet\n\n"; } } // Function to set room size (living, kitchen, bed, & bath). // Room width and length must be in the range of 6 to 14 feet. int setRoom(string type) { int built_area; cout << "Set the " << type << " room area (width and length in feet)\n"; int width = getInput("Width: ", 6, 14); int length = getInput("Length: ", 6, 14); built_area = width * length; cout << endl; return built_area; } // Function to determine built-area optimization level. char getLevel(int percent) { if (percent >= 80) return 'A'; else if (percent >= 60) return 'B'; else return 'C'; 5 110 111 112 113 114 115 116 117 118 119 120 121 122 123 } // Function to determine built-area optimization status. string getStatus(int percent) { string status; swicth(getLevel(percent)) { case 'A': status = "excellent"; case 'B': status = "good"; break; case 'C': status = "bad"; } return 0; }
You are given a C++ program (Test2Q1.cpp) with 10 errors (syntax errors and/ or logical
errors, if any). The program is developed to determine the optimization level of apartment
design built-up area. It has five (5) user-defined functions as listed below:
Study how all of the above functions were used/called inside the main function of the program.
You are required to debug the errors, compile, and run the program. You are NOT ALLOWED
to remove any statements in the program. You are only allowed to update the statements
provided in the program and add a new statement(s) if absolutely necessary.
Figure 1 is the source code of the program and Table 1 is the 3 (three) test cases that you can
use to test the program to know if you have completely and correctly solved all the bugs.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 |
#include <iostream> #include <string> #include <cctype> // Max and min of built-up area (square feet) #define MAX_AREA 900 #define MIN_AREA 600 using namespace std; // function prototypes int getInput(string, int, int); void setBuiltArea(int, int); int setRoom(string); char getLevel(int); string getStatus(); // start main function int main() { // built area dimension / size (feet / square feet) int builtWidth, builtLength, builtArea; // total occupied area for rooms (square feet) int roomArea = 0; setBuiltArea(builtWidth, builtLength); builtArea = builtWidth * builtLength; cout << endl; // set and get the total room built size/area roomArea += setRoom("living"); roomArea += setRoom("kitchen"); roomArea += setRoom("bed"); roomArea = setRoom("bath"); // percentage of built-area occupied for room float percentOccupied = static_cast<float>(roomArea) / builtArea * 100; cout << endl; |
4
43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 07 98 99 100 101 102 103 104 105 106 107 108 109 |
cout << "Built-up area is " << builtArea << " (square feet)" << endl; cout << "Total room area is " << roomArea << " (square feet)" << endl; cout << "Free space is "<< builtArea - roomArea << " (square feet)" << endl; cout << "Percentage of occupied area for rooms: " << percentOccupied << "%" << endl; cout << "Area optimization status is " << getStatus(percentOccupied) << endl; return 0; } // implement new user-defined functions // function to get integer number input (feet) for // built area and room size int getInput(string prompt, int min, int max) { int input; do { cout << prompt; cin << input; } while(input > min && input < max); } // Function to set built-up area. Then width must be in the // range of 14 to 20 feet while the length must be in the // range of 30 to 50 feet void setBuiltArea(int width, int length) { int area; // built-up area must be in the range of // 600 to 900 square feet while (area < MIN_AREA || area > MAX_AREA) { cout << "Set the built-up area (width and length in feet)\n"; width = getInput("Width: ", 14, 20); length = getInput("Length: ", 30, 50); area = width * length; if (area < MIN_AREA) cout << "Built-up area can't less than " << MIN_AREA << " square feet\n\n"; else if (area > MAX_AREA) cout << "Built-up area can't exceed " << MAX_AREA << " square feet\n\n"; } } // Function to set room size (living, kitchen, bed, & bath). // Room width and length must be in the range of 6 to 14 feet. int setRoom(string type) { int built_area; cout << "Set the " << type << " room area (width and length in feet)\n"; int width = getInput("Width: ", 6, 14); int length = getInput("Length: ", 6, 14); built_area = width * length; cout << endl; return built_area; } // Function to determine built-area optimization level. char getLevel(int percent) { if (percent >= 80) return 'A'; else if (percent >= 60) return 'B'; else return 'C'; |
5
110 111 112 113 114 115 116 117 118 119 120 121 122 123 |
} // Function to determine built-area optimization status. string getStatus(int percent) { string status; swicth(getLevel(percent)) { case 'A': status = "excellent"; case 'B': status = "good"; break; case 'C': status = "bad"; } return 0; } |



Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 12 images

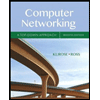
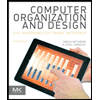
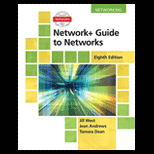
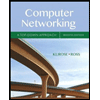
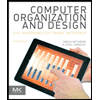
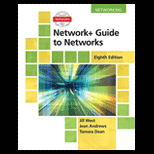
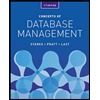
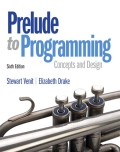
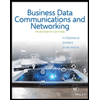